School Event Registration
Submitted by rinvizle on Saturday, November 19, 2016 - 09:18.
School Event Registration System will show you how to create this simple project. This system creates a registration form for all the user's to fill up the form and creates a record of each users details who had been registered. And it is useful for different kind of event to track every users that they are in the record or list of registered member. This project is compose of PHP, Bootsrap, Javascript, Modal and MySQL, this programs are written in a way that any one can understand and customize for their own and personal projects.
And for the UI Design of the Member Registration.
And For The Admin Side. We put all the forms in one location and then creating a link to each form so the code will be shorten and it will be easy to use and understand by the user.
Home.php - This form is compose of php script to make the code short and call every sessions by the syntax of php function.
Hope that you learn in this tutorial. And for more updates and programming tutorials don't hesitate to ask and we will answer your questions and suggestions. Don't forget to LIKE & SHARE this website.
Sample Code
Index.php - Script for registering a member from the system through the database.- <?php
- $fn=$_POST['fn'];
- $ln=$_POST['ln'];
- $age=$_POST['age'];
- $gender=$_POST['gender'];
- $address=$_POST['address'];
- $email=$_POST['email'];
- $c_number=$_POST['c_number'];
- ?>
- <script type="text/javascript">
- alert('You Are Successfully Registered Thank You');
- window.location="index.php";
- </script>
- <?php
- }
- ?>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <div class="navbar-header">
- <a class="navbar-brand" href="https://www.sourcecodester.com">Sourcecodester</a>
- </div>
- <ul class="nav navbar-nav">
- <li class="active"><a href="index.php">Home</a></li>
- <li><a href="#">About</a></li>
- <li><a href="#">Contact Us</a></li>
- </ul>
- </div>
- </nav>
- <div class="container">
- <h2 align="center">School Event Registration</h2><hr>
- <p align="center" style="color:#fff">Fill Up All The Details Below!</p>
- <form class="form-horizontal" method="POST">
- <div class="form-group">
- <label class="control-label col-sm-2" for="inputEmail">FirstName:</label>
- <div class="span4">
- <input type="text" name="fn" class="form-control" id="inputEmail" placeholder="FirstName" required>
- </div>
- </div>
- <div class="form-group">
- <label class="control-label col-sm-2" for="inputEmail">LastName:</label>
- <div class="span4">
- <input type="text" name="ln" class="form-control" id="inputEmail" placeholder="LastName" required>
- </div>
- </div>
- <div class="form-group">
- <label class="control-label col-sm-2" for="inputEmail">Age:</label>
- <div class="span4">
- <input type="text" name="age" class="form-control" id="inputEmail" placeholder="Age" required>
- </div>
- </div>
- <div class="form-group">
- <label class="control-label col-sm-2" for="inputEmail">Gender:</label>
- <div class="span4">
- <select class="span2" name="gender" required>
- <option></option>
- <option>Male</option>
- <option>Female</option>
- </select>
- </div>
- </div>
- <div class="form-group">
- <label class="control-label col-sm-2" for="inputEmail">Address:</label>
- <div class="span4">
- <input type="text" name="address" class="form-control" id="inputEmail" placeholder="Address" required>
- </div>
- </div>
- <div class="form-group">
- <label class="control-label col-sm-2" for="inputEmail">Email:</label>
- <div class="span4">
- <input type="text" name="email" class="form-control" id="inputEmail" placeholder="Email" required>
- </div>
- </div>
- <div class="form-group">
- <label class="control-label col-sm-2" for="inputEmail">Contact Number:</label>
- <div class="span4">
- <input type="text" name="c_number" class="form-control" id="inputEmail" placeholder="Contact Number" required>
- </div>
- </div>
- <div class="form-group">
- <div class="col-sm-offset-2 col-sm-10">
- <button type="submit" name="submit" class="btn btn-primary">Submit</button>
- </div>
- </div>
- </form>
- <?php
- include('header.php');
- include('session.php');
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>School Event Registration</title>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <div class="navbar-header">
- <a class="navbar-brand" href="https://www.sourcecodester.com">Sourcecodester</a>
- </div>
- <ul class="nav navbar-nav">
- <li class="active"><a href="index.php">Home</a></li>
- <li><a href="#">About</a></li>
- <li><a href="#">Contact Us</a></li>
- </ul>
- </div>
- </nav>
- <h1 align="center">School Event Registration</h1>
- <div class="container">
- <div class="row-fluid">
- <div class="span12">
- <ul id="myTab" class="nav nav-tabs">
- <li class="active"><a href="#member" data-toggle="tab"><i class="icon-group icon-large"></i> Registered Member</a></li>
- <li><a href="#user" data-toggle="tab"><i class="icon-user icon-large"></i> User</a></li>
- <li>
- <a data-toggle="modal" data-target="#myModal"><i class="icon-signout icon-large"></i> Logout</a>
- </li>
- </ul>
- </div>
- </div>
- <div class="tab-content">
- <?php
- include('tab_member.php');
- ?>
- <?php
- include('tab_user.php');
- ?>
- </div>
- <?php
- include('modal.php');
- ?>
- </div>
- </body>
- </html>
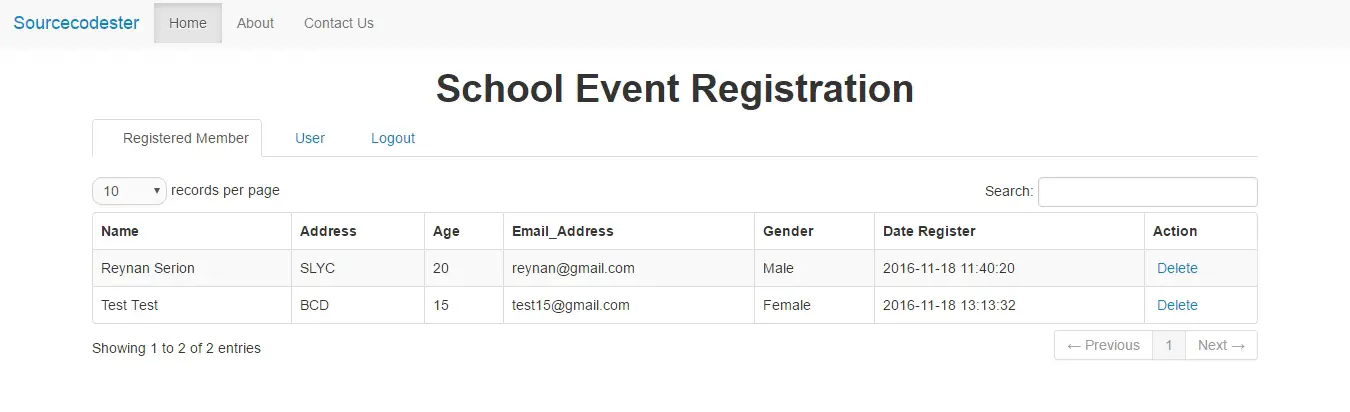
Comments
Add new comment
- Add new comment
- 618 views