PHP - Simple Email Verification Using PHPMailer
Submitted by razormist on Wednesday, September 12, 2018 - 15:38.
In this tutorial we will create a Simple Email Verification Using PHPMailer. PHP is a server-side scripting language designed primarily for web development. PHP is a server-side scripting language designed primarily for web development. It is mostly used by a newly coders for its user friendly environment. So let's now do the coding...
Then, open your command prompt and cd to your working directory. After that type this command and hit enter afterwards.
After installation you will see different directory inside on your working directory, namely vendor, etc.
Without Composer
To manually install the phpmailer to your app, first download the file here https://github.com/PHPMailer/PHPMailer
After you finish downloading the file, move the file inside the directory that you are working on. Now you're ready to use the phpmailer.
register_form.php
home.php
verification.php
verified.php
Into this:
So that it will recognize the phpmailer class when you call it in the code.
Note: To be able to send a mail to gmail, make sure you provide your own gmail username and password or much better is to make a dummy account to use.
Inside your google account make sure you allow the less secure apps so that you can have permission to send the email.
There you have it we successfully created a Simple Email Verification Using PHPMailer. I hope that these simple tutorial help you to your projects. For more updates and tutorials just kindly visit this site. Enjoy Coding!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And this is the link for the jquery that i used in this tutorial https://jquery.com/. Lastly, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Installing the PHPMailer
Using Composer If you dont' have a composer installed on your machine you can get it here, https://getcomposer.org/download/ First, Create a file that will receive the phpmailer file, write these code inside the text editor and save it as composer.json.- <?php
- {
- "phpmailer/phpmailer": "~6.0"
- }
- ?>
- composer require phpmailer/phpmailer
Creating Database
Open your database web server then create a database name in it db_verify, after that click Import then locate the database file inside the folder of the application then click ok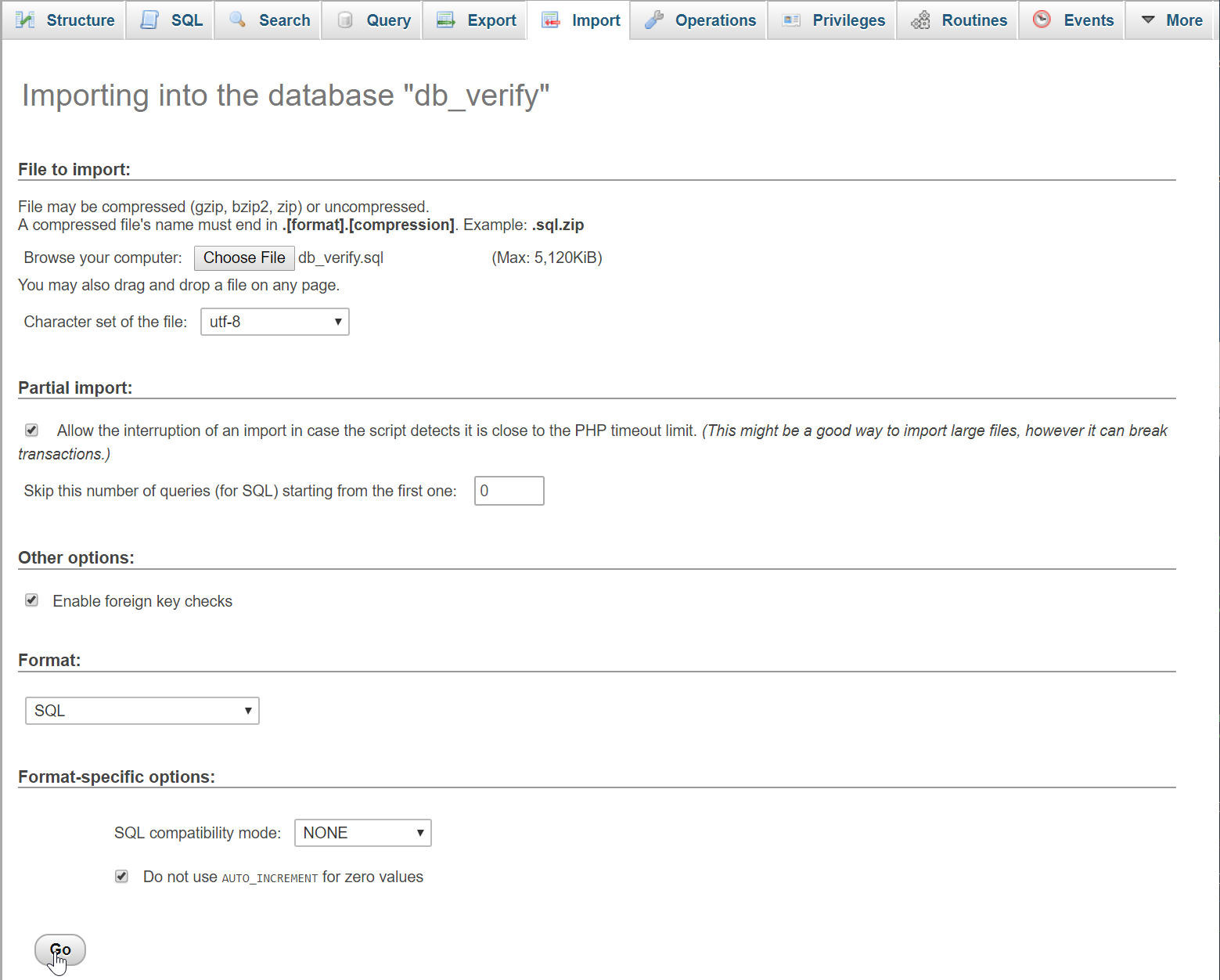
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as shown below. index.php- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Email Verification Using PHPMailer</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-3"></div>
- <div class="col-md-6">
- <a href="register_form.php">Not a member? Sign up here...</a>
- <br /><br />
- <form method="POST" action="login.php">
- <div class="form-group">
- <label>Username</label>
- <input type="text" name="username" class="form-control" required="required"/>
- </div>
- <div class="form-group">
- <label>Password</label>
- <input type="password" name="password" maxlength="12" class="form-control" required="required"/>
- </div>
- <div class="form-group">
- <button name="login" class="btn btn-primary btn-block"><span class="glyphicon glyphicon-log-in"></span> Login</button>
- </div>
- <?php
- ?>
- <div class="alert alert-danger"><?php echo $_SESSION['message']?></div>
- <?php
- }
- ?>
- </form>
- </div>
- </div>
- </body>
- </html>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Email Verification Using PHPMailer</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-6">
- <a href="index.php">Already a member? Sign in here...</a>
- <br /><br />
- <form method="POST" action="register.php">
- <div class="form-group">
- <label>Username</label>
- <input type="text" name="username" class="form-control" required="required"/>
- </div>
- <div class="form-group">
- <label>Password</label>
- <input type="password" name="password" minlength="12" class="form-control" required="required"/>
- </div>
- <div class="form-group">
- <label>Firstname</label>
- <input type="text" name="firstname" class="form-control" required="required"/>
- </div>
- <div class="form-group">
- <label>Lastname</label>
- <input type="text" name="lastname" class="form-control" required="required"/>
- </div>
- <div class="form-group">
- <label>Email</label>
- <input type="email" name="email" class="form-control" required="required"/>
- </div>
- <div class="form-group">
- <button name="register" class="btn btn-primary btn-block">Sign up</button>
- </div>
- <?php
- if($_SESSION['status'] == "error"){
- ?>
- <div class="alert alert-danger"><?php echo $_SESSION['result']?></div>
- <?php
- }
- }
- ?>
- </form>
- </div>
- </div>
- </body>
- </html>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Email Verification Using PHPMailer</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-3"></div>
- <div class="col-md-6">
- <h2>Welcome User</h2>
- </div>
- </div>
- </body>
- </html>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Email Verification Using PHPMailer</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-3"></div>
- <div class="col-md-6">
- <?php
- ?>
- <h3><strong>You're ready to go!</strong></h3>
- <br />
- <h5>Hi, <?php echo $_REQUEST['firstname']." ".$_REQUEST['lastname']?><h5>
- <h5>We've finished setting up your account.<h5>
- <h5>We sent you a confirmation to your email account<h5>
- <a class="btn btn-primary" href="https://<?php echo $_REQUEST['email']?>" target="_blank">Confirm Email</a>
- <?php
- }
- ?>
- </div>
- </div>
- </body>
- </html>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Email Verification Using PHPMailer</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-2"></div>
- <div class="col-md-8">
- <?php
- $email = $_REQUEST['email'];
- ?>
- <center><h4>The email that you provided is already verified.</h4></center>
- <h5>Please let me know if you have any questions or would like further information, otherwise, no response is needed.</h5>
- <h5>You may now login to your account <a href="confirm_account.php?email=<?php echo $email?>">here</a></h5>
- <h5>Thank You!,</h5>
- <?php
- }
- ?>
- </div>
- </div>
- </body>
- </html>
Creating the Login Function
This code contains the login function of the Application. This code will check if the user account is already verified to have permission to login. To this just copy and write these code below inside the text editor, then save it as login.php- <?php
- require_once 'conn.php';
- $username = $_POST['username'];
- $password = $_POST['password'];
- $query = mysqli_query($conn, "SELECT * FROM `user` WHERE `username` = '$username' && `password` = '$password' && `status` = 'Verified'") or die(mysqli_error());
- if($count > 0){
- }else{
- $_SESSION['message'] = "Invalid username or password";
- }
- }
- ?>
Creating Confirm Account
This code contains the confirming of account in the application. This code will confirm the user account to enable the login access. To this just copy and write these block of code inside the text editor, then save it as confirm_account.php- <?php
- require_once 'conn.php';
- $email = $_REQUEST['email'];
- mysqli_query($conn, "UPDATE `user` SET `status` = 'Verified' WHERE `email` = '$email'") or die(mysqli_error());
- }
- ?>
Creating the Main Function
This code contains the main function of the application. This code will sent a confirmation to the user email, to verify if the email account exist. To make this just follow and write these code below inside the text editor, then save it as register.php Note: To make this script work without composer, make sure you change this code:- require 'vendor/autoload.php';
- require 'path/to/PHPMailer/src/Exception.php';
- require 'path/to/PHPMailer/src/PHPMailer.php';
- require 'path/to/PHPMailer/src/SMTP.php';
- <?php
- use PHPMailer\PHPMailer\PHPMailer;
- use PHPMailer\PHPMailer\Exception;
- require_once 'conn.php';
- $username = $_POST['username'];
- $password = $_POST['password'];
- $firstname = $_POST['firstname'];
- $lastname = $_POST['lastname'];
- $email = $_POST['email'];
- mysqli_query($conn, "INSERT INTO `user` VALUES('', '$username', '$password', '$firstname', '$lastname', '$email', '')") or die(mysqli_error());
- $link = "localhost/PHP - Simple Email Verification Using PHPMailer/verified.php?email=".$email."";
- $message = "Hello $firstname $lastname! <br>"
- . "Please click the link below to confirm your email and complete the registration process.<br>"
- . "You will be automatically redirected to a welcome page where you can then sign in.<br><br>"
- . "Please click below to activate your account:<br>"
- . "<a href='$link'>Click Here!</a>";
- //Load composer's autoloader
- require 'vendor/autoload.php';
- $mail = new PHPMailer(true);
- try {
- //Server settings
- $mail->isSMTP();
- $mail->Host = 'smtp.gmail.com';
- $mail->SMTPAuth = true;
- $mail->Username = '[email protected]';
- $mail->Password = 'mypassword';
- 'verify_peer' => false,
- 'verify_peer_name' => false,
- 'allow_self_signed' => true
- )
- );
- $mail->SMTPSecure = 'ssl';
- $mail->Port = 465;
- //Send Email
- $mail->setFrom('[email protected]');
- //Recipients
- $mail->addAddress($email);
- $mail->addReplyTo('[email protected]');
- //Content
- $mail->isHTML(true);
- $mail->Subject = "Account registration confirmation";
- $mail->Body = $message;
- $mail->send();
- header("location:verification.php?firstname=".$firstname."&lastname=".$lastname."&email=".$email."");
- } catch (Exception $e) {
- $_SESSION['result'] = 'Message could not be sent. Mailer Error: '.$mail->ErrorInfo;
- $_SESSION['status'] = 'error';
- }
- }
- ?>
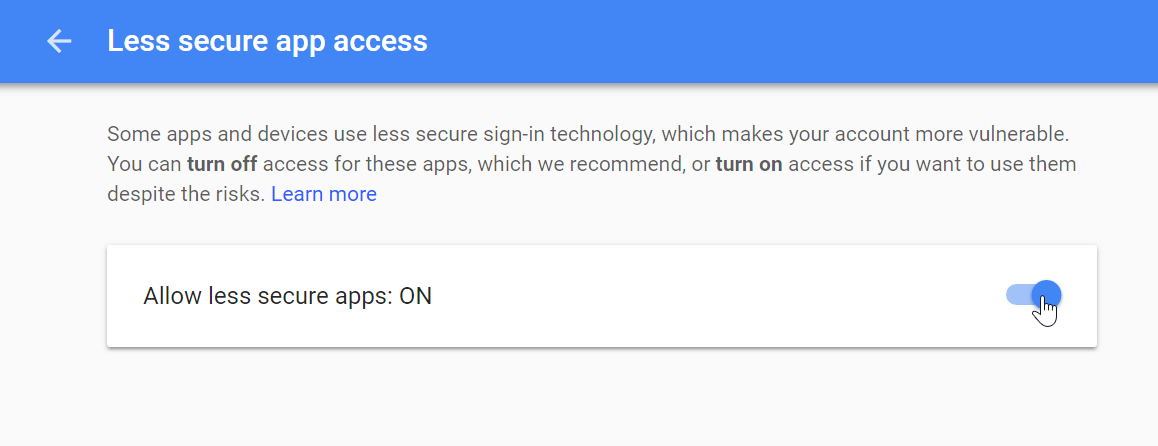