PHP - Simple Insert Multiple Entries
Submitted by razormist on Wednesday, May 1, 2019 - 20:56.
In this tutorial we will create a Simple Insert Multiple Entries using PHP. This code will allow the user to insert multiples form data in just one click. The code use javascript onclick() function to create a new element in the form, then when submit the php script will break down the form array by using foreach in order to insert the in the MySQLi database one by one. This a user-friendly program feel free to modify and use it to your system.
We will be using PHP as a scripting language that interepret in the webserver such as xamp, wamp, etc. It is widely use by modern website application to handle and protect user confidential information.
script.js
Note: To make this code work make sure you save this file inside the js directory.
There you have it we successfully created Simple Insert Multiple Entries using PHP. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating Database
Open your database web server then create a database name in it db_entry, after that click Import then locate the database file inside the folder of the application then click ok.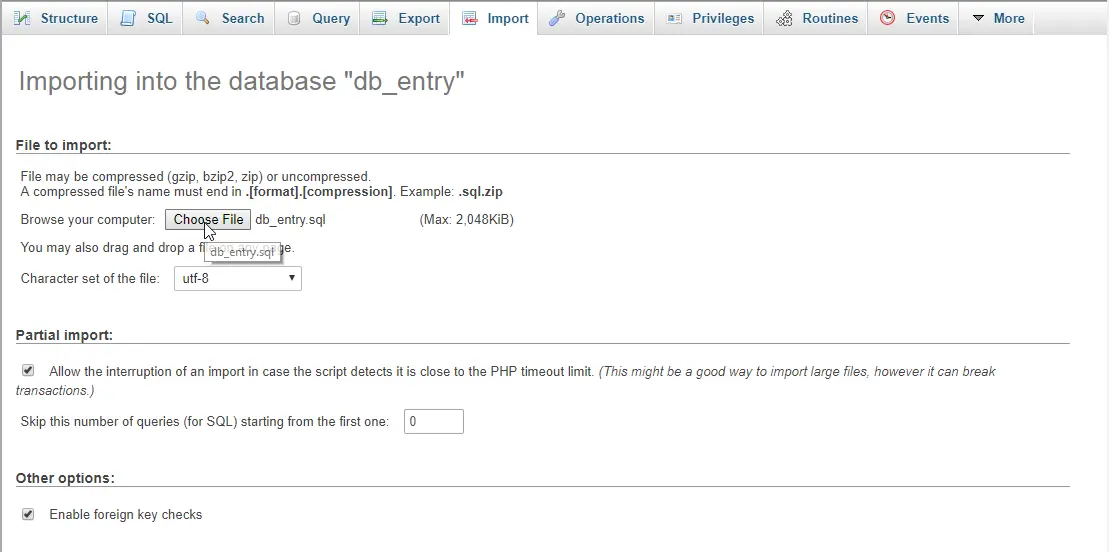
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="comtainer-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Insert Multiple Entries</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <button class="btn btn-success" onclick="addEntry();"><span class="glyphicon glyphicon-plus"></span> Add entry</button>
- <br />
- <br />
- <br />
- <div class="col-md-5">
- <form method="POST" action="insert.php">
- <div id="form-entry">
- <div class="form-group">
- <input type="text" name="entry[]" placeholder="Enter here..." class="form-control" required="required"/>
- </div>
- </div>
- <button class="btn btn-primary" name="insert"><span class="glyphicon glyphicon-save"></span> Insert</button>
- </form>
- </div>
- <div class="col-md-2"></div>
- <div class="col-md-4">
- <table class="table table-bordered">
- <thead>
- <tr>
- <th>Name</th>
- </tr>
- </thead>
- <tbody>
- <?php
- require'conn.php';
- ?>
- <tr>
- <td><?php echo $fetch['name']?></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- </div>
- </div>
- <script src="js/script.js"></script>
- </body>
- </html>
Creating the Main Function
This code contains the main function of the application. This code will insert multiple data when the button is clicked. To make this just copy and write these block of codes below inside the text editor, then save it as shown below. insert.php- <?php
- require_once 'conn.php';
- $entry = $_POST['entry'];
- foreach($entry as $value){
- }
- }
- ?>
- function addEntry(){
- var entry="<input type='text' name='entry[]' placeholder='Enter here...' class='form-control' required='required'/>";
- var element=document.createElement("div");
- element.setAttribute('class', 'form-group');
- element.innerHTML=entry;
- document.getElementById('form-entry').appendChild(element);
- }
Add new comment
- 557 views