How to Create Random Password in JavaScript
How to Create Random Password in JavaScript
Introduction
In this tutorial we will create a How to Create Random Password in JavaScript. This tutorial purpose is to teach how to generate your own unique password. This will tackle the randomization of characters. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is very easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use when you want create a random password. I will try my best to give you the easiest way of creating this program Random Password Generator. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will display an html form that we will be needed to to generate our password. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-5">
- <form class="form-group">
- <input type="text" id="password" placeholder="Password here..." class="form-control" readonly = "readonly"/>
- </form>
- <br />
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will generate a random password characters. To do this just copy and write these block of codes inside the text editor and save it as script.js.- var characters = "abcdefghijklmnopqrstubwsyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890";
- var password = '';
- function GeneratePassword(plength){
- password='';
- for(i=0; i<plength; i++){
- password+=characters.charAt(Math.floor(Math.random()*characters.length));
- }
- return password;
- }
- function displayPassword(){
- var plength = document.getElementById('plength').value;
- document.getElementById('password').value = GeneratePassword(plength);
- }
In this code we first create a variable that contains a different characters, this will be the base value of the password. After setting the variables we will now try to randomize the password, we use the empty variable called password to store the generated password.
To randomize a characters we use charAt() function to target characters and Math.floor() to formulate the entry where the value is the multiplied by Math.random() and password length
Output:
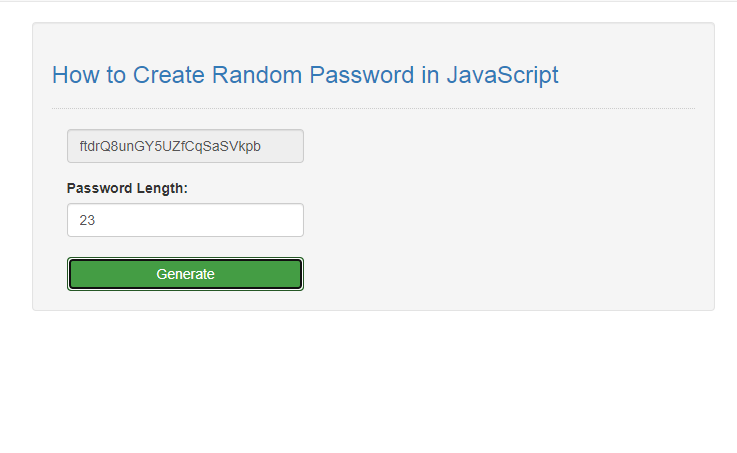
The How to Create Random Password in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Create Random Password in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language
Add new comment
- 265 views