PHP - Simple Change Image Name When Upload
Submitted by razormist on Sunday, June 16, 2019 - 14:18.
In this tutorial we will create a Simple Change Image Name When Upload using PHP. This code can rename the upload images when the user enter a value in the textbox and upload the file to the database server. The code use explode() a php built-in function that disassemble any string base on the given parameter in order to concatenate the value with the POST data. This a user-friendly program feel free to modify and use it to your system.
We will be using PHP as a scripting language that interpret in the webserver such as xamp, wamp, etc. It is widely use by modern website application to handle and protect user confidential information.
There you have it we successfully created Simple Change Image Name When Upload using PHP. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating Database
Open your database web server then create a database name in it db_rename, after that click Import then locate the database file inside the folder of the application then click ok.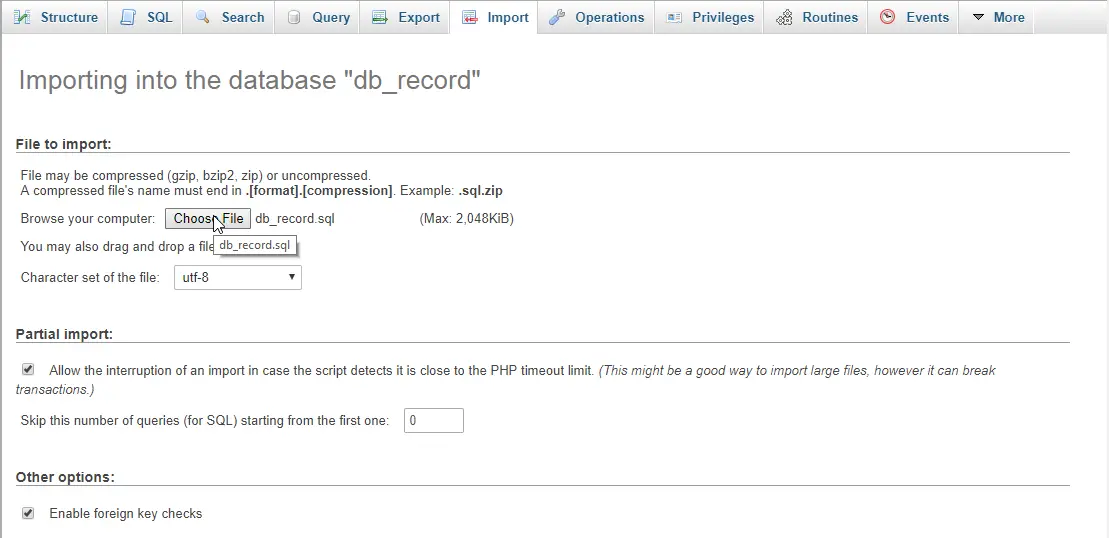
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- $conn = new mysqli('localhost', 'root', '', 'db_rename');
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css" />
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Change Image Name When Upload</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-3">
- <form method="POST" enctype="multipart/form-data">
- <h4>Upload here</h4>
- <input type="file" name="file" required="required"/>
- <br />
- <div class="form-group">
- <input type="text" class="form-control" name="filename" placeholder="Enter file name" required="required"/>
- </div>
- <center><button class="btn btn-primary" name="upload"><span class="glyphicon glyphicon-upload"></span> Upload</button></center>
- </form>
- </div>
- <div class="col-md-3"></div>
- <div class="col-md-6">
- <?php include'rename.php'?>
- </div>
- </div>
- </body>
- </html>
Creating Main Function
This code contains the main function of the application. This code rename the uploaded image when the button is clicked. To do that just copy and write this block of codes inside the text editor, then save it as rename.php.- <?php
- require_once 'conn.php';
- $file_name=$_POST['filename'];
- if($_FILES['file']['size'] < 1048576){
- $name = $file_name.".".$end;
- $path = "upload/".$name;
- $conn->query("INSERT INTO `image` VALUES('', '$name', '$path')");
- }
- echo "<center><img src='$path' width='200' height='200'/></center>";
- echo"<center><h3>".$name."</h3></center>";
- }else{
- echo "File too large to upload";
- }
- }else{
- echo "Invalid file format";
- }
- }
- ?>