Typeerror: not enough arguments for format string [Solved]
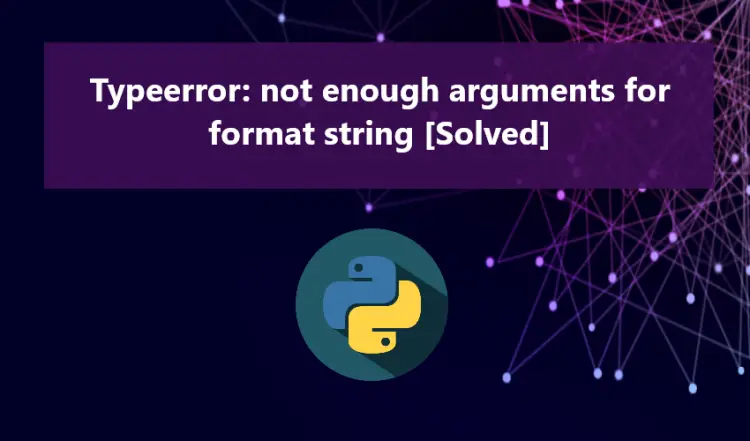
In this article, we will delve into the reasons behind the Python TypeError exception, specifically when it reads TypeError: not enough arguments for format string, and we will explore solutions to resolve this error. If you are currently grappling with this error during the development of your Python project, this article aims to provide you with insights and knowledge on why this error occurs and how to rectify it.
The TypeError: not enough arguments for format string is one of the error exceptions thrown by Python, categorized under TypeError. TypeError is a type of error in Python that pertains to data or object types. This error arises when attempting an operation that requires a specific type of object or data but a different type is provided. It is often accompanied by exception messages such as the following:
- "TypeError: 'str' object cannot be interpreted as an integer"
- "TypeError: 'int' object is not callable"
- "Typeerror: can't multiply sequence by non-int of type 'numpy.float64'"
What Causes the Python "TypeError: not enough arguments for format string" Error?
The "TypeError: not enough arguments for format string" error occurs when you use string formatting, either with the format() method or f-strings string formatting syntax, and the number of provided placeholders, such as `%s` or `{}`, does not match the number of values provided. This error may also occur due to an incorrect syntax when formatting the string.
In essence, this error occurs when attempting to format a string like the following:
- # Sample List of Employees
- employees = ["Mark Cooper", "Samantha Lou", "George Wilson", "John Smith"]
- # Message format
- msg = "Pay slips for %s, %s, %s, %s, and %s have been generated successfully."
- # Formatted Message
- formattedMsg = msg % (employees[0], employees[1], employees[2], employees[3])
- #output
- print(formattedMsg)
The error can be easily fixed by ensuring that the count of placeholders matches the number of values provided when formatting the string.
Moreover, the mentioned error will also be triggered when the syntax for formatting the string is incorrect. Here's an example Python snippet that triggers the TypeError: not enough arguments for format string error:
- # Sample List of Employees
- employees = ["Mark Cooper", "Samantha Lou", "George Wilson", "John Smith"]
- # Message format
- msg = "%s, %s, %s, and %s payslip's has been generated successfully"
- # Formatted Message
- formattedMsg = msg % employees[0], employees[1], employees[2], employees[3]
- #output
- print(formattedMsg)
Despite the placeholder count matching the number of values provided in the scenario above, running the script will still throw the mentioned exception and lead to the entire script's execution crashing. This error occurs because of the incorrect syntax used for formatting the string.
Solution #1
The simple solution for the provided snippet is to enclose the values within parentheses (). By doing so, the syntax for formatting the string will be corrected, and the script will execute properly.
- # Sample List of Employees
- employees = ["Mark Cooper", "Samantha Lou", "George Wilson", "John Smith"]
- # Message format
- msg = "Pay slips for %s, %s, %s, and %s have been generated successfully."
- # Formatted Message
- formattedMsg = msg % (employees[0], employees[1], employees[2], employees[3])
- #output
- print(formattedMsg)
Solution #2
We can also utilize the format() method to correctly format the string. The format() method is a Python built-in function designed for formatting strings with placeholders and actual values.
Here's the following snippet that demonstrate this solution:
- # Sample List of Employees
- employees = ["Mark Cooper", "Samantha Lou", "George Wilson", "John Smith"]
- # Message format
- msg = "Pay slips for {}, {}, {}, and {} have been generated successfully."
- # Formatted Message
- formattedMsg = msg.format(employees[0], employees[1], employees[2], employees[3])
- #output
- print(formattedMsg)
Solution #3
Furthermore, we can also iterate through the values of the string format from a list object using the asterisk ( * ). Refer to the following snippet for a better understanding of this:
- # Sample List of Employees
- employees = ["Mark Cooper", "Samantha Lou", "George Wilson", "John Smith"]
- # Message format
- msg = "Pay slips for {}, {}, {}, and {} have been generated successfully."
- # Formatted Message
- formattedMsg = msg.format(*employees)
- #output
- print(formattedMsg)
Conclusion
In simple terms, the "TypeError: not enough arguments for format string" error occurs when attempting to format strings with an unequal number of placeholders and provided values, or due to incorrect syntax for formatting the string. By following the solutions provided above, you can resolve this error.
And there you have it! I hope this article helps you address the error you are currently facing. Explore more on this website for additional Free Source Codes, Tutorials, and Articles covering various programming languages.