Encrypt and Decrypt a Text using VB6
Submitted by donbermoy on Friday, March 21, 2014 - 06:10.
Last week, we made a program in vb.net that has encryption only in a text. Now in this article, we will create a program in VB6.0 that will encrypt and decrypt a text.
Now, let's start this tutorial!
1.Let's start this tutorial by following the following steps in Microsoft Visual Basic 6.0: Open Microsoft Visual Basic 6.0, click Choose Standard EXE, and click Open.
2.Next, add two Buttons named Command1 and labeled it as "Encrypt". Add also DTPicker named DTPicker1 and format it dtpShortDate .You must design your interface like this:
3. Now put this code for your code module.
Download the source code and try it! :)
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below and hire me.
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09488225971
Telephone:826-9296
E-mail:[email protected]
Follow and add me in my Facebook Account: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
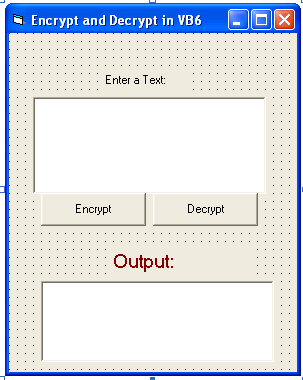
- Private Sub Command1_Click()
- Text2.Text = Encrypt(Text1.Text, Len(Text1.Text))
- End Sub
- Public Function Encrypt(Name As String, Key As Long) As String
- Dim v As Long, c1 As String, z As String
- For v = 1 To Len(Name)
- c1 = Asc(Mid(Name, v, 1))
- c1 = Chr(c1 + Key)
- z = z & c1
- Next v
- Encrypt = z
- End Function
- Private Sub Command2_Click()
- Text2.Text = Decrypt(Text2.Text, Len(Text1.Text))
- Text1.Text = ""
- End Sub
- Public Function Decrypt(Name As String, Key As Long) As String
- Dim v As Long, c1 As String, z As String
- For v = 1 To Len(Name)
- c1 = Asc(Mid(Name, v, 1))
- c1 = Chr(c1 - Key)
- z = z & c1
- Next v
- Decrypt = z
- End Function
Explanation:
We have created a function for Encrypt and Decrypt that has the parameters of Name As String and Key As Long. We have initialized v as Long Integer, variable c1 as String, and variable z as String. Then we have created a For Next Loop where v=1 up to the Length of text to our Name variable. Variable c1 now will be equal to Asc Function of Mid text of the name, starts at variable v, and the length of 1. Asc Function returns an Integer representing the character code corresponding to the first letter in a string. The required string argument is any valid string expression. If the string contains no characters, a run-time error occurs. Then c1 = Chr(c1 + Key). This statement is the main syntax for encrypting and decrypting the text. If we put Chr(c1 + Key) it is used for encryption and Chr(c1 - Key) is used for decryption. Chr Function returns a String containing the character associated with the specified character code. The required charcode argument is a Long that identifies a character. Command1/Button1 was used for encryption as Text2 will have the value of the encrypt word inputted in Text1 and its length. And Command2/Button2 was used for decryption as Text2 will be decrypted the encrypted text in Text2.Output:
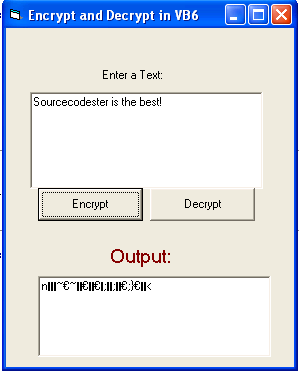
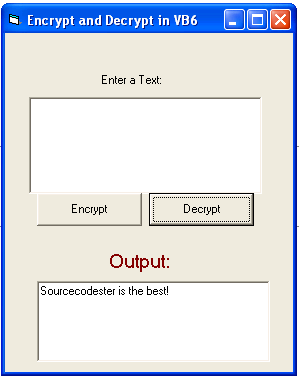
Comments
Disclaimer
This post should have a giant disclaimer that this encryption algorithm should in no possible way be considered secure. It should only be used to obscure a text for personal fun at the most, never for any data that you want to secure. Even if you don't care about the data, in this day and age you should jut download a control that does real encryption.