How to Create Input Field Dynamically in JavaScript
How to Create Input Field Dynamically in JavaScript
Introduction
In this tutorial we will create a How to Create Input Field Dynamically in JavaScript. This tutorial purpose is to teach how to create input field dynamically. This will cover all the basic function that will show the adding of new input field. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any form that needed more information.. I will give my best to provide you the easiest way of creating this program Dynamic input field. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will only display form inputs and the button. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-7">
- <form method="POST">
- <div id="forms" class="form-inline">
- <input type="text" class="form-control" style="width:80%;" placeholder="Please enter here" name="form[]">
- </div>
- <br />
- </form>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will dynamically add new input field when the button is clicked. To do this just copy and write these block of codes inside the text editor and save it as script.js.- var fieldId = 0;
- function addElement(parentId, elementTag, elementId, html){
- var id = document.getElementById(parentId);
- var newElement = document.createElement(elementTag);
- newElement.setAttribute('id', elementId);
- newElement.innerHTML = html;
- id.appendChild(newElement);
- }
- function removeField(elementId){
- var fieldId = "field-"+elementId;
- var element = document.getElementById(fieldId);
- element.parentNode.removeChild(element);
- }
- function addField(){
- fieldId++;
- var html = '<br /><input type="text" name="form[]" class="form-control" placeholder="Please enter here" style="width:80%;">' + '<button onclick="removeField('+fieldId+');"><span class="glyphicon glyphicon-minus"></span></button><br />';
- addElement('forms', 'div', 'field-'+ fieldId, html);
- }
In the first line of code we create a variable called fieldID, this act as the id of the input we create. We will then create a method called addElement(), this method will be the one who will create a new input field dynamically. This include all the creating of new element and append it into the targeted parent. Next we create the remove input method called removeField(), this function purpose is to delete the target field when you clicked the button.
Output:
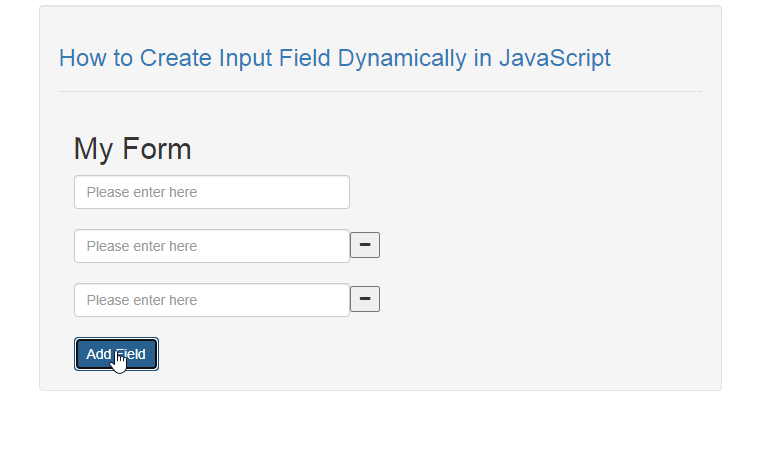
The How to Create Input Field Dynamically in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Create Input Field Dynamically in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language
Add new comment
- 4805 views