How to have a Center Screen Window in Java
- import java.awt.*; //used to access the Dimension and Toolkit class
- import javax.swing.*; //used to access the JFrame class
- frame.setBounds(wndSize.width / 4, wndSize.height / 4, // Position
- wndSize.width / 2, wndSize.height / 2); // Size
- frame.setVisible(true); // Display the window
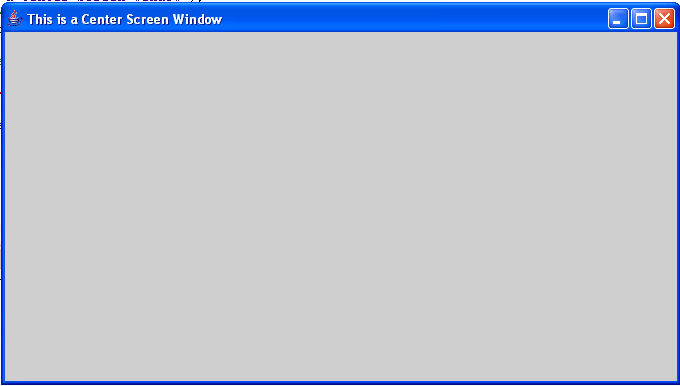
- import java.awt.*; //used to access the Dimension and Toolkit class
- import javax.swing.*; //used to access the JFrame class
- public class centerScreen {
- // Set the position to screen center & size to half screen size
- frame.setBounds(wndSize.width / 4, wndSize.height / 4, // Position
- wndSize.width / 2, wndSize.height / 2); // Size
- frame.setVisible(true); // Display the window
- }
- }
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Add new comment
- 110 views