Checking Account Balance in Java Application
Submitted by GeePee on Wednesday, May 27, 2015 - 22:28.
The following Java Program shows how to Check Account Balance. This program calculates a customer’s checking account balance at the end of the month. . I will be using the JCreator IDE in developing the program.
To start in this tutorial, first open the JCreator IDE, click new and paste the following code.
Sample Run:
The algorithm of the program is:
The first line of data shows the account number, followed by the account balance at the beginning of the month.
Thereafter, each line has two entries: the transaction code and the transaction amount.
The transaction code ‘W’ or ‘w’ means withdrawal, ‘D’ or ‘d’ means deposit, and ‘I’ or ‘I’ means interests paid by the bank.
The program updates the balance after each transaction.
If at any time during the month the balance goes $1000.00, a $25.00 service fee is charged for the month.
The program prints the following information: account number, balance at the beginning of the month, balance at the end of the month, interest paid by the bank, total amount of deposit, number of deposits, total amount of withdrawal, number of withdrawals, and service charge if any.
- import java.io.*;
- import java.util.*;
- public class CheckingAccountBalance
- {
- static final double MINIMUM_BALANCE = 1000.00;
- static final double SERVICE_CHARGE = 25.00;
- throws FileNotFoundException
- {
- int acctNumber;
- double beginningBalance;
- double accountBalance;
- double amountDeposited = 0.0;
- int numberOfDeposits = 0;
- double amountWithdrawn = 0.0;
- int numberOfWithdrawals = 0;
- double interestPaid = 0.0;
- char transactionCode;
- double transactionAmount;
- boolean isServiceCharged = false;
- acctNumber = inFile.nextInt();
- beginningBalance = inFile.nextDouble();
- accountBalance = beginningBalance;
- while (inFile.hasNext())
- {
- transactionCode = inFile.next().charAt(0);
- transactionAmount = inFile.nextDouble();
- switch (transactionCode)
- {
- case 'D':
- case 'd':
- accountBalance = accountBalance + transactionAmount;
- amountDeposited = amountDeposited + transactionAmount;
- numberOfDeposits++;
- break;
- case 'I':
- case 'i':
- accountBalance = accountBalance + transactionAmount;
- interestPaid = interestPaid + transactionAmount;
- break;
- case 'W':
- case 'w':
- accountBalance = accountBalance - transactionAmount;
- amountWithdrawn = amountWithdrawn + transactionAmount;
- numberOfWithdrawals++;
- if ((accountBalance < MINIMUM_BALANCE) && (!isServiceCharged))
- {
- accountBalance = accountBalance - SERVICE_CHARGE;
- isServiceCharged = true;
- }
- break;
- default:
- + transactionCode + " "
- + transactionAmount);
- }
- }
- outFile.printf("Account Number: %d%n", acctNumber);
- outFile.printf("Beginning Balance: $%.2f %n", beginningBalance);
- outFile.printf("Ending Balance: $%.2f %n", accountBalance);
- outFile.println();
- outFile.printf("Interest Paid: $%.2f %n", interestPaid);
- outFile.printf("Amount Deposited: $%.2f %n", amountDeposited);
- outFile.printf("Number of Deposits: $%.2f %n", numberOfDeposits);
- outFile.println();
- outFile.printf("Amount Withdraw: $%.2f %n", amountWithdrawn);
- outFile.printf("Number of Withdrawals: %d%n", numberOfWithdrawals);
- outFile.println();
- if (isServiceCharged)
- outFile.printf("Service Charge: $%.2f %n", SERVICE_CHARGE);
- outFile.close();
- }
- }
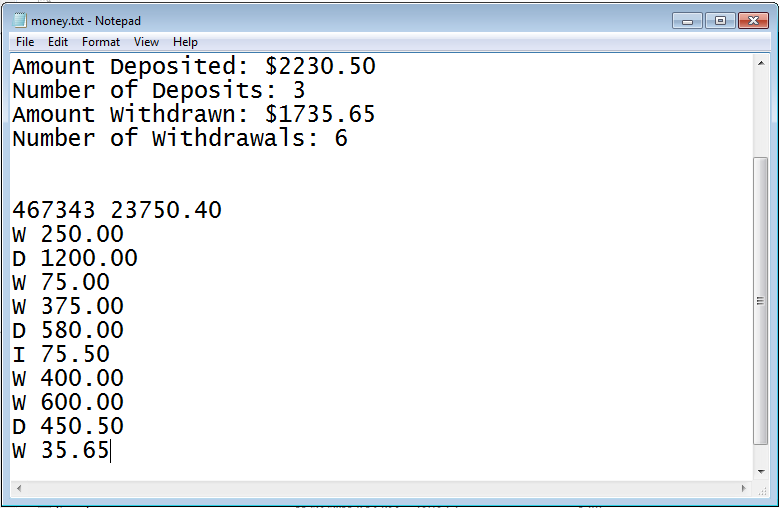
Comments
Add new comment
- Add new comment
- 4269 views