How to Count Number of Words in a String using JavaScript
How to Count Number of Words in a String using JavaScript
Introduction
In this tutorial we will create a How to Count Number of Words in a String using JavaScript. This tutorial purpose is to teach you on how to count the words you are typing. This will cover all the basic function that will count the number of entered words. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any publishing system that needed to track the word that you typed. I will give my best to provide you the easiest way of creating this program Count number of words. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will only display form inputs and the status detail. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="form-group">
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will automatically count the words you are typing. To do this just copy and write these block of codes inside the text editor and save it as script.js.- function countNumWords() {
- var text = document.getElementById("wordInput").value;
- var wordCount;
- if(text == ""){
- wordCount = 0;
- }else{
- var regex = /\s+/gi;
- wordCount = text.trim().replace(regex, ' ').split(' ').length;
- }
- document.getElementById("count").innerHTML = wordCount;
- }
In this code we only create one method called countNumWords(), this will be the function we will use to count the words. In the first line of code we only create two the variables that only bind the text input and an empty one. We use a conditional statement to track if there is a word enterer if not the word count will remain zero, otherwise it display the counter. In order to track the word that needed to be count we create a variable that will set the special regex, this the function that will only target the word we are entering.
Output:
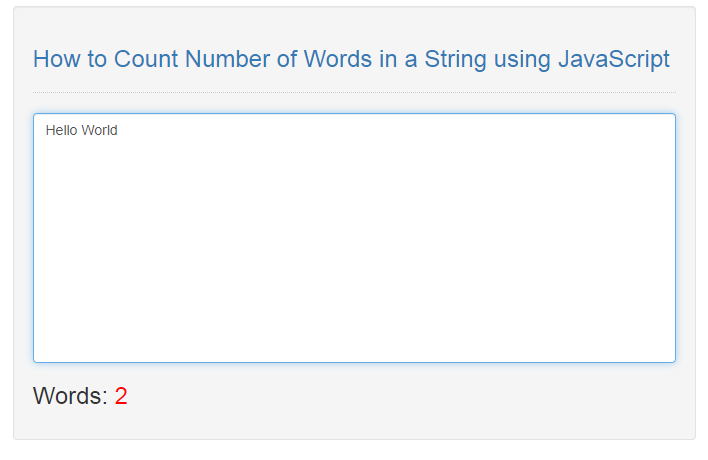
The How to Count Number of Words in a String using JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Count Number of Words in a String using JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language
Add new comment
- 973 views