How to Redirect a Page with Timer in JavaScript
How to Redirect a Page with Timer in JavaScript
Introduction
In this tutorial we will create a How to Redirect a Page with Timer in JavaScript. This tutorial purpose is to make redirecting timer that will open to a targeted website. This will tackle the basic functionality for the redirecting. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any of your on working website that needed redirecting function. I will try my best to give you the easiest way of creating this program Redirecting Timer. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will display only the string that will display some specific information. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="widht=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;">
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will automatically start the timer and redirect you to another website when the timer expire. To do this just copy and write these block of codes inside the text editor and save it as script.js.- let time = 10;
- let counter;
- function countDownTimer() {
- let display = document.getElementById("timer");
- display.innerHTML = time;
- time--;
- if (time < 0) {
- clearInterval(counter);
- window.location.href = "https://sourcecodester.com";
- }
- }
- function startTimer() {
- counter = setInterval(countDownTimer, 1000);
- }
- startTimer();
In this code we first create a variable called time and set the value to 10. We also create an empty variable called counter that we will use to set a value. We will now create a method called countDownTimer(). In this method we will first bind the html text that will act as a timer naming display and set the value of the time on it.
To start our timer we will decrement first the time value, and set it to a condition when it reach zero it will call a specific function. The first we use is the clearInterval() this will destroy the timer when the timer expire and then we use window.location.href to redirect the page to a targeted website. Lastly we will create a method called startTimer() that will only set the interval of timer.
Output:
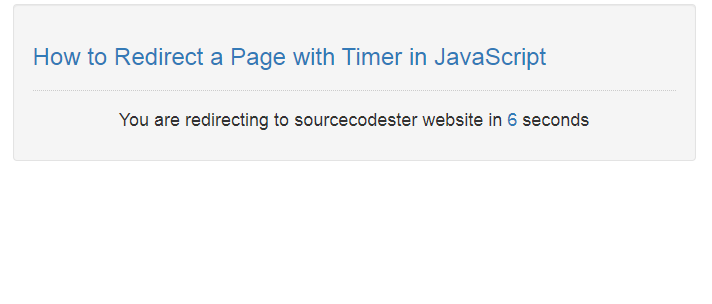
The How to Redirect a Page with Timer in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Redirect a Page with Timer in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language