How to Clear ComboBox with DataSource in VB.Net and MySQL Database
Submitted by janobe on Monday, September 10, 2018 - 16:58.
This time, I will teach you how to clear ComboBox with DataSource in VB.Net and MySQL database. Removing list of records in the combo box that has a data source is a bit hard to do especially for beginners in programming. So I made this tutorial to help you clear all the data that’s inside the combo box in just a click. Below are the procedures on how to make it work.
Note: add
Creating the Database
Create a database and named it "test" Then, create a table in the database that you have created.Creating an Application
Step 1
Open Microsoft Visual Studio 2015 and create a new window form application. Then do the form just like this.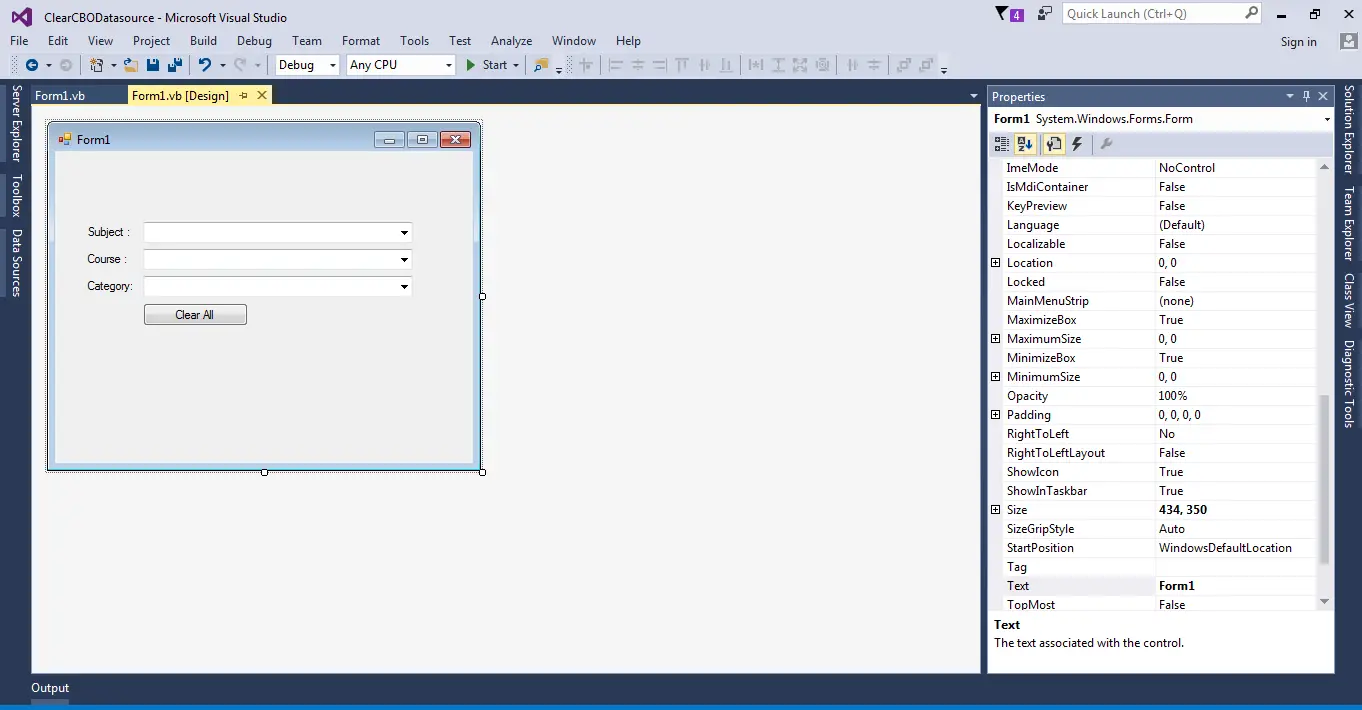
Step 2
Double click the form to fire the code editor.Step 3
In the code editor, set the import above the Public Class.- Imports MySql.Data.MySqlClient
Step 4
Initialize all the classes that are needed inside the Public Class.- Dim con As MySqlConnection = New MySqlConnection("server=localhost;user id=root;password=;database=test;sslMode=none")
- Dim cmd As MySqlCommand
- Dim da As MySqlDataAdapter
- Dim dt As DataTable
- Dim sql As String
Step 5
Create a sub procedure for retrieving the data in the combobox.- Private Sub fillComboBox(sql As String, feilds As String, combo As ComboBox)
- Try
- con.Open()
- cmd = New MySqlCommand
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- da = New MySqlDataAdapter
- da.SelectCommand = cmd
- dt = New DataTable
- da.Fill(dt)
- With combo
- .DataSource = dt
- .DisplayMember = feilds
- End With
- Catch ex As Exception
- MsgBox(ex.Message)
- Finally
- con.Close()
- da.Dispose()
- End Try
- End Sub
Step 6
Do this following code for retrieving data on the first load of the form.- Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
- fillComboBox("select * from tblrecords", "Subject", cboSubject)
- fillComboBox("select * from tblrecords", "Course", cboCourse)
- fillComboBox("select * from tblrecords", "Category", cboCategory)
- End Sub
Step 7
Go to the design views and double click the “Clear All” button to fire theclick event handler
of it. After that, create a code for clearing the entire combo box with data source.
- Try
- For Each combo In Me.Controls
- If TypeOf combo Is ComboBox Then
- combo.DataSource = Nothing
- End If
- Next combo
- Catch ex As Exception
- MsgBox(ex.Message)
- End Try
mysql.data.dll
for the references to access MySQL Library
The complete sourcecode is included. You can download it and run it on your computer.
For more question about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
FB Account – https://www.facebook.com/onnaj.soicalap