C++ Template Functions
Submitted by rinvizle on Thursday, June 30, 2016 - 12:32.
This source code is written in C++ and it is a simple code. The template functions are actually a bit easier to use than template classes, as the compiler can often deduce the desired type from the function's argument list. C++ templates can be used both for classes and for functions in C++.
For instance, to declare a function to add two values together using this syntax.
It is also possible to have a class that has a member function that is itself a template, separate from the class template. Just like this.
The function myFunc is a function inside of a class, and when you actually define the function, you must respect this by using the template keyword twice. And avoid the following attempt to combine the two and it is wrong and it will not work, look at the syntax of badcode at the badcode source code sample.
Sample Code
The syntax for declaring a function is similar to that for a class.template <class type> type func_name(type arg1, arg2, ...);
Now, when you actually use the add function, you can simply treat it like any other function because the desired type is also the type given for the arguments. This means that upon compiling the code, the compiler will know what type is desired.
int x = add(1, 2);
int x = add<int>(1, 2);
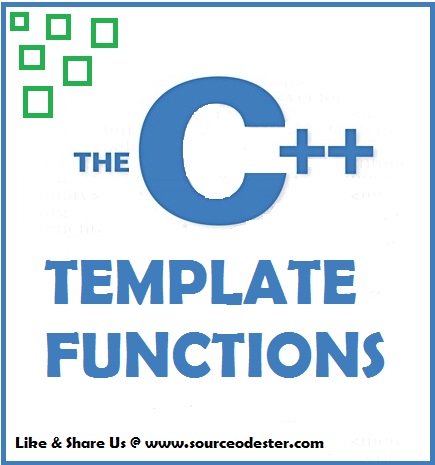
- template <class type> type add(type a, type b)
- {
- return a + b;
- }
- template <class type> class TClass
- {
- // constructors, etc
- template <class type2> type2 myFunc(type2 arg);
- };
- template <class type> // For the class
- template <class type2> // For the function
- type2 TClass<type>::myFunc(type2 arg)
- {
- // code
- }
- // bad code!
- template <class type, class type2> type2 TClass<type>::myFunc(type2 arg)
- {
- // ...
- }
Add new comment
- 330 views