Get IP Address of a Website using C#
Submitted by donbermoy on Saturday, May 31, 2014 - 00:17.
In this tutorial, i will teach you how to create a program that gets an IP address of a website using C#. When a user inputs the link of a particular website, then the program determines its ip address.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name your program IP Address of a Host.
2. Next, add a TextBox named TextBox1 for the inputting of a website and TextBox2 for displaying its IP address. Insert also a Button named Button1 that will trigger to get the IP address of the website inputted. You must design your interface like this:
3. Import System.Net namespace because this library has the internet and network provider.
4. Now, put this code in Button1_Click. This will trigger to determine the IP address of the site inputted.
Use the IPHostEntry Class to provide a class for Internet host address information and have its variable named hostname. Have its value by the Dns class (Domain Name Service) with GetHostByName method hold the inputted site in Textbox1.
Now, create an IPAddress Class with variable name ip because this will provide an access for IP (Internet Protocol) addresses with the address list of your hostname variable of textbox1.
Then display the output (IP address of the host) in textbox2 by converting the IP address to string.
Full source code:
Press F5 to run the program.
Output:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
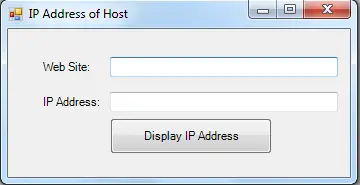
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- using System.Net;
- IPHostEntry hostname = Dns.GetHostByName(TextBox1.Text);
- IPAddress[] ip = hostname.AddressList;
- TextBox2.Text = (string) (ip[0].ToString());
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- using System.Net;
- namespace IP_Address_of_Host
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- IPHostEntry hostname = Dns.GetHostByName(TextBox1.Text);
- IPAddress[] ip = hostname.AddressList;
- TextBox2.Text = (string) (ip[0].ToString());
- }
- }
- }
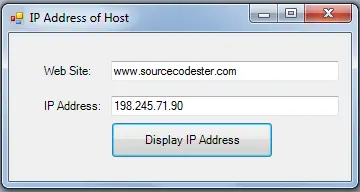