How to Find Hash of File in Terminal Console using Python
In this tutorial, we’ll learn how to program "How to Find the Hash of a File in Python." We’ll focus on accurately hashing the targeted file into a secure sequence of characters. The objective is to achieve a proper hash of a given file format. I'll provide a sample program to demonstrate the coding process, making it easy to understand and implement. So, let’s get started with coding!
This topic is straightforward to understand. Just follow the instructions I provide, and you'll be able to complete it with ease. The program I'll show you demonstrates the correct way to hash a file. I'll also provide a simple and efficient method to achieve this effectively. So, let’s start coding!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- import hashlib
- def compute_file_hash(file_path, algorithm='sha256'):
- hash_func = hashlib.new(algorithm)
- with open(file_path, 'rb') as file:
- while chunk := file.read(8192):
- hash_func.update(chunk)
- return hash_func.hexdigest()
- def main():
- while True:
- print("\n================= Find Hash of File =================\n\n")
- file_path = input("Enter the full filename: ")
- print("\n")
- algorithm = input("Enter the hash algorithm (e.g., md5, sha1, sha256): ")
- print("\n")
- try:
- file_hash = compute_file_hash(file_path, algorithm)
- print(f"The {algorithm} hash of the file is: {file_hash}")
- except FileNotFoundError:
- print("File not found. Please enter a valid file path.")
- except ValueError:
- print(f"Invalid hash algorithm: {algorithm}. Please enter a valid algorithm (e.g., md5, sha1, sha256).")
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
- if __name__ == "__main__":
- main()
This program calculates the cryptographic hash of a file based on a specified algorithm (such as MD5, SHA-1, or SHA-256). It reads the file in binary mode in chunks, updating the hash until the file is fully processed, and then returns the file's hash digest in hexadecimal format. The user can specify the hash algorithm and file path, and the program will validate both inputs, catching cases where the file doesn’t exist or the algorithm name is invalid. After each hash calculation, the user has the option to repeat the process or exit.
Output:
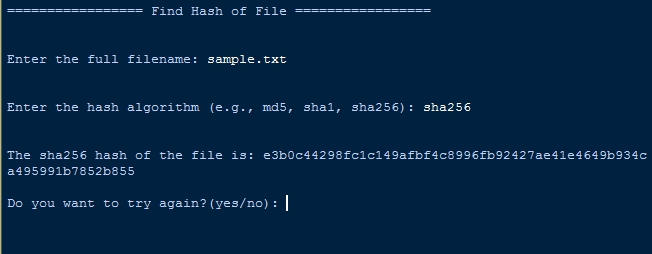
There you have it we successfully created How to Find Hash of File in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 49 views