Today, you will learn
how to save Image in Access Database using VB.Net. This project will illustrate how to save an object in the field with a data type of “
OLE Object” in the
Access database. In this process, the
image itself will be automatically saved in the database when the button is clicked. See the procedure below to see how it works.
Creating an Application
Step 1
Open
Microsoft Visual Studio 2015 and create a new windows form application in visual basic.
Step 2
Do the form just like shown below.
Step 3
Press
F7 to open the code editor. In the code editor, add a namespace for
OLeDB
to access
OLeDB
libraries and .
Imports System.Data.OleDb
Step 4
Create a
connection between the access database and c#. After that,
declare all the classes and variables that are needed.
Dim con As OleDbConnection = New OleDbConnection("Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" & Application.StartupPath & "\dbsaveimg.accdb")
Dim cmd As OleDbCommand
Dim sql As String
Step 5
Create a method for
saving an image in the database.
Private Sub saveImage(sql As String)
Try
Dim arrImage() As Byte
Dim mstream As New System.IO.MemoryStream()
'SPECIFIES THE FILE FORMAT OF THE IMAGE
PictureBox1.Image.Save(mstream, System.Drawing.Imaging.ImageFormat.Jpeg)
'RETURNS THE ARRAY OF UNSIGNED BYTES FROM WHICH THIS STREAM WAS CREATED
arrImage = mstream.GetBuffer()
'GET THE SIZE OF THE STREAM IN BYTES
Dim FileSize As UInt32
FileSize = mstream.Length
'CLOSES THE CURRENT STREAM AND RELEASE ANY RESOURCES ASSOCIATED WITH THE CURRENT STREAM
mstream.Close()
con.Open()
cmd = New OleDbCommand
With cmd
.Connection = con
.CommandText = sql
.Parameters.AddWithValue("@img", arrImage)
.ExecuteNonQuery()
End With
Catch ex As Exception
MsgBox(ex.Message)
Finally
con.Close()
End Try
End Sub
Step 6
Write the following code for getting the
image from the external directory.
Private Sub PictureBox1_Click(sender As Object, e As EventArgs) Handles PictureBox1.Click
Try
With OpenFileDialog1
'CHECK THE SELECTED FILE IF IT EXIST OTHERWISE THE DIALOG BOX WILL DISPLAY A WARNING.
.CheckFileExists = True
'CHECK THE SELECTED PATH IF IT EXIST OTHERWISE THE DIALOG BOX WILL DISPLAY A WARNING.
.CheckPathExists = True
'GET AND SET THE DEFAULT EXTENSION
.DefaultExt = "jpg"
'RETURN THE FILE LINKED TO THE LNK FILE
.DereferenceLinks = True
'SET THE FILE NAME TO EMPTY
.FileName = ""
'FILTERING THE FILES
.Filter = "(*.jpg)|*.jpg|(*.png)|*.png|(*.jpg)|*.jpg|All files|*.*"
'SET THIS FOR ONE FILE SELECTION ONLY.
.Multiselect = False
'SET THIS TO PUT THE CURRENT FOLDER BACK TO WHERE IT HAS STARTED.
.RestoreDirectory = True
'SET THE TITLE OF THE DIALOG BOX.
.Title = "Select a file to open"
'ACCEPT ONLY THE VALID WIN32 FILE NAMES.
.ValidateNames = True
If .ShowDialog = DialogResult.OK Then
Try
PictureBox1.Image = Image.FromFile(OpenFileDialog1.FileName)
Catch fileException As Exception
Throw fileException
End Try
End If
End With
Catch ex As Exception
MsgBox(ex.Message, MsgBoxStyle.Exclamation, Me.Text)
End Try
End Sub
Step 7
Do the following code for
saving the picture when the button is clicked.
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
sql = "Insert into tblimage (img) Values (@img)"
saveImage(sql)
MsgBox("Image has been saved in the database")
End Sub
Output:
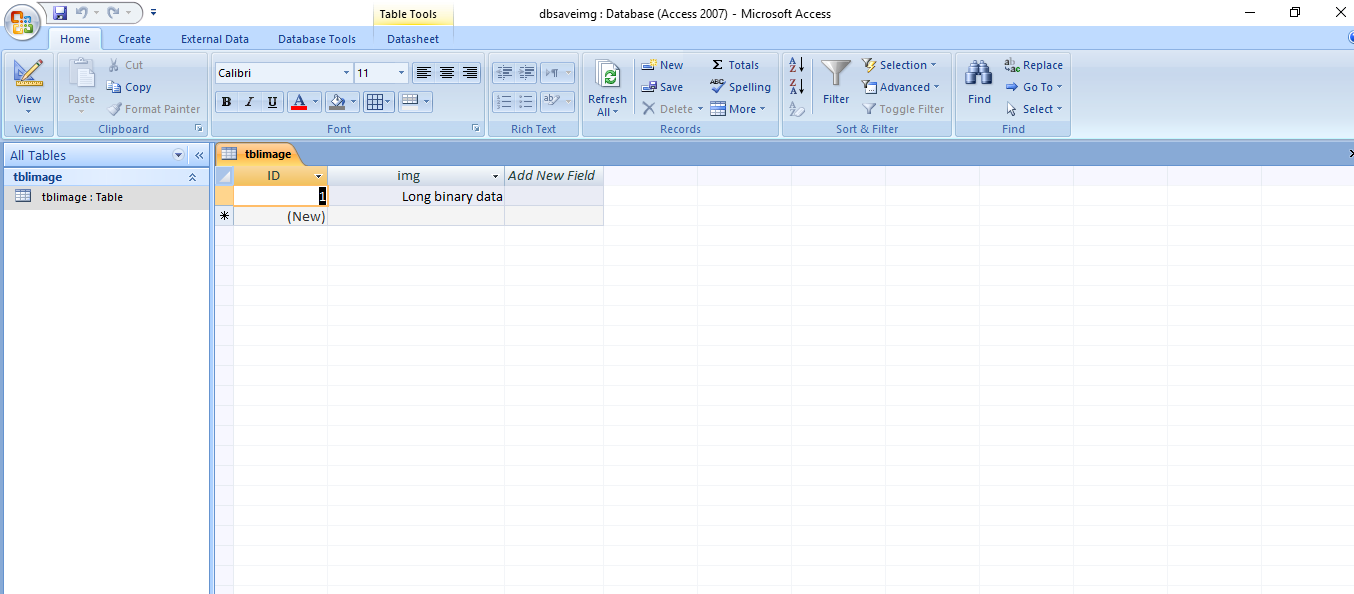
Download the complete source code and run it on your computer.
For any questions about this article. You can contact me @
Email –
[email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below