Upload and Display Image using jQuery
Upload and Display Image using jQuery
Introduction
In this tutorial we will create a Upload and Display Image with jQuery. This tutorial purpose is to teach how to properly upload and display an image. This will cover all the important parts for creating an uploading form. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is easy to understand just follow my instruction I provided and you can do it without a problem. This program is useful if you want upload a profile image for your registered account. I will try my best to give you the easiest way of creating this program Upload and Display Image using jQuery. So let's do the coding.
Before we get started:
First you have to download the jQuery plugin.
Here is the link for the jQuery that i used in this tutorial https://jquery.com/.
Lastly, this is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will the uploading form for the image upload. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css">
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-1">
- </div>
- <div class="col-md-8">
- <form method="POST" enctype="multipart/form-data">
- <input type="file" id="photo" accept="image/*" />
- </form>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will upload the image file and then display it in the html page. To do this just copy and write these block of codes inside the text editor and save it as script.js.- $(document).ready(function(){
- $pic = $('<img id = "image" width = "100%" height = "100%"/>');
- $("#photo").change(function(){
- var files = !!this.files ? this.files : [];
- if(!files.length || !window.FileReader){
- $("#image").remove();
- $lbl.appendTo("#preview");
- }
- if(/^image/.test(files[0].type)){
- var reader = new FileReader();
- reader.readAsDataURL(files[0]);
- reader.onloadend = function(){
- $pic.appendTo("#preview");
- $("#image").attr("src", this.result);
- }
- }
- });
- });
In the given code we first call the basic jQuery ready event to signal that the DOM of the page is now ready to be use. After that we create new variable with a new element called $pic. We then get the input file attribute using id and append it in change() function. This function will trigger an event when a certain file is uploaded.
After we uploaded the file then check the image if it is actually a image being upload. We use FileReader() function to read the image file. Then use readAsDataURL() to get the image url. After that we use onloadend() to display the image in the targeted div.
Output:
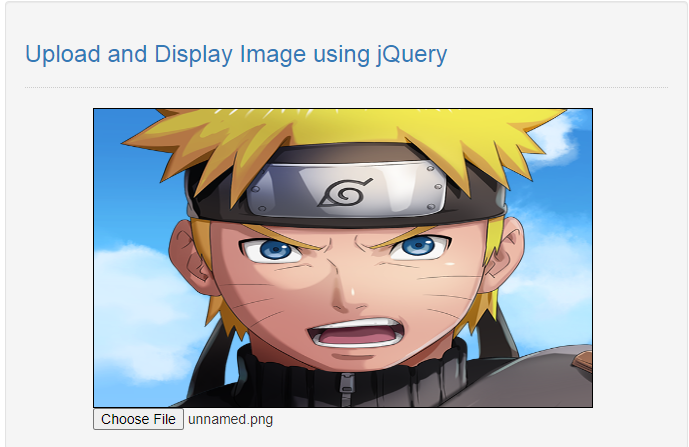
The Upload and Display Image using jQuery source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created Upload and Display Image using jQuery. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language
Add new comment
- 3122 views