How to Create a Simple Record Navigation in C#
Submitted by joken on Wednesday, April 16, 2014 - 19:00.
This tutorial is a continuation of our last topic called “How to Display Selected Row from Datagridview into Textbox using C#”. But this time, I’m going to show you how to create a simple record navigation using C#. This will help the user to navigate through all the record from the database easily. To start this application open our last project called “student_info”, then add another button and arrange it that looks like as shown below.
Then we will add two lines of code below this line of code
Next, we are going to create a subroutine called “Navigate()”. We call this subroutine when we navigate through the datatable. And this subroutine will be called when the previous and next button is clicked. And here’s the code for this subroutine.
This time we proceed to the “Load All Data” button and let’s modify the by adding new lines of code. And then here’s the new modified code for “Load All Data” button.
Next, we will add a code for our Previous button.this previous will simply do the decrementing the row number of the Datatable by one. And here’s the code for “Previous” button.
And for the “Next” will simply increment the row number of Datatable by one.And here’s the code for “Next” Button.
This time, you can test the application by pressing the “F5” or the “Start” button. And it should look like as shown below.
And here’s all the code used for this application.
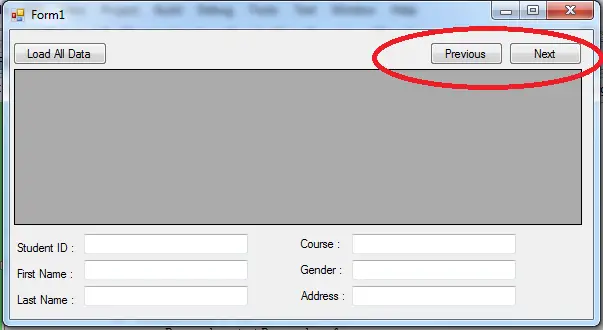
OleDbConnection conn = new OleDbConnection(connection);
.
In this new line of code, we are just simply declaring an integer variable that has an initial value of zero.
- int curRecord = 0;
- int totRecord = 0;
- private void Navigate()
- {
- txtid.Text = dt.Rows[curRecord][0].ToString();
- txtfname.Text = dt.Rows[curRecord][1].ToString();
- txtlname.Text = dt.Rows[curRecord][2].ToString();
- txtcourse.Text = dt.Rows[curRecord][3].ToString();
- txtgender.Text = dt.Rows[curRecord][4].ToString();
- txtaddress.Text = dt.Rows[curRecord][5].ToString();
- }
- private void btnload_Click(object sender, EventArgs e)
- {
- //create a new datatable
- //create our SQL SELECT statement
- string sql = "Select * from tblstudent";
- //then we execute the SQL statement against the Connection using OleDBDataAdapter
- //we fill the result to dt which declared above as datatable
- da.Fill(dt);
- //set the curRecord to zero
- curRecord = 0;
- //get the total number of record available in the database and stored to totRecord Variable
- totRecord = dt.Rows.Count;
- //then we populate the datagridview by specifying the datasource equal to dt
- dataGridView1.DataSource = dt;
- }
- private void btnprev_Click(object sender, EventArgs e)
- {
- //decrement the curRecord
- curRecord--;
- if (curRecord < 0)
- curRecord = totRecord - 1;
- //call subroutine
- Navigate();
- }
- private void btnnext_Click(object sender, EventArgs e)
- {
- curRecord++;
- if (curRecord >= totRecord)
- curRecord = 0;
- Navigate();
- }
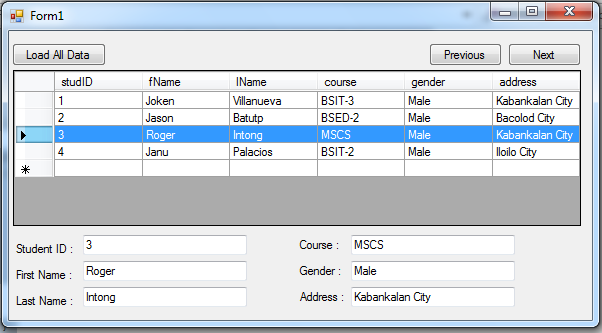
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Linq;
- using System.Text;
- using System.Windows.Forms;
- using System.Data.OleDb;
- namespace student_info
- {
- public partial class Form1 : Form
- {
- //declare new variable named dt as New Datatable
- //this line of code used to connect to the server and locate the database (usermgt.mdb)
- static string connection = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source= " + Application.StartupPath + "/studentdb.mdb";
- int curRecord = 0;
- int totRecord = 0;
- public Form1()
- {
- InitializeComponent();
- }
- private void btnload_Click(object sender, EventArgs e)
- {
- //create a new datatable
- //create our SQL SELECT statement
- string sql = "Select * from tblstudent";
- //then we execute the SQL statement against the Connection using OleDBDataAdapter
- //we fill the result to dt which declared above as datatable
- da.Fill(dt);
- //set the curRecord to zero
- curRecord = 0;
- //get the total number of record available in the database and stored to totRecord Variable
- totRecord = dt.Rows.Count;
- //then we populate the datagridview by specifying the datasource equal to dt
- dataGridView1.DataSource = dt;
- }
- private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
- {
- //it checks if the row index of the cell is greater than or equal to zero
- if (e.RowIndex >= 0)
- {
- //gets a collection that contains all the rows
- DataGridViewRow row = this.dataGridView1.Rows[e.RowIndex];
- //populate the textbox from specific value of the coordinates of column and row.
- txtid.Text = row.Cells[0].Value.ToString();
- txtfname.Text = row.Cells[1].Value.ToString();
- txtlname.Text = row.Cells[2].Value.ToString();
- txtcourse.Text = row.Cells[3].Value.ToString();
- txtgender.Text = row.Cells[4].Value.ToString();
- txtaddress.Text = row.Cells[5].Value.ToString();
- }
- }
- private void Form1_Load(object sender, EventArgs e)
- {
- }
- private void Navigate()
- {
- txtid.Text = dt.Rows[curRecord][0].ToString();
- txtfname.Text = dt.Rows[curRecord][1].ToString();
- txtlname.Text = dt.Rows[curRecord][2].ToString();
- txtcourse.Text = dt.Rows[curRecord][3].ToString();
- txtgender.Text = dt.Rows[curRecord][4].ToString();
- txtaddress.Text = dt.Rows[curRecord][5].ToString();
- }
- private void btnprev_Click(object sender, EventArgs e)
- {
- //decrement the curRecord
- curRecord--;
- if (curRecord < 0)
- curRecord = totRecord - 1;
- //call subroutine
- Navigate();
- }
- private void btnnext_Click(object sender, EventArgs e)
- {
- curRecord++;
- if (curRecord >= totRecord)
- curRecord = 0;
- Navigate();
- }
- }
- }
Add new comment
- 434 views