Facade Design Pattern with C#.NET and SQL Server
Submitted by thusitcp on Wednesday, October 8, 2014 - 14:20.
Most of software companies are making high cost software for their clients around the world so software development should become profitable business to development companies. Standard development process, well define cording style always helpful to achieve their business goals. As my experience lot of software has some portion of common occurring problems. Developing same component for each client will be reducing profit of software companies. To overcome above issues now a day’s software companies used reusable component. What we called is software design pattern.
In this tutorial I am going to teach you facade design pattern with c#.net. Facade design pattern provides unified interface to set of subsystems. This will simplified the interface to larger body of the code. So it is commonly used developing class libraries. Façade make software library easy to used, make library more readable and provide well define interface to poorly design code.
Façade design pattern highly used when simple interface required to access complex subsystem, system is very complex and difficult to understand and need entry point to each level of layered software system.
Insert method
Update method
get all method
Facade
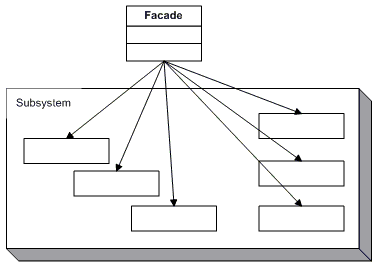
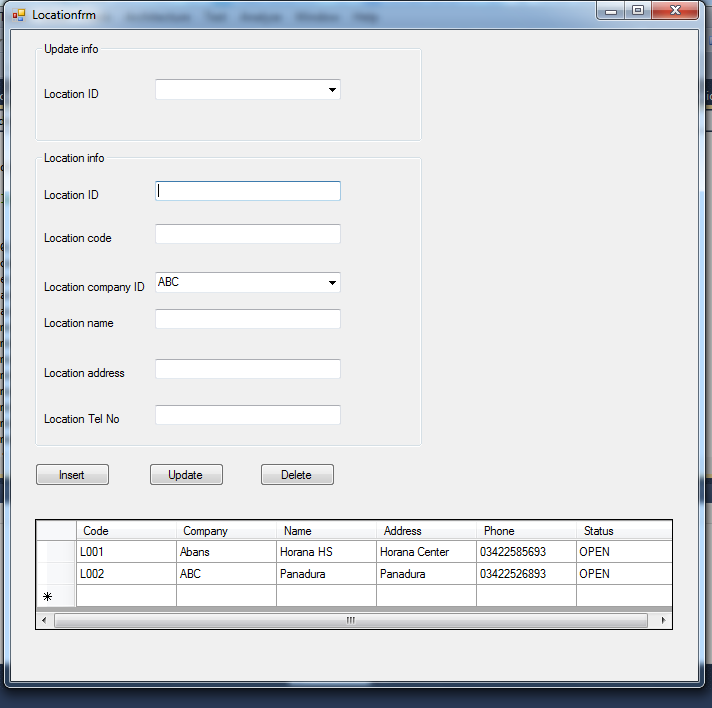
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using Sonic_Core.Data.Core;
- using Sonic_Core.DomainObjects;
- using System.Data.SqlClient;
- using System.Data;
- using Sonic_Core.Exceptions.DataBase;
- namespace Sonic_Core.Data.Commands.Locations
- {
- class InsertLocationDataAction : SoniceDataAction<int>
- {
- Location location_O = null;
- public InsertLocationDataAction(Location location)
- {
- location_O = location;
- }
- public override int Body(System.Data.SqlClient.SqlConnection PConn)
- {
- //throw new NotImplementedException();
- try
- {
- int LVCount = 0;
- LVCommand.Connection = PConn;
- LVCommand.CommandType = CommandType.StoredProcedure;
- LVCommand.CommandText = "[dbo].[sp_tblLocationInsert]";
- LVCommand.Parameters.AddWithValue("@loc_code", location_O.loc_code);
- LVCommand.Parameters.AddWithValue("@loc_com_id", location_O.loc_company_id);
- LVCommand.Parameters.AddWithValue("@loc_name", location_O.loc_name);
- LVCommand.Parameters.AddWithValue("@loc_address", location_O.loc_address);
- LVCommand.Parameters.AddWithValue("@loc_tele_phone", location_O.loc_tele_phone);
- LVCommand.Parameters.AddWithValue("@loc_status", location_O.loc_status);
- LVCount = LVCommand.ExecuteNonQuery();
- return LVCount;
- }
- catch (SonicDatabaseException ex)
- {
- }
- }
- }
- }
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using Sonic_Core.Data.Core;
- using Sonic_Core.DomainObjects;
- using System.Data.SqlClient;
- using System.Data;
- using Sonic_Core.Exceptions.DataBase;
- namespace Sonic_Core.Data.Commands.Locations
- {
- class UpdateLocationDataAction : SoniceDataAction<int>
- {
- Location location_O = null;
- public UpdateLocationDataAction(Location location)
- {
- location_O = location;
- }
- public override int Body(System.Data.SqlClient.SqlConnection PConn)
- {
- //throw new NotImplementedException();
- try
- {
- int LVCount = 0;
- LVCommand.Connection = PConn;
- LVCommand.CommandType = CommandType.StoredProcedure;
- LVCommand.CommandText = "[dbo].[sp_tblLocationUpdate]";
- LVCommand.Parameters.AddWithValue("@loc_id", location_O.loc_id);
- LVCommand.Parameters.AddWithValue("@loc_code", location_O.loc_code);
- LVCommand.Parameters.AddWithValue("@loc_com_id", location_O.loc_company_id);
- LVCommand.Parameters.AddWithValue("@loc_name", location_O.loc_name);
- LVCommand.Parameters.AddWithValue("@loc_address", location_O.loc_address);
- LVCommand.Parameters.AddWithValue("@loc_tele_phone", location_O.loc_tele_phone);
- LVCommand.Parameters.AddWithValue("@loc_status", location_O.loc_status);
- LVCount = LVCommand.ExecuteNonQuery();
- return LVCount;
- }
- catch (SonicDatabaseException ex)
- {
- }
- }
- }
- }
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using Sonic_Core.Data.Core;
- using Sonic_Core.DomainObjects;
- using System.Data.SqlClient;
- using System.Data;
- using Sonic_Core.Exceptions.DataBase;
- namespace Sonic_Core.Data.Commands.Locations
- {
- class GetAllLocations : SoniceDataAction<DataTable>
- {
- Location location_O = null;
- public GetAllLocations()
- {
- }
- public GetAllLocations(Location location)
- {
- location_O = location;
- }
- public override DataTable Body(System.Data.SqlClient.SqlConnection PConn)
- {
- //throw new NotImplementedException();
- tblLocation.Columns.Add("ID");
- tblLocation.Columns.Add("Code");
- tblLocation.Columns.Add("Company ID");
- tblLocation.Columns.Add("Company");
- tblLocation.Columns.Add("Name");
- tblLocation.Columns.Add("Address");
- tblLocation.Columns.Add("Phone");
- tblLocation.Columns.Add("Status");
- try
- {
- //int LVReader = 0;
- LVCommand.Connection = PConn;
- LVCommand.CommandType = CommandType.StoredProcedure;
- LVCommand.CommandText = "[dbo].[sp_tblLocationSelect_By_Status]";
- if (location_O.loc_id == 0)
- {
- LVCommand.Parameters.AddWithValue("@loc_id", location_O.loc_id);
- }
- else
- {
- LVCommand.Parameters.AddWithValue("@loc_id", location_O.loc_id);
- }
- LVCommand.Parameters.AddWithValue("@status", location_O.loc_status);
- SqlDataReader LVReader = LVCommand.ExecuteReader();
- while (LVReader.Read())
- {
- //comp_o = new Sonic_Core.DomainObjects.Company();
- //comp_o._com_id = LVReader.GetInt32(0);
- //comp_o.com_code = LVReader.GetString(1);
- //comp_o.com_name = LVReader.GetString(2);
- //comp_o.com_address = LVReader.GetString(3);
- //comp_o.com_PhoneNo = LVReader.GetString(4);
- //CompList.Add(comp_o);
- tblLocation.Rows.Add(tblLocation.NewRow());
- tblLocation.Rows[tblLocation.Rows.Count - 1][0] = LVReader.GetInt32(0);
- tblLocation.Rows[tblLocation.Rows.Count - 1][1] = LVReader.GetString(1);
- tblLocation.Rows[tblLocation.Rows.Count - 1][2] = LVReader.GetInt32(2);
- tblLocation.Rows[tblLocation.Rows.Count - 1][3] = LVReader.GetString(3);
- tblLocation.Rows[tblLocation.Rows.Count - 1][4] = LVReader.GetString(4);
- tblLocation.Rows[tblLocation.Rows.Count - 1][5] = LVReader.GetString(5);
- tblLocation.Rows[tblLocation.Rows.Count - 1][6] = LVReader.GetString(6);
- tblLocation.Rows[tblLocation.Rows.Count - 1][7] = LVReader.GetString(7);
- }
- return tblLocation;
- }
- catch (SonicDatabaseException ex)
- {
- }
- }
- }
- }
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using Sonic_Core.DomainObjects;
- using Sonic_Core.Data.Commands;
- using System.Data;
- using Sonic_Core.Data.Commands.Locations;
- namespace Sonic_Core.Data.Fascade
- {
- public class LocationDAO
- {
- public static int LocationInsert(Location location)
- {
- return location_DA.execute();
- }
- public static int LocationUpdate(Location location)
- {
- return Location_Upd.execute();
- }
- public static DataTable GetAllLocations(Location location)
- {
- return getLocations.execute();
- }
- public static int DeleteLocationByStatus(Location location)
- {
- return deletLocation.execute();
- }
- }
- }