How to Trap Error in Visual Basic
Submitted by donbermoy on Sunday, February 16, 2014 - 19:39.
Error trapping let you intercept and deal with run-time errors, rather than having programs abort or ignore a fatal error and can possibly causing loss of data. But there is one way to trap error in Visual Basic. It has the same syntax with VB 6.0 and VB.NET.
To prevent the program from error we have to use the On Error Goto Statement.
Now lets start this tutorial! :)
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add a Label named lblAnswer, a Button named Button1 labeled as Compute, and lastly add two textboxes named TextBox1 and TextBox2. You must design your layout like this:
3. In your code module, code this for your Button1_Click:
Now, Try to input 7 in TextBox1 and 0 in TextBox2. This will trap the error.
Download the source code below and try it! :)
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
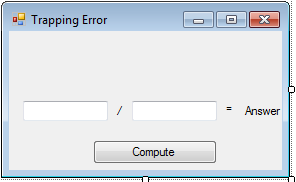
Note:
On Error GoTo here is our syntax for error trapping and the err is our variable if there is an error in our code. We initialized variable result as we divide the value of our TextBox1 and TextBox2. Take note that there is not a number that can be divided by zero so we put the Cannot be divided by 0. in our err variable if zero is inputed in TextBox2. Otherwise, the answer will display in lblAnswer. Try to input 7 in TextBox1 and 1 in TextBox2. The result will be like this: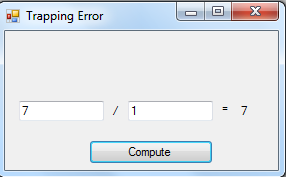
Output:
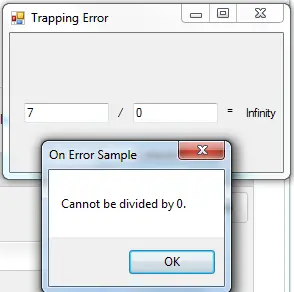
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz