Update and Delete Multiple User Account In PHP
Submitted by rinvizle on Wednesday, August 3, 2016 - 15:04.
In this tutorial we will teach you how to create and implement a Update and Delete Multiple User Account from the database using PHP. To select or identify multiple user accounts, we have to used check boxes and passed the ID of each user which were brought from the database to the check box assigned to each user in a html form. To submit the selected check boxes which holds the ID of each user detail for an update or deletion purpose, javascript is used for redirection to the pages with the scripts that performs the actions needed for each operation. See the sample code below.
User_Update.php - This script is for the updating the users acount or information.
User_Deletion.php - This short script of code is for deleting a one or group of users account in the database.
Hope that you learn in this project and enjoy coding. Don't forget to LIKE & SHARE this website.
Sample Code
Index.php - This script of file is compose of PHP and Html Form, this file is for displaying the users accounts from the database through the html form so the user will not go directly in the mysql database and they can easily viewed through the html interface.- <!DOCTYPE html>
- <html>
- <head>
- <title>Update & Delete Multiple User Account In PHP</title>
- <link rel="stylesheet" type="text/css" href="css/styles.css" />
- <script language="javascript" type="text/javascript">
- function update_data()
- {
- document.form_name.action = "users-update.php";
- document.form_name.submit();
- }
- function delete_data()
- {
- if(confirm("Do you really mean to delete the details?"))
- {
- document.form_name.action = "users-deletion.php";
- document.form_name.submit();
- }
- }
- </script>
- </head>
- <?php
- include "config.php";
- ?>
- <body>
- <div style="width:500px; margin:0 auto; margin-top: 100px;">
- <form name="form_name" method="post" action="">
- <div class="header_title" align="center"><h1>Update & Delete Multiple User Account In PHP</h1></div>
- <table border="0" cellpadding="10" cellspacing="1" width="500" class="table_wrapper">
- <tr class="table_header">
- <td>First Name</td>
- <td>Last Name</td>
- <td>Username</td>
- <td>Password</td>
- <td style="text-align:center;">Action</td>
- </tr>
- <?php
- $tclass = "dark";
- {
- ?>
- <td style="text-align:center;"><input type="checkbox" name="users[]" class="users" id="users" value="<?php echo trim(strip_tags($get_data["id"])); ?>" ></td>
- </tr>
- <?php
- }
- ?>
- <tr class="table_header">
- <td colspan="5" align="center">
- <input class="button" type="button" name="update" value="Update" onClick="update_data();" />
- <input class="button" type="button" name="delete" value="Delete" onClick="delete_data();" />
- </td>
- </tr>
- </table>
- </form>
- </div>
- </body>
- </html>
- <?php
- include "config.php";
- {
- echo $counted_users;
- for($i=0; $i<$counted_users; $i++)
- {
- mysql_query("update `user_update` set `firstname` = '".mysql_real_escape_string(trim(strip_tags($_POST["firstname"][$i])))."', `lastname` = '".mysql_real_escape_string(trim(strip_tags($_POST["lastname"][$i])))."', `username` = '".mysql_real_escape_string(trim(strip_tags($_POST["username"][$i])))."', `password` = '".mysql_real_escape_string(trim(strip_tags($_POST["password"][$i])))."' where `id` = '".mysql_real_escape_string(trim(strip_tags($_POST["userId"][$i])))."'");
- }
- }
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>Update & Delete Multiple User Account In PHP</title>
- <link rel="stylesheet" type="text/css" href="css/styles.css" />
- </head>
- <body>
- <div style="width:523px; margin: 0 auto; margin-top: 100px;" align="center">
- <div class="header_title"><h1>Update & Delete Multiple User Account In PHP</h1></div>
- <?php
- if($users_id == 0)
- {
- ?>
- <div style="margin:0 auto; padding:10px; background:#FFFFEA; line-height:20px; border:1px solid #F1F1F1;">It seems you did not check any item to update.<br>Please click on the button below to go back and then check on an item to update.</div><br><br>
- <div align="center" class="button" onClick="window.location.replace('index.php');">Back</div>
- <?php
- }
- else
- {
- ?>
- <table border="0" cellpadding="10" cellspacing="0" width="500" align="center" class="table_wrapper">
- <tr class="table_header">
- <td>Update User Information</td>
- </tr>
- <?php
- for($i=0; $i<$users_id; $i++)
- {
- $users_details = mysql_query("select * from `user_update` where `id` = '".mysql_real_escape_string(trim(strip_tags($_POST["users"][$i])))."'");
- ?>
- <tr>
- <td>
- <table border="0" cellpadding="10" cellspacing="0" width="500" align="center" class="inner_table">
- <tr>
- <td><label>First Name</label></td>
- <td><input type="text" name="firstname[]" class="field" value="<?php echo $getdetail[$i]['firstname']; ?>"></td>
- </tr>
- <tr>
- <td><label>Last Name</label></td>
- <td><input type="text" name="lastname[]" class="field" value="<?php echo $getdetail[$i]['lastname']; ?>"></td>
- </tr>
- <tr>
- <td><label>Username</label></td>
- <td>
- <input type="hidden" name="userId[]" class="field" value="<?php echo $getdetail[$i]['id']; ?>">
- <input type="text" name="username[]" class="field" value="<?php echo $getdetail[$i]['username']; ?>"></td>
- </tr>
- <tr>
- <td><label>Password</label></td>
- <td><input type="password" name="password[]" class="field" value="<?php echo $getdetail[$i]['password']; ?>"></td>
- </tr>
- </table>
- </td>
- </tr>
- <?php
- }
- ?>
- <tr>
- <td colspan="2" align="center"><input type="submit" name="submit" value="Save Changes" class="button"></td>
- </tr>
- </table>
- </form>
- <?php
- }
- ?>
- </div>
- </body>
- </html>
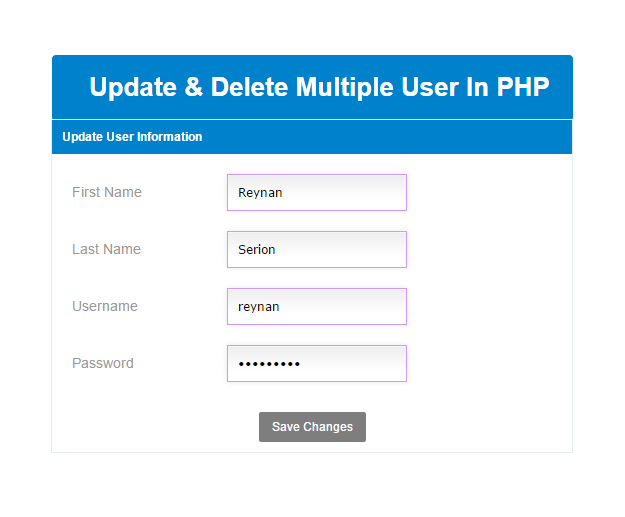
- <?php
- include "config.php";
- for($i=0; $i<$users_id; $i++)
- {
- mysql_query("delete from `user_update` where `id` = '".mysql_real_escape_string(trim(strip_tags($_POST["users"][$i])))."'");
- }
- ?>