GridLayout as Layout Manager in Java
Submitted by donbermoy on Saturday, November 29, 2014 - 08:35.
This tutorial is about the GridLayout as Layout Manager in Java. A GridLayout is a layout where it has a grid within a component. This layout ignores the sizes of the component and resizes them fit the cell's dimension of the grid.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of gridLayout.java.
2. Import the following packages:
3. Initialize your variable in your Main, variable frame for JFrame, and 6 buttons namely b1,b2,b3,b4,b5, and b6 as JButton.
As you can see there are two parameters of GridLayout class. The 2 indicates the number of rows and the 3 indicates the column of the grid.
When we create a GridLayout object, you indicate the number of rows and columns, and the container will be divided to that grid accordingly.
Then add the buttons into the frame using the add method.
5. Lastly, set the size, visibility, and the close operation of the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*; //used to access of GridLayout class
- import javax.swing.*; //used to access the JFrame and JButton class
4. To have a GridLayout as the layout manager, we will use the setLayout method of the frame.
- frame.getContentPane().add(b1);
- frame.getContentPane().add(b2);
- frame.getContentPane().add(b3);
- frame.getContentPane().add(b4);
- frame.getContentPane().add(b5);
- frame.getContentPane().add(b6);
- frame.setSize(500, 400);
- frame.setVisible(true);
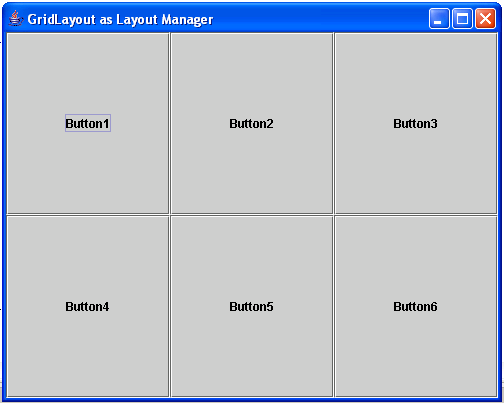
- import java.awt.*; //used to access of GridLayout class
- import javax.swing.*; //used to access the JFrame and JButton class
- public class gridLayout{
- JButton b1, b2, b3, b4, b5, b6;
- frame.getContentPane().add(b1);
- frame.getContentPane().add(b2);
- frame.getContentPane().add(b3);
- frame.getContentPane().add(b4);
- frame.getContentPane().add(b5);
- frame.getContentPane().add(b6);
- frame.setSize(500, 400);
- frame.setVisible(true);
- }
- }