How to Convert HTML Table to JSON Array with jQuery
How to Convert HTML Table to JSON Array with jQuery
Introduction
In this tutorial we will create a How to Convert HTML Table to JSON Array. This tutorial purpose is to teach how to convert html table into JSON data. This will cover all the important parts from creating a table and adding the converting script. I will provide a sample program to show the actual coding of this tutorial. So Let's do the coding.What is jQuery?
The purpose of jQuery is to make it much easier to use JavaScript on your working website projects. It will takes a lot of common tasks that require many lines of code to complete your function, and jQuery wraps them all into a methods that you can call with a single line of code. In short jQuery simplifies a lot of the complicated things from JavaScript, like AJAX calls and DOM manipulation.Before we get started:
First you have to download the jQuery plugin.
Here is the link for the jQuery that i used in this tutorial https://jquery.com/.
Lastly, this is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will display an interface that will show you the actual table with data included. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css" />
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="container-fluid">
- <div class="row">
- <div class="col-md-6 well">
- <div id="result">
- <div class="table-responsive">
- <table class="table table-bordered">
- <thead>
- <tr class="info">
- </tr>
- </thead>
- <tbody>
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- </tbody>
- </table>
- </div>
- </div>
- </div>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will compress and convert all the table data into JSON array object. To do this just copy and write these block of codes inside the text editor and save it as script.js.- $(document).ready(function(){
- $("#convert").click(function(){
- var myTable = { myTable: [] };
- var $th = $('table th');
- $('table tbody tr').each(function(i, tr){
- var obj = {}, $tds = $(tr).find('td');
- $th.each(function(index, th){
- obj[$(th).text()] = $tds.eq(index).text();
- });
- myTable.myTable.push(obj);
- });
- $("#result").html("<pre>"+JSON.stringify(myTable, null, 2));
- });
- $("#reset").click(function(){
- window.location = "index.html";
- });
- });
In the given code we first call the basic jQuery ready event to signal that the DOM of the page is now ready to be use. After we are all set up we then bind the button by targeting the ID and use the click function in order to trigger any button event.
To convert the table into json array object we must first create a global array variable to store the html data. Afterwards we then use the jQuery each function, this function will loop through the code base on the given condition. We now then use find function to track what data will be inserted and store it using push function into the objects
Output:
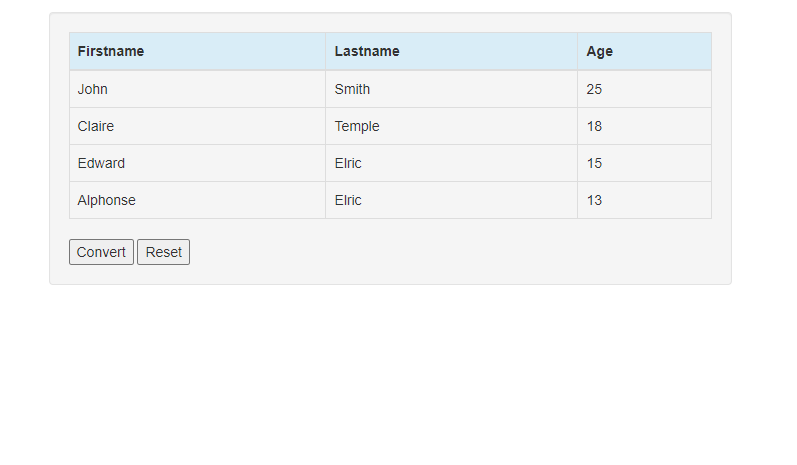
The How to Convert HTML Table to JSON Array with jQuery source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Convert HTML Table to JSON Array with jQuery. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language