Converting Celsius to Fahrenheit in Python
Converting Celsius to Fahrenheit in Python
Introduction
In this tutorial we will create a Converting Celsius to Fahrenheit in Python. This tutorial purpose is to help you create a program that can convert the Celsius to Fahrenheit. This will cover all the basic parts for creating the conversion. I will provide a sample program to show the actual coding of this tutorial.
This tutorial will make it easy for you to understand just follow my instruction I provided and you can do it without a problem. This program is useful if you know the value of the temperature. I will try my best to give you the easiest way of creating this program Temperature Conversion. So let's do the coding.
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Importing Modules
After setting up the installation and the database, run the IDLE and click file and then new file. After that a new window will appear containing a black file this will be the text editor for the python. Then copy code that I provided below and paste it inside the IDLE text editor.- from tkinter import *
Setting up the Main Frame
After importing the modules, we will now then create the main frame for the application. To do that just copy the code below and paste it inside the IDLE text editor.- root = Tk()
- root.title("Converting Celsius to Fahrenheit")
- width = 700
- height = 200
- screen_width = root.winfo_screenwidth()
- screen_height = root.winfo_screenheight()
- x = (screen_width/2) - (width/2)
- y = (screen_height/2) - (height/2)
- root.geometry("%dx%d+%d+%d" % (width, height, x, y))
- root.resizable(0, 0)
- root.config(bg="white")
Creating Main Function
This is the main function of the application is. This code will create the Temperature converter complete functionality. To do this just copy and write these block of codes inside the IDLE text editor.- def CelciusToFahren():
- FAHRENHEIT.set("")
- if CELCIUS.get() != "":
- FAHRENHEIT.set((float(CELCIUS.get())*(9/5)) + 32)
- def FahrenToCelcius():
- CELCIUS1.set("")
- if FAHRENHEIT1.get() != "":
- CELCIUS1.set((float(FAHRENHEIT1.get()) - 32) *( 5/9))
- CELCIUS = StringVar()
- CELCIUS1 = StringVar()
- FAHRENHEIT = StringVar()
- FAHRENHEIT1 = StringVar()
In the given code we created a two method for converting the temperature, Celsius to Fahrenheit and Fahrenheit to Celsius. To get the value Celsius to Fahrenheit we only use a simple formula using °F = °C × (9/5) + 32. And to get the Fahrenheit to Celsius value we use this formula °C = 5/9 x (F-32).
Designing the Layout
After creating the Functions we will now add some layout to the application. Just kindly copy the code below and paste it inside the IDLE text editor.- Top = Frame(root, width=700, bd=1, relief=SOLID)
- Top.pack(side=TOP)
- BottomTitle = Frame(root, width=700, bd=1, relief=SOLID)
- BottomTitle.pack(side=TOP, pady=10)
- BottomForm = Frame(root, width=700, bg="white")
- BottomForm.pack(side=TOP)
- BottomLeft = Frame(BottomForm, width=250, bd=1, relief=SOLID)
- BottomLeft.pack(side=LEFT)
- BottomRight = Frame(BottomForm, width=250, bd=1, relief=SOLID)
- BottomRight.pack(side=RIGHT, padx=10)
- lbl_title = Label(Top, text="Converting Celsius to Fahrenheit", width=700 ,font=('arial', 18))
- lbl_title.pack(fill=X)
- lbl3 = Label(BottomLeft, text="Celcius To Fahrenheit",font=('arial', 12))
- lbl3.grid(row=0, columnspan=5, column=0)
- lbl4 = Label(BottomRight, text="Fahrenheit To Celcius",font=('arial', 12))
- lbl4.grid(row=0, columnspan=5, column=0)
- lbl_celcius = Label(BottomLeft, text="Celcius", font=(12))
- lbl_celcius.grid(row=1, padx=5, pady=10)
- lbl_celcius1 = Label(BottomRight, text="Celcius", font=(12))
- lbl_celcius1.grid(row=1, column=3 )
- lbl_to2 = Label(BottomLeft, text="-")
- lbl_to2.grid(row=1, column=2)
- lbl_to3 = Label(BottomRight, text="-")
- lbl_to3.grid(row=1, column=2)
- lbl_fahrenheit = Label(BottomLeft, text="Fahrenheit", font=(12))
- lbl_fahrenheit.grid(row=1, column=3)
- lbl_fahrenheit1 = Label(BottomRight, text="Fahrenheit", font=(12))
- lbl_fahrenheit1.grid(row=1, padx=5, pady=10)
- celcius1 = Entry(BottomLeft, textvariable=CELCIUS, width=12)
- celcius1.grid(row=1, column=1)
- celcius2 = Entry(BottomRight, textvariable=CELCIUS1, width=12, state=DISABLED)
- celcius2.grid(row=1, column=4, padx=5)
- fahrenheit1 = Entry(BottomLeft, textvariable=FAHRENHEIT, width=12, state=DISABLED)
- fahrenheit1.grid(row=1, column=4, padx=5)
- fahrenheit2 = Entry(BottomRight, textvariable=FAHRENHEIT1, width=12)
- fahrenheit2.grid(row=1, column=1)
- btn3 = Button(BottomLeft, text="Convert", width=20, bg="white", command=CelciusToFahren)
- btn3.grid(row=2, columnspan=5, column=0, pady=10)
- btn4 = Button(BottomRight, text="Convert", width=20, bg="white", command=FahrenToCelcius)
- btn4.grid(row=2, columnspan=5, column=0, pady=10)
Initializing the Application
After finishing the function save the application as index.py. This function will run the code and check if the main is initialize properly. To do that copy and write these block code after the main function inside the IDLE text editor.
- if __name__ == '__main__':
- root.mainloop()
Output:
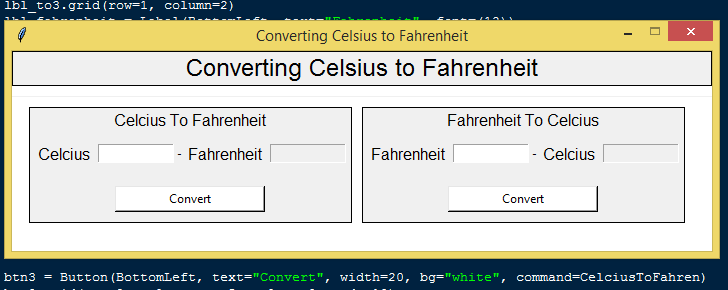
The Converting Celsius to Fahrenheit in Python source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created Converting Celsius to Fahrenheit in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language