How to Drag External File in the ListView Using C#
Submitted by janobe on Tuesday, June 11, 2019 - 17:15.
This time, I will teach you how to drag an external file in the ListView using C#. By using this kind of method this allows you to easily drag and drop an image in the Listview. You might encounter this kind of problem in programming, but you will only achieve it once you follow the steps below.
The complete sourcecode is included. You can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.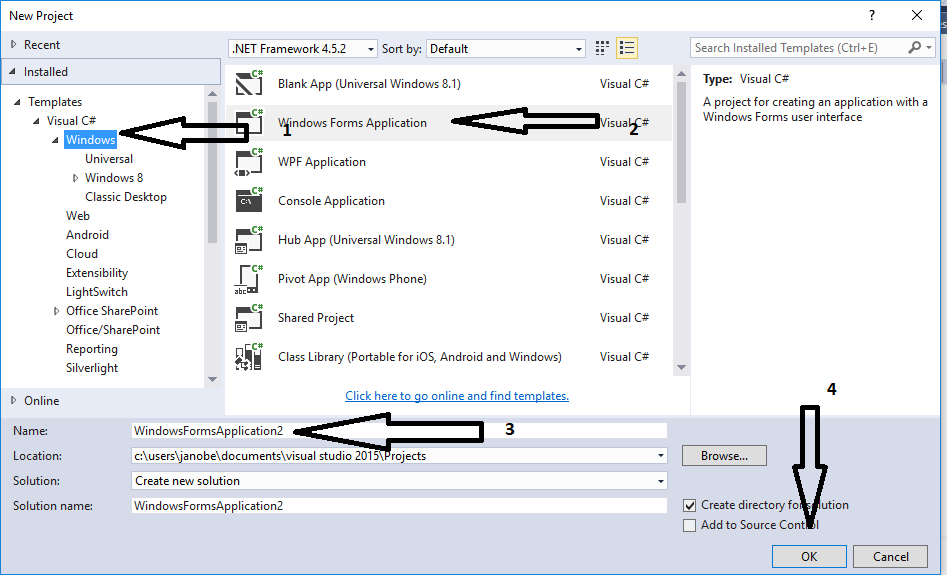
Step 2
Add a ListView and PictureBox inside the Form just like shown below.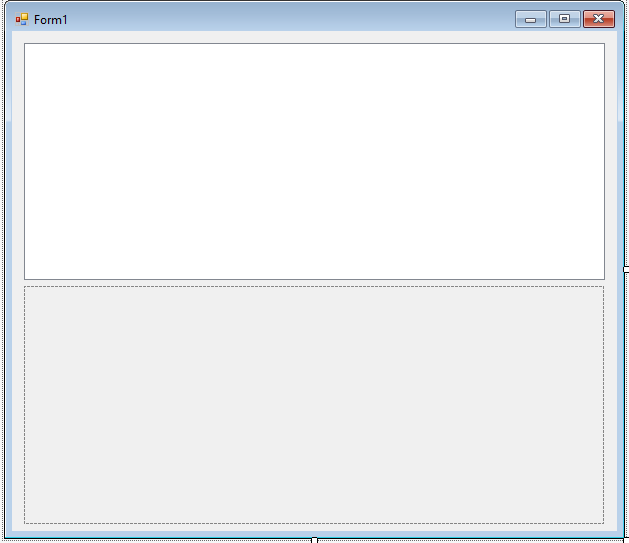
Step 3
Press F7 to open the code editor. In the code editor, declare an item in the listview control.- ListViewItem lstviewItem;
Step 4
Double click the form and add the following code to setup a ListView and PictureBox properties in the first load of a form.- listView1.AllowDrop = true;
- listView1.View = View.Details;
- listView1.AllowDrop = true;
- listView1.Columns.Add("File");
- listView1.Columns[0].Width = 500;
- pictureBox1.SizeMode = PictureBoxSizeMode.StretchImage;
Step 5
Write the following code for theDragEnter
event of the ListView.
- private void listView1_DragEnter(object sender, DragEventArgs e)
- {
- if (e.Data.GetDataPresent(DataFormats.FileDrop, false) == true)
- {
- e.Effect = DragDropEffects.All;
- }
- }
Step 6
Write the following code for theDragDrop
event of the ListView.
- private void listView1_DragDrop(object sender, DragEventArgs e)
- {
- try
- {
- string[] file;
- file = (string[])e.Data.GetData(DataFormats.FileDrop);
- foreach(string files in file)
- {
- listView1.Items.Add(lstviewItem);
- }
- }
- catch(Exception ex)
- {
- MessageBox.Show("error : " + ex.Message);
- }
- }
Step 7
Double click the ListView and do the following code to display the image in the PictureBox when the ListViewItem is selected.- if (listView1.SelectedItems.Count == 0) return;
- pictureBox1.Image = Image.FromFile(listView1.SelectedItems[0].Text);
- this.Text = listView1.SelectedItems[0].Text;
Comments
Add new comment
- Add new comment
- 222 views