How to Create Rock Paper Scissor Game in Python
In this tutorial, we will program 'How to Create Rock Paper Scissors Game in Python'. This time, we will learn to code a game called Rock, Paper, Scissors. The objective is to provide a proper insight for creating a simple game in Python programming. I will provide a sample program to demonstrate the actual coding of this tutorial.
This tutorial will demonstrate the proper way of creating a game using a specific library. The program I will show you is the creation of a simple game called Rock, Paper, Scissors. I will do my best to provide you with the best way to retrieve the CSV file. So, let's start with the coding.
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- import random
- import os
- import re
- def check_play_status():
- option = ['yes', 'no']
- while True:
- try:
- response = input('Do you wish to play again? (Yes or No): ')
- if response.lower() not in option:
- raise ValueError('Yes or No only')
- if response.lower() == 'yes':
- return True
- else:
- os.system('cls' if os.name == 'nt' else 'clear')
- print('Thanks for playing!')
- exit()
- except ValueError as err:
- print(err)
- def play_rps():
- play = True
- while play:
- os.system('cls' if os.name == 'nt' else 'clear')
- print('')
- print('================ RPS Game ================\n')
- user_choice = input('Choose your weapon'
- ' [R]ock], [P]aper, or [S]cissors: ')
- if not re.match("[SsRrPp]", user_choice):
- print('Please choose a letter:')
- print('[R]ock, [P]aper, or [S]cissors')
- continue
- print(f'You chose: {user_choice}')
- choices = ['R', 'P', 'S']
- opp_choice = random.choice(choices)
- print(f'I chose: {opp_choice}')
- if opp_choice == user_choice.upper():
- print('Tie!')
- play = check_play_status()
- elif opp_choice == 'R' and user_choice.upper() == 'S':
- print('Rock beats scissors, I win!')
- play = check_play_status()
- elif opp_choice == 'S' and user_choice.upper() == 'P':
- print('Scissors beats paper! I win!')
- play = check_play_status()
- elif opp_choice == 'P' and user_choice.upper() == 'R':
- print('Paper beats rock, I win!')
- play = check_play_status()
- else:
- print('You win!\n')
- play = check_play_status()
- if __name__ == '__main__':
- play_rps()
This Python script implements a Rock-Paper-Scissors game. It allows the user to play against the computer. The game continues until the user decides to stop playing.
After each round, the script asks the user if they want to play again. If the user inputs invalid options, the script prompts them to choose again. If the user chooses to stop playing, the script prints a thank you message and exits.
Output:
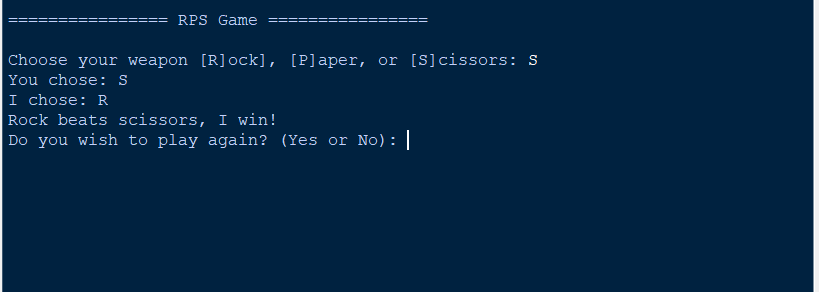
The How to Create Rock Paper Scissor Game in Python source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Create Rock Paper Scissor Game in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language