In this tutorial, im going to show you how to connect Microsoft Access Database in C#. First we should create a new database in MS Access named “usermgt”, then create new table named “tbluseraccounts” and the design the table fields that looks like as shown below.
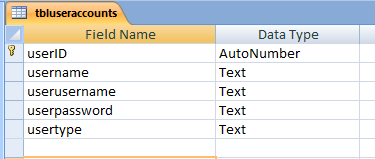
After this step, save the table and add one record to the table and it should look like as shown below.

At this time, we will now create a new project in
C#, for me I am using Visual studio 2008. Then here’s my step on how to create a C# in visual studion 2008. First open the visual studio and click file then choose new project.
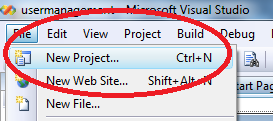
And on the new project, follow the all the step based on the figure shown below.
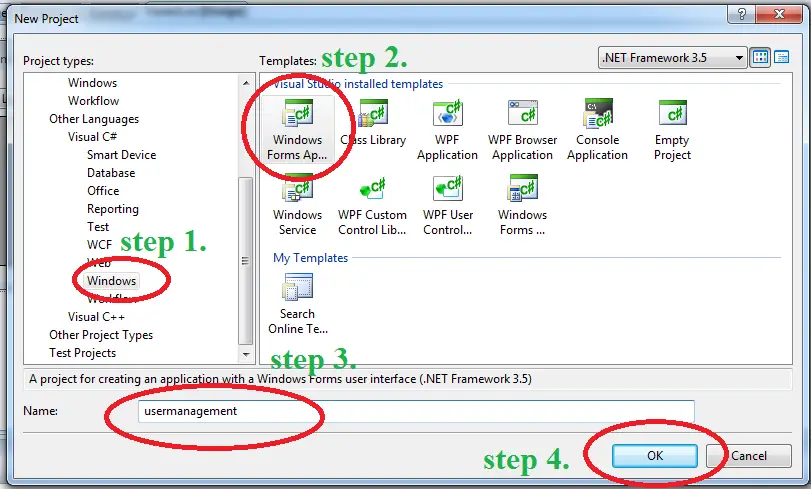
By the way folks in this application we are creating a “User Management System”. After you performed all the steps above, you may now design your application that looks like as the figure shown below.
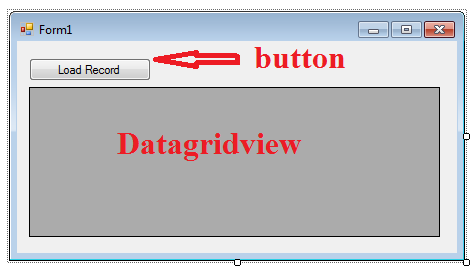
At this time, before we proceed on adding the code for connecting the Database in
C# we will copy first the database file inside the bin folder of our project. So that it will be easy for us later to connect it to our application.
After of all the steps weve done, I think its time for us to move to our main topic on how to connect the database in C#. First double click the form. And add this “
using System.Data.OleDb;” single line of code above “
namespace WindowsFormsApplication1” and it should be look like as shown below.
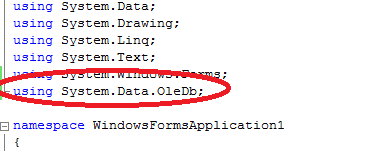
The new added code is a .NET Framework Data Provider for
OLE DB describes a collection of classes used to access an
OLE DB data source in the managed space.
Our next step is to set a new variable named “dt” as
Datatable that will be used later on. And in this step also, we will connect to the server and connect to the database named “usermgt”. And the code will look like as shown below.
public partial class Form1 : Form
{
//declare new variable named dt as New Datatable
DataTable dt = new DataTable();
//this line of code used to connect to the server and locate the database (usermgt.mdb)
static string connection = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source= " + Application .StartupPath + "/usermgt.mdb";
OleDbConnection conn = new OleDbConnection(connection);
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
}
}
Then at this time, we’re going to add code for the ”
Load record” button. To do this, double click the button and add the following code.
loadrecord();//calling the function Loadrecord
This code wre added is a function that will load all the record from our database to the datagridview. And we will now add a code for the our function named “
loadrecord()”. And here’s the following code:
private void loadrecord()
{
string sql = "Select * from tbluseraccounts";
OleDbDataAdapter da = new OleDbDataAdapter(sql , conn);
da.Fill(dt);
dataGridView1.DataSource = dt;
}
Then here’s all the code for this application.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.OleDb;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
//declare new variable named dt as New Datatable
DataTable dt = new DataTable();
//this line of code used to connect to the server and locate the database (usermgt.mdb)
static string connection = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source= " + Application .StartupPath + "/usermgt.mdb";
OleDbConnection conn = new OleDbConnection(connection);
public Form1()
{
InitializeComponent();// calling the function
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void loadrecord()
{
string sql = "Select * from tbluseraccounts";
OleDbDataAdapter da = new OleDbDataAdapter(sql , conn);
da.Fill(dt);
dataGridView1.DataSource = dt;
}
private void button1_Click(object sender, EventArgs e)
{
loadrecord();
}
}
}
And after completing all the steps above. You may now press “
F5” or start button. And when the user clicked the “
Load record” Button, it will look like as shown below.
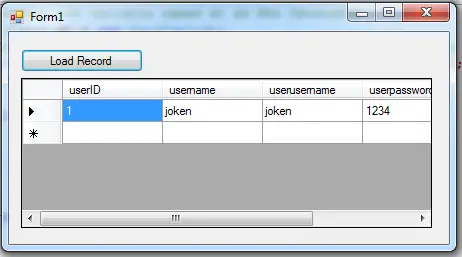