How to Create a Title Border in Java
Submitted by donbermoy on Monday, December 8, 2014 - 09:57.
This tutorial will teach you how to create a border with title in Java. A titled border has a combination of a String/text for title and any borders that put inside its text.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of titleBorder.java.
2. Import the following package library.
3. We will initialize variables in our Main, variable frame as JFrame and button as JButton.
4. Now, we will create first the titled border in the top. We will use the TitledBorder class here and named its variable as topBorder and we will set its position to the top using setTitlePosition method.
Next we will create a font for our title in the bottom border. Tahoma as the font, Italic as the font style, and 12 as the font size.
We will create again the TitledBorder class for the bottom position with right alignment, have its font, and will have its foreground color as red.
To have the bottomBorder visible, we will add it on the button that we have declared. We will use the setBorder method here.
5. Lastly, add the button to the frame with a default BorderLayout of Center position, set its size and visibility, and its close operation. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*; // used to access BorderFactory, JButton, and JFrame class
- import javax.swing.border.*; //used to access the TitledBorder subclass of the border class
- import java.awt.*; // used to access the Font and Color class
- TitledBorder bottomBorder = new TitledBorder(topBorder, "This is a Footer", TitledBorder.RIGHT, TitledBorder.BOTTOM, font, Color.red);
- button.setBorder(bottomBorder);
- frame.getContentPane().add(button, "Center");
- frame.setSize(300, 100);
- frame.setVisible(true);
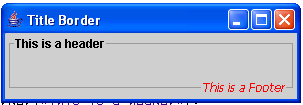
- import javax.swing.*; // used to access BorderFactory, JButton, and JFrame class
- import javax.swing.border.*; //used to access the TitledBorder subclass of the border class
- import java.awt.*; // used to access the Font and Color class
- public class titleBorder {
- TitledBorder bottomBorder = new TitledBorder(topBorder, "This is a Footer", TitledBorder.RIGHT, TitledBorder.BOTTOM, font, Color.red);
- button.setBorder(bottomBorder);
- frame.getContentPane().add(button, "Center");
- frame.setSize(300, 100);
- frame.setVisible(true);
- }
- }