TypeError: 'dict_keys' object is not subscriptable [Solved]
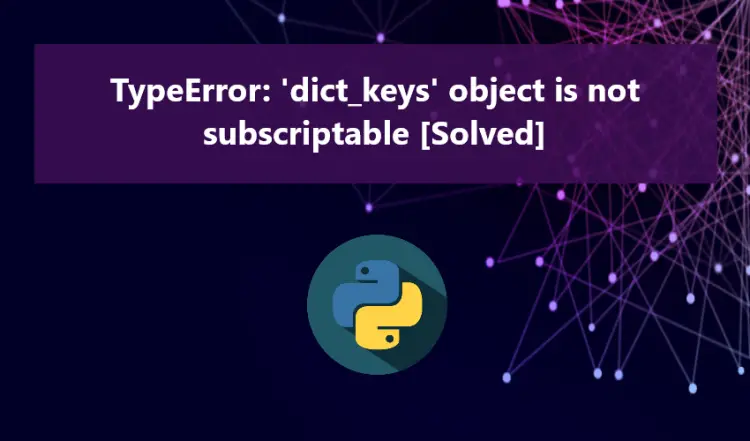
Within this article, we will delve into the causes of and solutions to avert the Python TypeError that manifests as "TypeError: 'dict_keys' object cannot be accessed with square brackets". If you are presently engaged in your Python project development and encounter this error unexpectedly, this piece will provide you with an understanding of why this issue arises and offer effective solutions for its resolution. I will also supply sample code snippets that reproduce the error and provide corresponding remedies to address it.
Python development can give rise to various error exceptions, one of which falls under the classification of Python TypeError. This error frequently surfaces when errors are made in writing Python scripts. Such errors stem from attempts to execute an operation or employ a function with an object of an incompatible data type. Aside the specific error that we will discuss here, TypeError can manifest in the following exceptions:
- TypeError: 'dict_values' object is not subscriptable
- TypeError: bad operand type for unary +: 'str'
- TypeError: cannot convert the series to class 'float'
To gain a deeper understanding of the listed exceptions, you can easily click on the messages above to be directed to their respective dedicated articles.
What Causes the Occurrence of Python's "TypeError: 'dict_keys' object is not subscriptable" Error?
The Python error "TypeError: 'dict_keys' object is not subscriptable" arises when you try to access the keys or index elements of a dict_keys object using square brackets ( `[]
` ), as if it were a list or dictionary object, which it is not.
Here's a sample Python snippet that demonstrate or reproduce the error to occur:
- # Sample Dictionary
- testDict = { 'a': 'SourceCodester', 'b': 'Free Source Codes', 'c': 'Python' }
- DictKeys = testDict.keys()
- # Output
- print(DictKeys[0])
The "TypeError: 'dict_keys' object is not subscriptable" occurs when attempting to retrieve the first key using `print(DictKeys[0])
`.
Resolving the "TypeError: 'dict_keys' object is not subscriptable" Python Error
Here are several potential solutions you can employ to address the error or exception that occurs when attempting to access a dict_keys object using square brackets ([]
):
Solution 1: Utilizing the list() Function
Python provides a built-in function called list(). This function is designed to generate a new list object and to transform other iterable objects into list objects. By applying this function, you can effortlessly convert the dict_keys into a list object, thereby enabling you to access the keys using square brackets ([]
).
Here's a sample snippet that demonstrate this solution:
- # Sample Dictionary
- testDict = { 'a': 'SourceCodester', 'b': 'Free Source Codes', 'c': 'Python' }
- DictKeys = list(testDict.keys())
- # Output
- print(DictKeys[0])
- print(DictKeys[1])
- print(DictKeys[2])
Solution 2: Employing List Comprehension
In Python, you can also utilize list comprehension to transform iterated keys or values into a list object by enclosing the square brackets around the iteration. To achieve this, you can employ a for loop to iterate through the keys contained within the dict_keys object. You can gain a clearer understanding of this solution by referring to the sample code snippet provided below:
- testDict = { 'a': 'SourceCodester', 'b': 'Free Source Codes', 'c': 'Python' }
- DictKeys = [key for key in testDict]
- # Output
- print(DictKeys[0])
- print(DictKeys[1])
- print(DictKeys[2])
Solution 3: Iterating through Keys Using a for loop
Alternatively, you can access the keys directly without converting the dict_keys object into a list. This solution involves using a for loop to dynamically set the key within square brackets for key access. Refer to the following code snippet that illustrates this approach:
- # Sample Dictionary
- testDict = { 'a': 'SourceCodester', 'b': 'Free Source Codes', 'c': 'Python' }
- for key in testDict:
- print(key)
Solution 4: Employing the sorted() Function
Python provides a built-in function called sorted(). This function is primarily intended for sorting iterable objects like lists, tuples, and strings, either into a new sorted list or as a sorted iterable. Because this function returns the keys as a list object, you can access the keys using square brackets. Refer to the following code snippet for a clearer grasp of this solution:
- # Sample Dictionary
- testDict = { 'a': 'SourceCodester', 'b': 'Free Source Codes', 'c': 'Python' }
- DictKeys = sorted(testDict.keys())
- # Output
- print(DictKeys[0])
- print(DictKeys[1])
- print(DictKeys[2])
Conclusion
In simple terms, the "TypeError dict_keys object is not subscriptable" Python error occurs when attempting to access keys using square brackets ([]
). You can resolve this error by employing one of the solutions provided above.
With this information, you now have the knowledge to address the error you may encounter during your Python project development. Explore this website for additional resources, including Free Source Codes, Tutorials, and Articles covering various programming languages.