Copy Data to a File into Another File using Java
Submitted by donbermoy on Wednesday, June 4, 2014 - 19:45.
Today in Java, I will teach you how to create a program that copies data of a file into another file using the FileInputStream and FileOutputStream of the IO package.
So, now let's start this tutorial!
1. Open Notepad. Put any data in there, for example, i have write "Sourcecodester is the best!". Save it to the same folder with your java program and named it as data1.txt. Now, create another text file using Notepad and leave it blank. Name this as data2.txt.
2. Open JCreator or NetBeans and make a java program with a file name of copyFile.java.
3. Import java.io package. Hence we will use an input/output in accessing files, FileInputStream, FileOutputStream, and IOException.
4. In your main, create an instance of source and destination files. The source will be your data1.txt as this contains data and the destination is the data2.txt as this is empty.
Create a FileInputStream and FileOutputStream variables and make it empty.
5. Now, create a try and catch method. In your try method, do the following code. This will trigger to copy the contents of data1.txt into the data2.txt.
Make the source file (data1.txt) as FileInputStream and destination file (data2.txt) as FileOutputStream. Define then the size of our buffer for buffering file data, and write the content of your source file into the destination file.
In your Catch method, prefer to catch IOException then use printStackTrace() method. This will help to trace the exception and identify which method causes the bug. And also the FileNotFoundException if the file is not found under the same folder of the program.
Full source code:
Output:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.io.*;
- try {
- byte[] buffer = new byte[4096];
- int read;
- while ((read = fis.read(buffer)) != -1) {
- fos.write(buffer, 0, read);
- }
- }
- {
- e.printStackTrace();
- {
- e.printStackTrace();
- }
- import java.io.*;
- public class copyFile {
- try {
- byte[] buffer = new byte[4096];
- int read;
- while ((read = fis.read(buffer)) != -1) {
- fos.write(buffer, 0, read);
- }
- e.printStackTrace();
- }
- }
- }
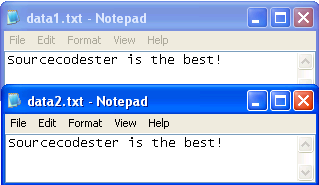