How to Create a Pygame Menu Selection
In this tutorial we will create a Simple Main Menu Selection. Pygame is a Free and Open Source python programming language framework for making game applications. It is highly portable and runs on nearly every platform and operating system. It is highly recommended for beginners to learn Pygame when making basic games.
So let's now do the coding...
Getting Started
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
After Python IDLE's is installed, open the command prompt then type "python -m pip install pygame", and hit enter.
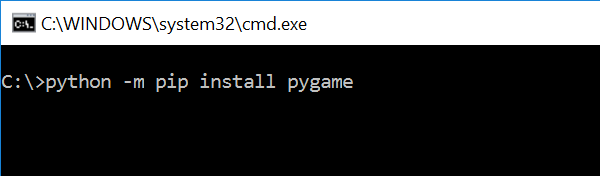
Importing Modules
After setting up the installation, run the IDLE and click the file and then the new file. After that, a new window will appear containing a black file this will be the text editor for the python.
Then copy the code that I provided below and paste it inside the IDLE text editor
- import pygame
- from pygame.locals import *
- import os
Writing The Variables
We will assign the certain variables that we will need to declare later in the main loop. To do that just kindly write this code inside your text editor.
- # Game Initialization
- pygame.init()
- # Center the Game Application
- os.environ['SDL_VIDEO_CENTERED'] = '1'
- # Game Resolution
- screen_width=800
- screen_height=600
- screen=pygame.display.set_mode((screen_width, screen_height))
- # Text Renderer
- def text_format(message, textFont, textSize, textColor):
- newFont=pygame.font.Font(textFont, textSize)
- newText=newFont.render(message, 0, textColor)
- return newText
- # Colors
- white=(255, 255, 255)
- black=(0, 0, 0)
- gray=(50, 50, 50)
- red=(255, 0, 0)
- green=(0, 255, 0)
- blue=(0, 0, 255)
- yellow=(255, 255, 0)
- # Game Fonts
- font = "Retro.ttf"
- # Game Framerate
- clock = pygame.time.Clock()
- FPS=30
Main Function
This is the Main Code for the Pygame application. The code contains several input functions that will allow the object to move and render it to the application. To do that just write this code inside the IDLE text editor.
- # Main Menu
- def main_menu():
- menu=True
- selected="start"
- while menu:
- for event in pygame.event.get():
- if event.type==pygame.QUIT:
- pygame.quit()
- quit()
- if event.type==pygame.KEYDOWN:
- if event.key==pygame.K_UP:
- selected="start"
- elif event.key==pygame.K_DOWN:
- selected="quit"
- if event.key==pygame.K_RETURN:
- if selected=="start":
- print("Start")
- if selected=="quit":
- pygame.quit()
- quit()
- # Main Menu UI
- screen.fill(blue)
- title=text_format("Sourcecodester", font, 90, yellow)
- if selected=="start":
- text_start=text_format("START", font, 75, white)
- else:
- text_start = text_format("START", font, 75, black)
- if selected=="quit":
- text_quit=text_format("QUIT", font, 75, white)
- else:
- text_quit = text_format("QUIT", font, 75, black)
- title_rect=title.get_rect()
- start_rect=text_start.get_rect()
- quit_rect=text_quit.get_rect()
- # Main Menu Text
- screen.blit(title, (screen_width/2 - (title_rect[2]/2), 80))
- screen.blit(text_start, (screen_width/2 - (start_rect[2]/2), 300))
- screen.blit(text_quit, (screen_width/2 - (quit_rect[2]/2), 360))
- pygame.display.update()
- clock.tick(FPS)
- pygame.display.set_caption("Python - Pygame Simple Main Menu Selection")
Initializing The Game
This is the Initialization code, this will run the entire code when the application starts. It will render the Main Loop to display the specific methods. To do that just write this code inside the IDLE text editor.
- #Initialize the Game
- main_menu()
- pygame.quit()
- quit()
DEMO
There you have it we successfully created a Simple Main Menu Selection using Pygame. I hope that this simple tutorial helps you to what you are looking for and enhance your programming capabilities. For more updates and tutorials just kindly visit this site.
Enjoy Coding!!
Comments
Error when running the code.
Add new comment
- Add new comment
- 15283 views