Creating a Live Search in Table using Vue.js and PHP
This tutorial tackles on how to create a search function that searches MySQL
table using Vue.js
with the help of axios.js
and PHP
. In this tutorial, you can search for either the first name or the last name of members in the members' table then it will return members that match your search.
Getting Started
I've used CDN for Bootstrap, Vue.js, and Axios.js so, you need an internet connection for them to work. And also download XAMPP
Creating our Database
- Open your XAMPP's Control Panel and start "Apache" and "MySQL"
- Open
PHPMyAdmin
- Click databases, create a database and name it as
vuetot
- After creating a database, click the
SQL
and paste the below codes. See the image below for detailed instructions.
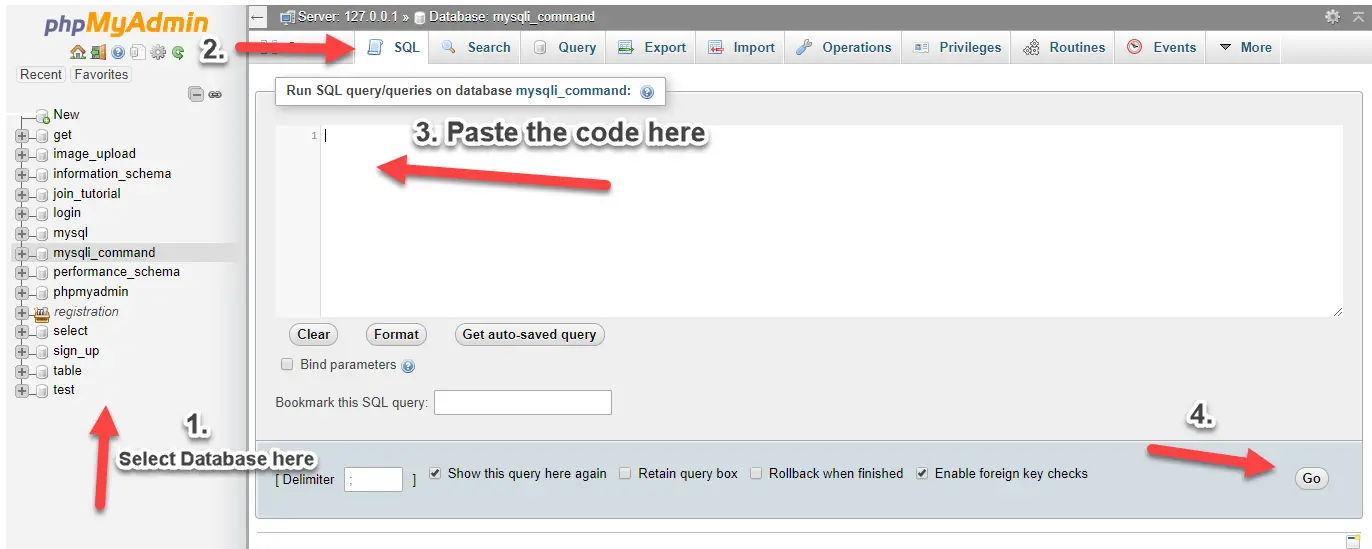
Inserting Data into our Database
Next, we insert sample data into our database to use in our search.
- Click
vuetot
database that we have created earlier. - Click
SQL
and paste the following codes.
- Click Go button below.
Creating the Interface
This contains our sample table and our search input.
index.php
- <!DOCTYPE html>
- <html>
- <head>
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" />
- </head>
- <body>
- <div id="myapp">
- <div class="container">
- <div class="col-md-8 col-md-offset-2">
- <div class="row">
- <div class="col-md-4 col-md-offset-8">
- <input type="text" class="form-control" placeholder="Search Firstname or Lastname" v-on:keyup="searchMonitor" v-model="search.keyword">
- </div>
- </div>
- <table class="table table-bordered table-striped">
- <thead>
- </thead>
- <tbody>
- <tr v-if="noMember">
- </tr>
- <tr v-for="member in members">
- </tr>
- </tbody>
- </table>
- </div>
- </div>
- </div>
- </body>
- </html>
Creating a Script
This contains our vue.js code.
app.js
- var app = new Vue({
- el: '#myapp',
- data:{
- members: [],
- search: {keyword: ''},
- noMember: false
- },
- mounted: function(){
- this.fetchMembers();
- },
- methods:{
- searchMonitor: function() {
- var keyword = app.toFormData(app.search);
- axios.post('action.php?action=search', keyword)
- .then(function(response){
- app.members = response.data.members;
- if(response.data.members == ''){
- app.noMember = true;
- }
- else{
- app.noMember = false;
- }
- });
- },
- fetchMembers: function(){
- axios.post('action.php')
- .then(function(response){
- app.members = response.data.members;
- });
- },
- toFormData: function(obj){
- var form_data = new FormData();
- for(var key in obj){
- form_data.append(key, obj[key]);
- }
- return form_data;
- },
- }
- });
Creating the API
Lastly, this contains our PHP
code in fetching member data from our database.
action.php
- <?php
- $conn = new mysqli("localhost", "root", "", "vuetot");
- if ($conn->connect_error) {
- }
- $action="show";
- $action=$_GET['action'];
- }
- if($action=='show'){
- $sql = "select * from members";
- $query = $conn->query($sql);
- while($row = $query->fetch_array()){
- }
- $out['members'] = $members;
- }
- if($action=='search'){
- $keyword=$_POST['keyword'];
- $sql="select * from members where firstname like '%$keyword%' or lastname like '%$keyword%'";
- $query = $conn->query($sql);
- while($row = $query->fetch_array()){
- }
- $out['members'] = $members;
- }
- $conn->close();
- ?>
DEMO
That ends this tutorial. I help you with what you are looking for and you'll find this tutorial useful for your future web application projects.
Happy Coding :)