How To Create Login Page In PHP/MySQL
Submitted by alpha_luna on Friday, April 29, 2016 - 17:04.
Related Code: How To Create Registration Page In PHP/MySQL
In the previous tutorial, we create on How To Create Registration Page In PHP/MySQL. For the continuation of Registration Page Tutorial it should have a Login Page, that's why I create this tutorial on How To Create Login Page In PHP/MySQL.
This program works by the user to enter their username or email and password to open the new page. This login page also has a log out function, where the user signs out their account after clicking the logout button on the home page. After the user clicks the logout button, the user cannot go back to the previous page. If the user wants to go back to the previous page, the user must log in again to go back on the previous page. So, let's do this.
First, we are going to make our database.
Second, we are going to make our form field.
Third, we are going to make our database connection.
Fourth, we are going to make our saving PHP Script.
Fifth, we are going to make our Home Page.
Output of our Home Page
So, this is it, just follow the steps to have this Login Page or you can download the full source code below by clicking the "Download Code" button below.
Related Code: How To Create Registration Page In PHP/MySQL
Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at [email protected]. Practice Coding. Thank you very much.
Creating our Table
We are going to make our database. To create a database:- Open the PHPMyAdmin
- Create a database and name it as "user_login".
- After creating a database name, click the SQL and kindly copy the code below.
- --
- -- Table structure for table `user`
- --
Creating Form Field
This form field that the user types their username or email and password to log in.- <form method="post" action="signin_form.php" enctype="multipart/form-data">
- <table>
- <tr>
- <td><input type="email" name="email" placeholder="[email protected]" class="form-1" title="Enter your email" required /></td>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- <td colspan="2">
- <input type="submit" name="submit" value="Log in" class="btn-sign-in" title="Log in" />
- <input type="reset" name="cancel" value="Cancel" class="btn-sign-up" title="Cancel" />
- </td>
- </tr>
- </table>
- </form>
Database Connection
This PHP Script is our database. Copy and paste this then save it as "database.php".- <?php
- ?>
Login Script - PHP
- <?php
- include('includes/database.php');
- {
- $email=$_POST['email'];
- $password=$_POST['password'];
- {
- if ($count == 0)
- {
- echo "<script>alert('Please check your username and password!'); window.location='signin.php'</script>";
- }
- else if ($count > 0)
- {
- $_SESSION['id'] = $row['user_id'];
- }
- }
- }
- ?>
Creating Home Page
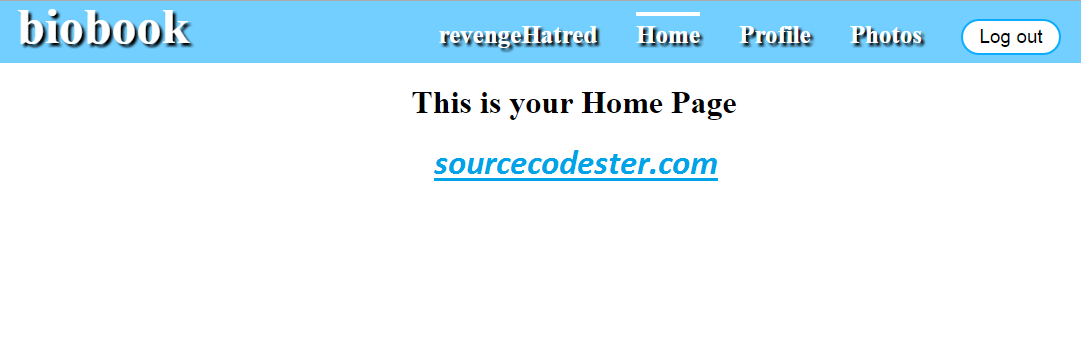