How to Filter Object Array Based on Attributes in JavaScript
What is JavaScript Array?
Arrays are a special type of objects where typeof operator in JavaScript returns "object" for array. It is a container object that holds a fixed number of values of a single type. It also enables storing a collection of multiple items under a single variable name.Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will display an interface that will list the array object in the HTML page. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css" />
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="container-fluid">
- <div class="row">
- <div class="col-md-6 well">
- <hr style="border-top: 1px SOLID #8c8b8b;"/>
- </div>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will dynamically filter the array object base on the given attribute conditional method. To do this just copy and write these block of codes inside the text editor and save it as script.js.- var array = {
- 'mobile': [{
- "mobile_id": "1",
- "price": "600",
- "brand": "samsung",
- "model": "Samsung S22",
- }, {
- "mobile_id": "2",
- "price": "750",
- "brand": "apple",
- "model": "Iphone 14",
- },{
- "mobile_id": "3",
- "price": "850",
- "brand": "samsung",
- "model": "Galaxy Z Fold 4",
- },{
- "mobile_id": "4",
- "price": "450",
- "brand": "vivo",
- "model": "Vivo V23",
- },
- ]
- };
- function reset(){
- document.getElementById("display").innerHTML=JSON.stringify(array, null, 2);
- }
- function filter(){
- let price=document.getElementById("price").value;
- if(typeof price !== 'undefined' && price){
- let newArray = array.mobile.filter(function (el) {
- return el.price >= price
- });
- document.getElementById("display").innerHTML=JSON.stringify(newArray, null, 2);
- }else{
- alert("Please enter price first!");
- }
- }
- document.getElementById("display").innerHTML=JSON.stringify(array, null, 2);
In this code we will display first the array object using the JSON.stringify() function into the html page. To filter the array object we will first bind the button and input text then we use the filter() function that will basically outputs all the element object that pass a specific test or satisfies a specific function. In my example I filter out the price data where the value is greater than the listed value.
Output:
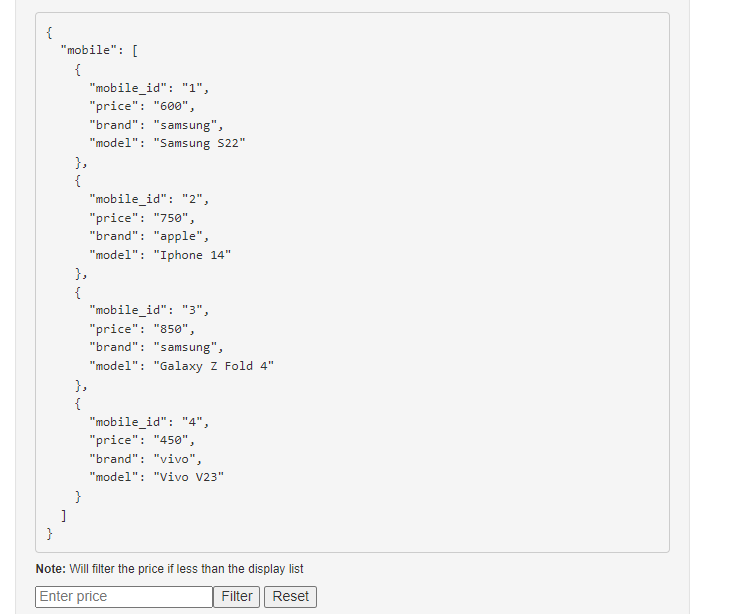
The How to Filter Object Array Based on Attributes in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Filter Object Array Based on Attributes in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language