Sign up Form with Email Verification in PHP/MySQLi Tutorial
This tutorial will teach you how to send email/verification email in PHP/MySQLi using the mail() function. The function will both work in local server(ex. XAMMP) or hosted server(online) but in case you wanted to send an email via the local server, you need to modify your local server to allow it to send an email. In my case, I'm using XAMMP, so, I'm going to show you how to set up your XAMMP to send an email.
Setting up Local Server to Send Email
Configure php.ini
- Open php.ini in C:\xampp\php\php.ini.
- Find extension=php_openssl.dll and remove the semicolon from the beginning of that line.
- Find
[mail function]
, enable the ff. by removing the semicolon at the start of the line then, change their values:- SMTP=smtp.gmail.com
- smtp_port=465
- sendmail_from = [email protected]
- sendmail_path = "C:\xampp\sendmail\sendmail.exe -t"
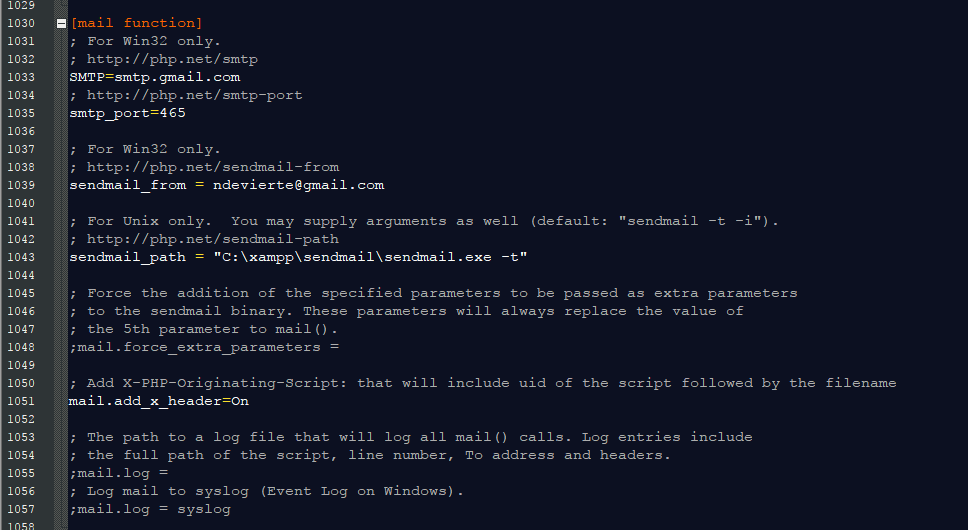
sendmail.ini
- Open sendmail.ini in C:\xampp\sendmail\sendmail.ini
- Find [sendmail], enable the ff. by removing the semicolon at the start of the line, then change their values:
- smtp_server=smtp.gmail.com
- smtp_port=465
- [email protected]
- auth_password=yourpassword
- [email protected]
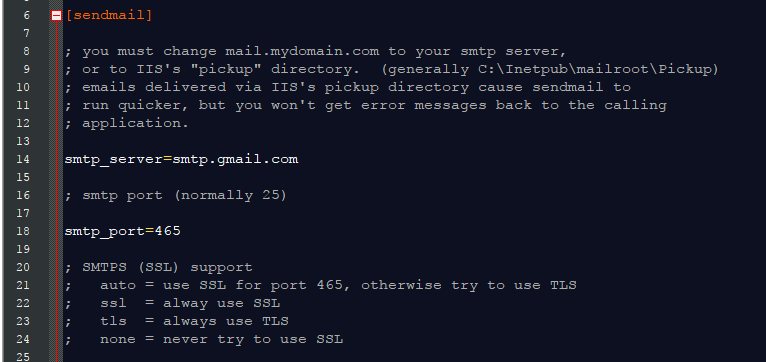
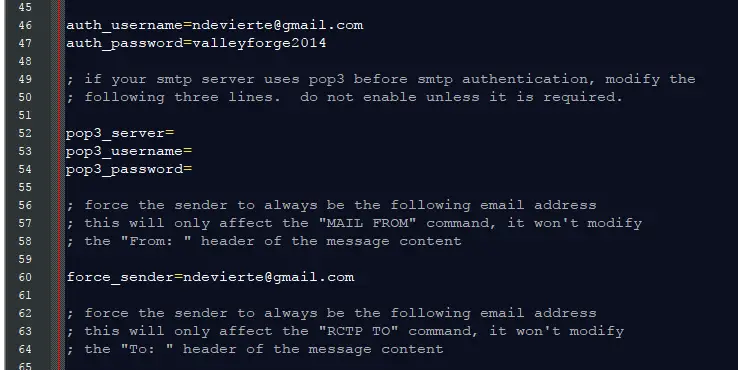
Lastly. make sure to turn this on to allow XAMMP to send an email to your email. Use this link.
And you're done. Restart XAMMP and you should now be able to send email using your XAMMP.
Working Example
To test if your XAMMP is working, here's our example. We will create a registration with a verification email.
Creating our Database
The first step is to create our database.- Open phpMyAdmin.
- Click databases, create a database and name it as sample.
- After creating a database, click the SQL and paste the below codes. See the image below for detailed instructions.
- CREATE TABLE `user` (
- `userid` INT(11) NOT NULL AUTO_INCREMENT,
- `email` VARCHAR(50) NOT NULL,
- `password` VARCHAR(50) NOT NULL,
- `code` VARCHAR(20) NOT NULL,
- `verify` INT(1) NOT NULL,
- PRIMARY KEY(`userid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
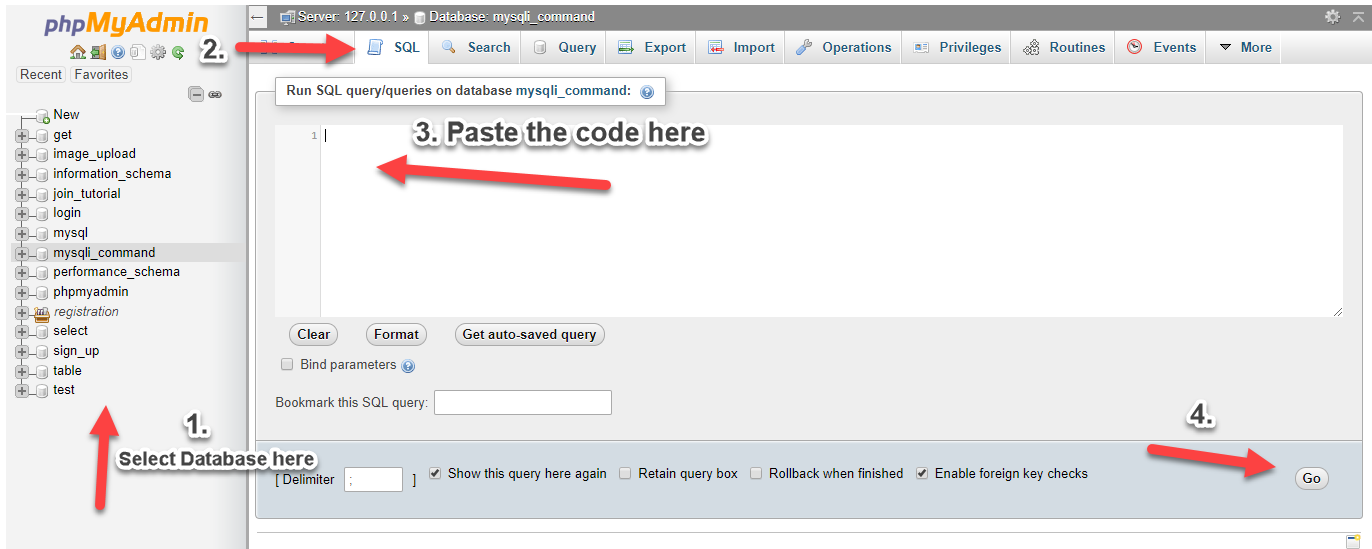
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as conn.php
.
- <?php
- if (!$conn) {
- }
- ?>
index.php
This contains our login form but you can access a link here if you want to sign up.
- <?php
- include('conn.php');
- }
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>Sign up Form with Email Verification in PHP/MySQLi</title>
- <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
- <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js"></script>
- <link href="https://fonts.googleapis.com/css?family=Open+San">
- <link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css">
- <style>
- #login_form{
- width:350px;
- position:relative;
- top:50px;
- margin: auto;
- padding: auto;
- }
- </style>
- </head>
- <body>
- <div class="container">
- <div id="login_form" class="well">
- <h2><center><span class="glyphicon glyphicon-lock"></span> Please Login</center></h2>
- <hr>
- <form method="POST" action="login.php">
- Email: <input type="text" name="email" class="form-control" required>
- <div style="height: 10px;"></div>
- Password: <input type="password" name="password" class="form-control" required>
- <div style="height: 10px;"></div>
- <button type="submit" class="btn btn-primary"><span class="glyphicon glyphicon-log-in"></span> Login</button> No account? <a href="signup.php"> Sign up</a>
- </form>
- <div style="height: 15px;"></div>
- <?php
- ?>
- <div style="height: 30px;"></div>
- <div class="alert alert-danger">
- <span><center>
- <?php echo $_SESSION['log_msg'];
- ?>
- </center></span>
- </div>
- <?php
- }
- ?>
- </div>
- </div>
- </body>
- </html>
signup.php
This is our sign-up form.
- <!DOCTYPE html>
- <html>
- <head>
- <title>Sign up Form with Email Verification in PHP/MySQLi</title>
- <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
- <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js"></script>
- <link href="https://fonts.googleapis.com/css?family=Open+San">
- <link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css">
- <style>
- #signup_form{
- width:350px;
- position:relative;
- top:50px;
- margin: auto;
- padding: auto;
- }
- </style>
- </head>
- <body>
- <div class="container">
- <div id="signup_form" class="well">
- <h2><center><span class="glyphicon glyphicon-user"></span> Sign Up</center></h2>
- <hr>
- <form method="POST" action="register.php">
- Email: <input type="text" name="email" class="form-control" required>
- <div style="height: 10px;"></div>
- Password: <input type="password" name="password" class="form-control" required>
- <div style="height: 10px;"></div>
- <button type="submit" class="btn btn-primary"><span class="glyphicon glyphicon-save"></span> Sign Up</button> <a href="index.php"> Back to Login</a>
- </form>
- <?php
- ?>
- <div style="height: 40px;"></div>
- <div class="alert alert-danger">
- <span><center>
- <?php echo $_SESSION['sign_msg'];
- ?>
- </center></span>
- </div>
- <?php
- }
- ?>
- </div>
- </div>
- </body>
- </html>
register.php
This contains our signup code as well as our send verification email code.
- <?php
- include('conn.php');
- if ($_SERVER["REQUEST_METHOD"] == "POST") {
- function check_input($data){
- return $data;
- }
- $email=check_input($_POST['email']);
- $_SESSION['sign_msg'] = "Invalid email format";
- }
- else{
- $_SESSION['sign_msg'] = "Email already taken";
- }
- else{
- //depends on how you set your verification code
- $set='123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
- mysqli_query($conn,"insert into user (email, password, code) values ('$email', '$password', '$code')");
- //default value for our verify is 0, means it is unverified
- //sending email verification
- $to = $email;
- $subject = "Sign Up Verification Code";
- $message = "
- <html>
- <head>
- <title>Verification Code</title>
- </head>
- <body>
- <h2>Thank you for Registering.</h2>
- <p>Your Account:</p>
- <p>Email: ".$email."</p>
- <p>Password: ".$_POST['password']."</p>
- <p>Please click the link below to activate your account.</p>
- <h4><a href='http://localhost/send_mail/activate.php?uid=$uid&code=$code'>Activate My Account</h4>
- </body>
- </html>
- ";
- //dont forget to include content-type on header if your sending html
- $headers = "MIME-Version: 1.0" . "\r\n";
- $headers .= "Content-type:text/html;charset=UTF-8" . "\r\n";
- "CC: [email protected]";
- $_SESSION['sign_msg'] = "Verification code sent to your email.";
- }
- }
- }
- ?>
activate.php
The goto page from registered email. This is our verification page.
- <?php
- include('conn.php');
- $user=$_GET['uid'];
- $code=$_GET['code'];
- if($row['code']==$code){
- //activate account
- ?>
- <p>Account Verified!</p>
- <p><a href="index.php">Login Now</a></p>
- <?php
- }
- else{
- $_SESSION['sign_msg'] = "Something went wrong. Please sign up again.";
- }
- }
- else{
- }
- ?>
login.php
Our login code will check if the user has successfully signed up and verified its account.
- <?php
- include('conn.php');
- if ($_SERVER["REQUEST_METHOD"] == "POST") {
- function check_input($data) {
- return $data;
- }
- $email=check_input($_POST['email']);
- $_SESSION['log_msg'] = "Invalid email format";
- }
- else{
- $_SESSION['log_msg'] = "User not found";
- }
- else{
- if($row['verify']==0){
- $_SESSION['log_msg'] = "User not verified. Please activate account";
- }
- else{
- $_SESSION['id']=$row['userid'];
- }
- }
- }
- }
- ?>
home.php
This page will show if the user that login is verified.
- <!DOCTYPE html>
- <html>
- <head>
- <link href="https://fonts.googleapis.com/css?family=Open+San">
- <link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css">
- </head>
- <body>
- <div class="container">
- </div>
- </body>
- </html>
logout.php
Lastly, our logout will destroy our login session.
- <?php
- ?>
That ends this tutorial. If you have any questions or comments, feel free to write them below or message me.
Happy Coding :)Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.