How $_GET Variable Works and How to Use It in PHP/MySQL
Submitted by nurhodelta_17 on Sunday, August 20, 2017 - 19:56.
Language
This tutorial will show you how does $_GET method works and how to use it. $_GET is a kind of method in getting a particular value in the webpage's url. This will be possible by sending this particular variable in the url of the "go to" page. In this tutorial, I will show you how to send a value in your url and getting that particular value via $_GET method.
Creating our Database
First, we're going to create a database that contains the user data. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as "get". 3. After creating a database, click the SQL and paste the below code. See image below for detailed instruction.- CREATE TABLE `user` (
- `userid` INT(11) NOT NULL AUTO_INCREMENT,
- `firstname` VARCHAR(30) NOT NULL,
- `lastname` VARCHAR(30) NOT NULL,
- PRIMARY KEY (`userid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
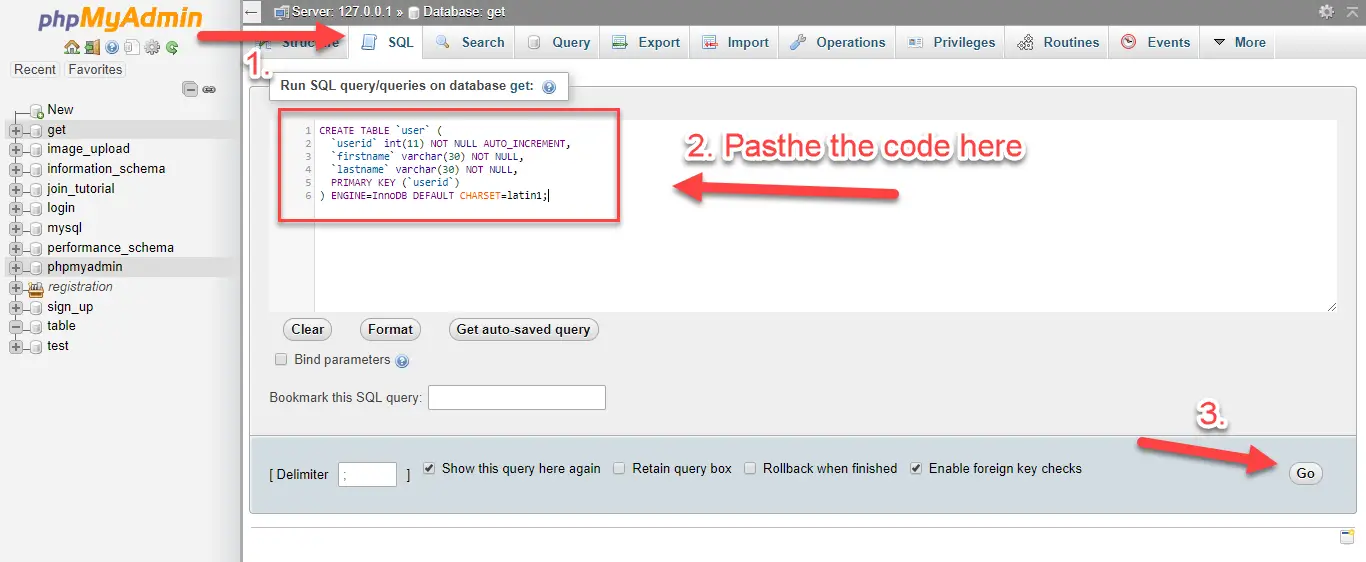
Inserting Data into our Database
Next, we insert data into our database. These data will serve as our reference. 1. Click our database "get". 2. Click SQL and paste the below code.- INSERT INTO `user` (`firstname`, `lastname`) VALUES
- ( 'neovic', 'devierte'),
- ( 'lee', 'ann');
Creating our Connection
Next step is to create a database connection and save it as "conn.php". This file will serve as our bridge between our page and our database. To create the file, open your HTML code editor and paste the code below after the tag.- <?php
- // Check connection
- {
- }
- ?>
Creating our Reference Table
Next step is to create our reference table and name it as "index.php". This table contains the data that we inserted in our database earlier. To create the table, open your HTML code editor and paste the code below after the tag.Creating our Go to Page
Lastly, we create our go to page and name it as "goto.php". This page will received the value that we are sending and get that value using the $_GET method. To create the page, open your HTML code editor and paste the code below after the tag.Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.