How to Filter Data in the DataGridview Using CheckBoxes in VB.Net
Submitted by janobe on Sunday, September 22, 2019 - 10:02.
In this tutorial, I will teach you how to filter the data in the datagridview using checkboxes in vb.net. This method has the ability to retrieve the data in the database and It will be displayed in the datagridview depending on the checked in a group of checkboxes. This procedure is very helpful if you are currently working on the inventory of products.
The complete source code is included you can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating a Database
Go to http://localhost/phpmyadmin/ and create a new database named "tuts_persondb". after that execute the query for creating a table and adding the data in the table.- --
- -- Dumping data for table `tblperson`
- --
- (1, 'Janobe Sourcecode', 'Single'),
- (2, 'Mark Lim', 'Married'),
- (3, 'Jake Cueca', 'Widow');
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for visual basic.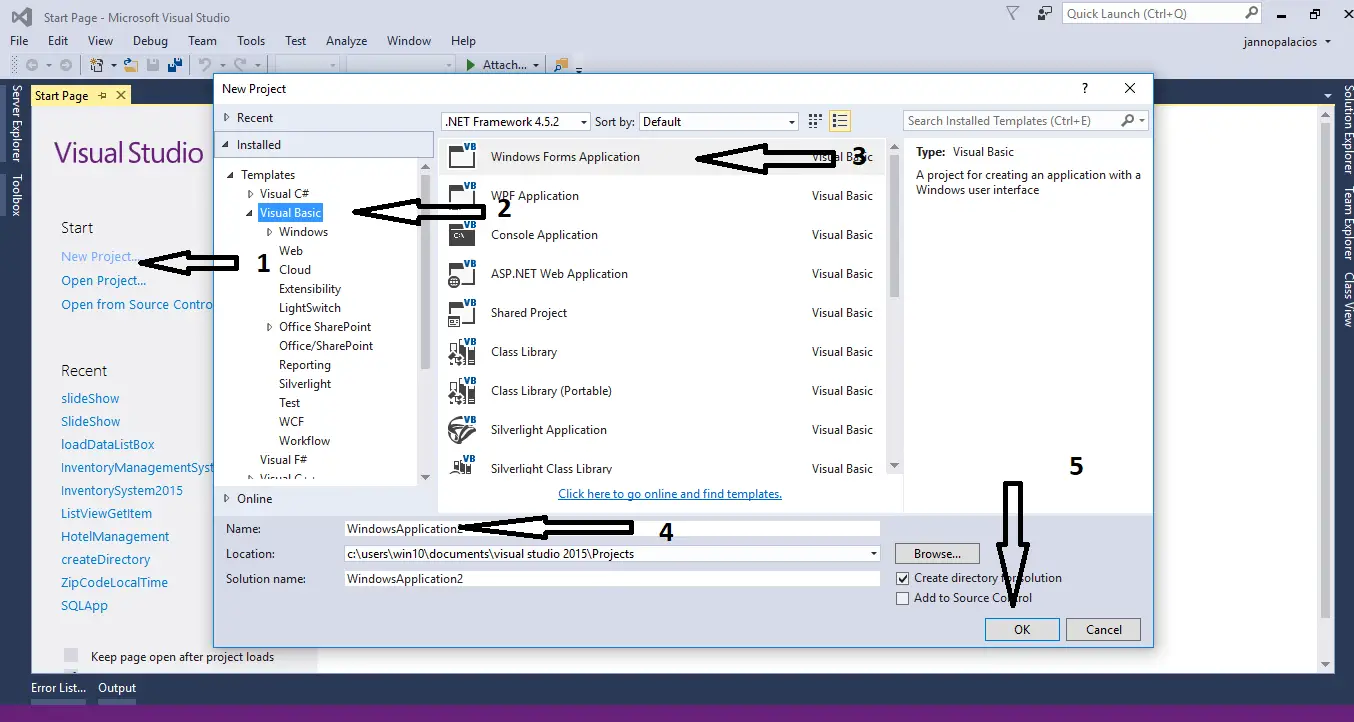
Step 2
Do the form just like shown below.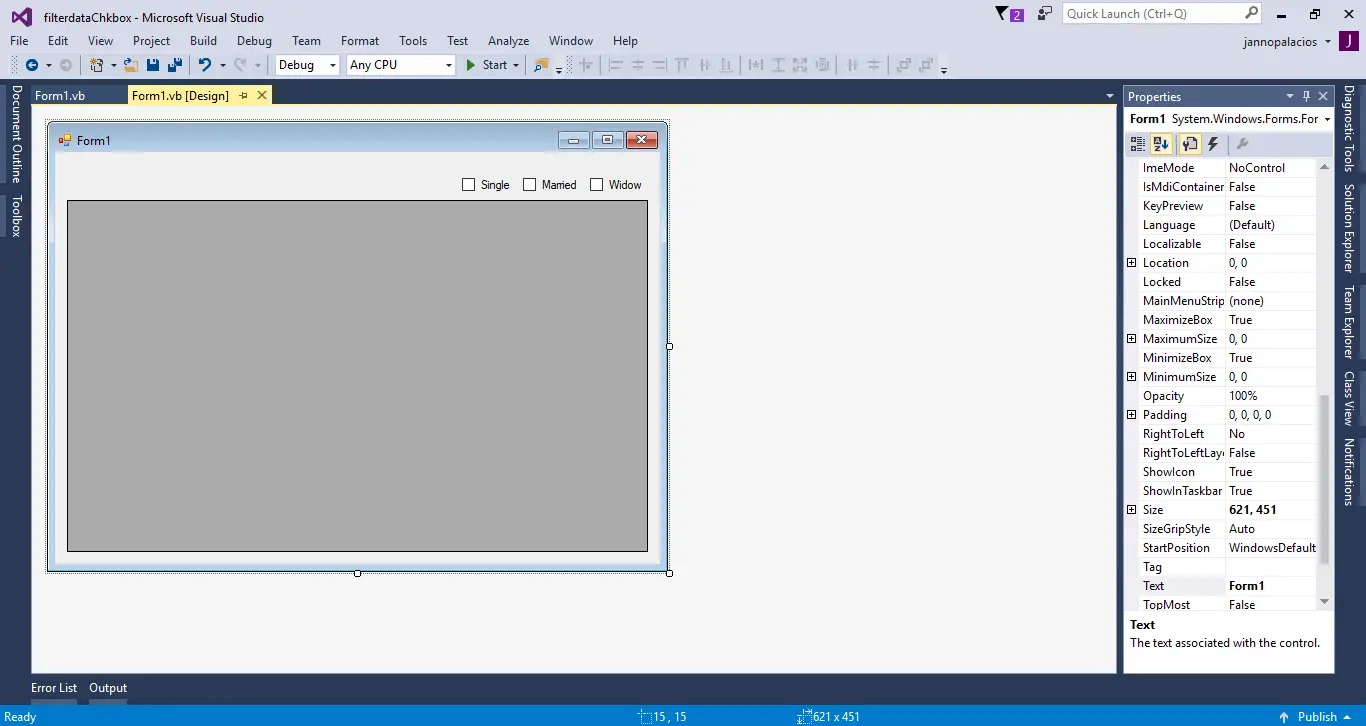
Step 3
Add MySQL.Data.dllStep 4
Press F7 to open the code editor. In the code editor, add a namespace to accessMySQL
libraries
- using MySql.Data.MySqlClient;
Step 5
Establish a connection between visual basic and MySQL database. After that, declare all the classes and variables that are needed.- Dim con As MySqlConnection = New MySqlConnection("server=localhost;user id =root;password=;database=tuts_persondb;sslMode=none")
- Dim cmd As MySqlCommand
- Dim da As MySqlDataAdapter
- Dim dt As DataTable
- Dim sql As String = ""
- Dim stat_single As String = ""
- Dim stat_married As String = ""
- Dim stat_widow As String = ""
Step 6
Create a method for retrieving data in the database.- Private Sub loadData(sql As String)
- Try
- con.Open()
- cmd = New MySqlCommand
- da = New MySqlDataAdapter
- dt = New DataTable
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- da.SelectCommand = cmd
- da.Fill(dt)
- DataGridView1.DataSource = dt
- Catch ex As Exception
- MsgBox(ex.Message)
- Finally
- con.Close()
- da.Dispose()
- End Try
- End Sub
Step 7
Write the following code to display the data into the DataGridView in the first load of the form.- Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
- sql = "SELECT * FROM `tblperson`"
- loadData(sql)
- End Sub
Step 7
Write the following code for filtering the data in the DataGridView using the checkboxes when it's checked.- Private Sub chkSingle_CheckedChanged(sender As Object, e As EventArgs) Handles chkSingle.CheckedChanged, chkMarried.CheckedChanged, chkWidow.CheckedChanged
- If chkSingle.Checked Then
- stat_single = chkSingle.Text
- End If
- If chkMarried.Checked Then
- stat_married = chkMarried.Text
- End If
- If chkWidow.Checked Then
- stat_widow = chkWidow.Text
- End If
- sql = "SELECT * FROM `tblperson` WHERE `CivilStatus` in ('" & stat_single & "','" & stat_married & "','" & stat_widow & "')"
- loadData(sql)
- End Sub