How to Dynamically Update an Array from Array Object in JavaScript
How to Dynamically Update an Array from Array Object in JavaScript
Introduction
In this tutorial we will create a How to Dynamically Update an Array from Array Object in JavaScript. This tutorial purpose is to show you how to update your array object data dynamically. This will cover all the basic function that will update your array data. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any system or application that use array as your temporary data storage. I will give my best to provide you the easiest way of creating this program Update array object. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will display the html form and tables with array object. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-8">
- <table class="table table-bordered">
- <thead class="alert-success">
- <tr>
- </tr>
- </thead>
- </table>
- </div>
- <div class="col-md-4">
- <form action="javascript:void(0);" method="POST" class="form-inline" id="update">
- <input type="text" id="edit_name" class="form-control"/>
- </form>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will dynamically update the data of an array object when the button is clicked. To do this just copy and write these block of codes inside the text editor and save it as script.js.- var names = ["John Smith", "Joseph Marcus", "Claire Temple"];
- displayArray();
- Cancel();
- function displayArray() {
- let data = '';
- if (names.length > 0) {
- for (i = 0; i < names.length; i++) {
- data += '<tr>';
- data += '<td>' + names[i] + '</td>';
- data += '<td colspan="2"><center><button class="btn btn-warning" onclick="updateArray(' + i + ')"><span class="glyphicon glyphicon-edit"></span> Edit</button></center></td>';
- data += '</tr>';
- }
- }
- document.getElementById('result').innerHTML = data;
- };
- function updateArray(item) {
- let el = document.getElementById('edit_name');
- el.value = names[item];
- document.getElementById('edit_name').removeAttribute("disabled");
- document.getElementById('btn_update').removeAttribute("disabled");
- document.getElementById('btn_cancel').removeAttribute("disabled");
- self = this;
- document.getElementById('update').onsubmit = function() {
- let name = el.value;
- if (name) {
- self.names.splice(item, 1, name.trim());
- self.displayArray();
- Cancel();
- }
- }
- };
- function Cancel() {
- document.getElementById('edit_name').setAttribute("disabled", "disabled");
- document.getElementById('edit_name').value="";
- document.getElementById('btn_update').setAttribute("disabled", "disabled");
- document.getElementById('btn_cancel').setAttribute("disabled", "disabled");
- }
In the code above we will first set the variable for our array object called names. Next is to display our array object into the html page. We will create a method called displayArray(), this function purpose is to only append the array data into the html element. Lastly we will create a method that will update the array object called updateArray(). This function will update the array object data based on the targeted index position. We use the function splice() to update the data of array object.
Output:
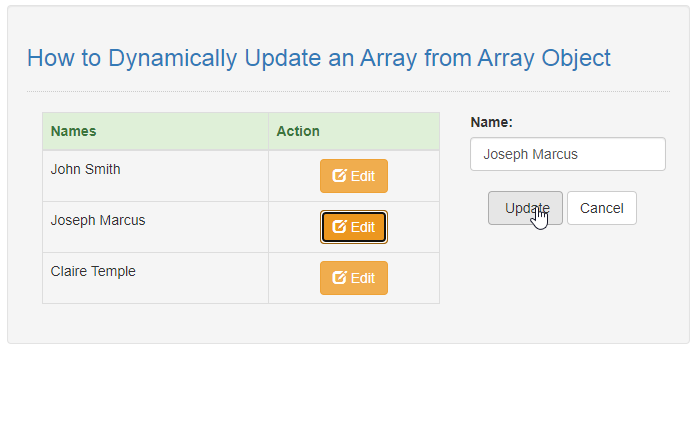
The How to Dynamically Update an Array from Array Object in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Dynamically Update an Array from Array Object in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language