Creating a Simple Sign Up Form with Validation in PHP/MySQLi Tutorial
This tutorial will show you how to create a simple sign-up form with validation using PHP/MySQLi. This tutorial does not include a good design but will give you an idea of how to create a simple Sign Up form using PHP/MySQLi. The sign-up form that we will create has a validation that returns messages in each field that has a value that hasn't reach the input field requirement such as null values and email type.
Before we start, please download and install XAMPP first or any equivalent to run PHP Script in you local machine. If you are using XAMPP/WAMP open the software Control Panel and start Apache
and MySQL
.
Let's Get Started ...
Creating our Database
First, we're going to create a database that will store our data.
- Open
phpMyAdmin
. - Click databases, create a database and name it as
sign_up
. - After creating a database, click the
SQL
andpaste
the below code. See the image below for detailed instructions.
- CREATE TABLE `user` (
- `userid` INT(11) NOT NULL AUTO_INCREMENT,
- `username` VARCHAR(30) NOT NULL,
- `password` VARCHAR(30) NOT NULL,
- `email_add` VARCHAR(100) NOT NULL,
- `fullname` VARCHAR(100) NOT NULL,
- PRIMARY KEY (`userid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
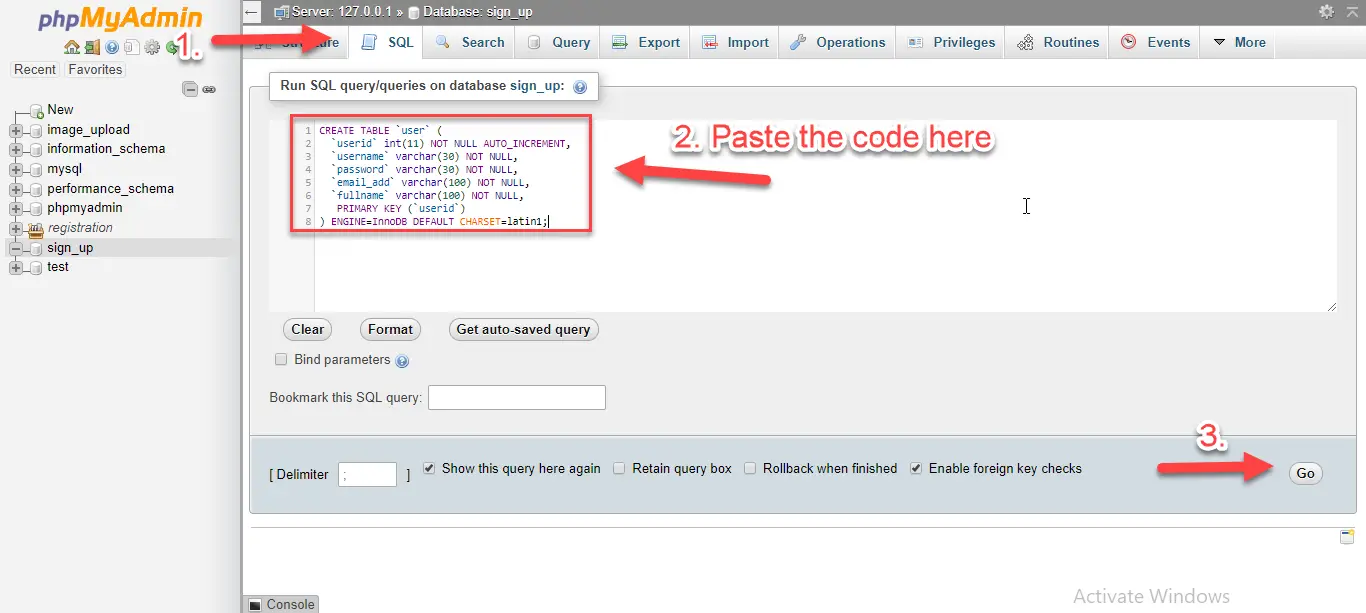
Creating our Connection
Next, we create a database connection and save it as conn.php
. This file will serve as the bridge between our form and our database.
- <?php
- // Check connection
- {
- }
- ?>
Creating our Form and Save Sign Up Script
Lastly, we create our sign-up form with the save script and save it as index.php
. In this form, the inputted data will be saved upon user submission if there are no errors in the input. To create the form, open your HTML code editor and paste the code below after the tag.
- <!DOCTYPE HTML>
- <html>
- <head>
- <title>Register and Login Form with Validation PHP, MySQLi</title>
- <style>
- .error {color: #FF0000;}
- </style>
- </head>
- <body>
- <?php
- // define variables and set to empty values
- $Message = $ErrorUname = $ErrorPass = $ErrorEmail = $ErrorName = "";
- $username = $password = $email = $fullname = "";
- if ($_SERVER["REQUEST_METHOD"] == "POST") {
- $ErrorUname = "Userame is required";
- } else {
- $username = check_input($_POST["username"]);
- // check if name only contains letters and whitespace
- $ErrorUname = "Space and special characters not allowed but you can use underscore(_).";
- }
- else{
- $fusername=$username;
- }
- }
- $ErrorPass = "Password is required";
- } else {
- $fpassword = check_input($_POST["password"]);
- }
- $ErrorEmail = "Email is required";
- } else {
- $email = check_input($_POST["email"]);
- // check if e-mail address is well-formed
- $ErrorEmail = "Invalid email format";
- }
- else{
- $femail=$email;
- }
- }
- $ErrorName = "Full name is required";
- } else {
- $fullname = check_input($_POST["fullname"]);
- // check if name only contains letters and whitespace
- $ErrorName = "Only letters and white space allowed";
- }
- else{
- $ffullname=$fullname;
- }
- }
- if ($ErrorUname!="" OR $ErrorPass!="" OR $ErrorEmail!="" OR $ErrorName!=""){
- $Message = "Registration failed! Errors found";
- }
- else{
- include('conn.php');
- mysqli_query($conn,"insert into `user` (username,password,email_add,fullname) values ('$fusername','$fpassword','$femail','$ffullname')");
- $Message = "Registration Successful!";
- }
- }
- function check_input($data) {
- return $data;
- }
- ?>
- <h2>Sign Up Form</h2>
- <p><span class="error">* required field.</span></p>
- Username: <input type="text" name="username" value="<?php echo isset($_POST['username']) ? $_POST['username'] : "" ?>">
- <span class="error">* <?php echo $ErrorUname;?></span>
- <br><br>
- Password: <input type="password" name="password" value="<?php echo isset($_POST['password']) ? $_POST['password'] : "" ?>">
- <span class="error">* <?php echo $ErrorPass;?></span>
- <br><br>
- Email: <input type="text" name="email" value="<?php echo isset($_POST['email']) ? $_POST['email'] : "" ?>">
- <span class="error">* <?php echo $ErrorEmail;?></span>
- <br><br>
- Name: <input type="text" name="fullname" value="<?php echo isset($_POST['fullname']) ? $_POST['fullname'] : "" ?>">
- <span class="error">* <?php echo $ErrorName;?></span>
- <br><br>
- <input type="submit" name="submit" value="Submit">
- <br><br>
- <span class="error"><?php echo $Message;?></span>
- </form>
- </body>
- </html>
DEMO
There you go. You can now test the source code. I hope this Simple Sign Up Form with Validation in PHP/MySQLi Tutorial will help you with what you are looking for and also for your future PHP Projects.
Happy Coding :))Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.