How to Check if a File is Hidden or Not using Java
Submitted by donbermoy on Thursday, May 29, 2014 - 13:48.
In this tutorial, i will teach you how to create a program in java that will check if a file is hidden or not.
So, now let's start this tutorial!
1. Open Notepad. It's up to you if you will write data in there or not. Save it to the same folder with your java program and named it as READ FIRST.txt. and make sure to hide this file.
2. Open JCreator or NetBeans and make a java program with a file name of hiddenFile.java.
3. Import java.io package. Hence the file class is in the input/output library. Import also javax.swing package because we will use JOptionPane.showMessageDialog for displaying the output.
4. In your main, initialize the READ FIRST.txt that you have created a while ago that has a file variable of File class. This will access this file inside the folder of your program.
5. Now, create an if-else statement. In If statement, have the parameter of file.isHidden(). isHidden() method of the file class triggers to check if a file is hidden or not. By default, it is in True in nature as it is a Boolean data type. And it will prompt the user that the file is hidden. Then in Else statement, it will prompt the user that the file is not hidden. Note: READ FIRST.txt is hidden.
Press F5 to run the program.
Output:
full source code:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.io.*;
- import javax.swing.*;
- if (file.isHidden())
- {
- } else
- {
- }
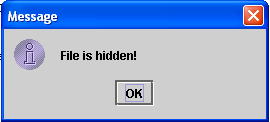
- import java.io.*;
- import javax.swing.*;
- public class hiddenFile
- {
- {
- if (file.isHidden())
- {
- } else
- {
- }
- }
- }
Comments
Add new comment
- Add new comment
- 102 views