AttributeError: 'list' object has no attribute 'items' [Solved]
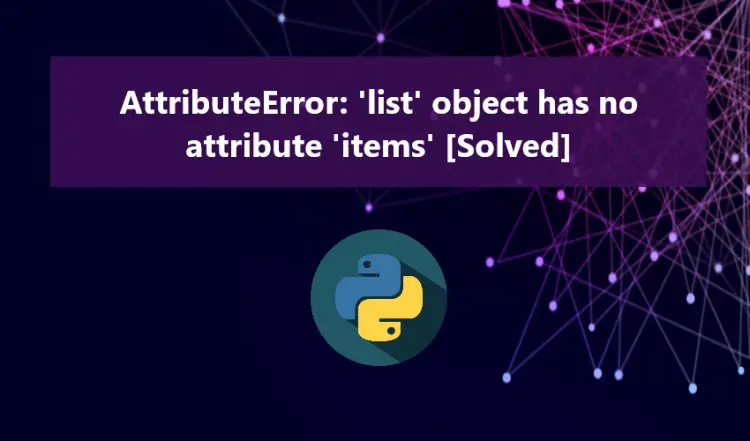
In this article, we will discuss the reasons behind the Python AttributeError error, specifically when it reads AttributeError: 'list' object has no attribute 'items'. If you are currently facing this error in your Python project's development phase, this article will provide insights into the causes of the error and how to resolve it.
In Python, there are numerous types of error exceptions that may occur during your project development, and some of these fall under the category of Python AttributeError. This error is an exception that arises when you attempt to access or manipulate an attribute of an object that doesn't exist or isn't applicable to that object's type. Here are the common reasons why AttributeError occurs:
- Accessing Non-Existent Attributes: Occurs when trying to access an attribute that is not defined for an object.
- Typographical Errors: Happens due to misspelling or typing the attribute name incorrectly.
- Using the Wrong Data Type: Arises when attempting to call or use a certain attribute or method that is not applicable to the data type of an object.
Some of the Python AttributeError exceptions that are often occurs such as the following:
- "AttributeError: module 'datetime' has no attribute 'now'"
- "AttributeError: 'list' object has no attribute 'lower'"
- "AttributeError: 'DataFrame' object has no attribute 'concat'"
Click on the error messages to be directed to a dedicated article for a deeper understanding of the issue.
What Causes the Python "AttributeError: 'list' object has no attribute 'items'" Error?
The Python "AttributeError: 'list' object has no attribute 'items'" error occurs when an attempt is made to use the items() method on a list data object. It's important to note that the items() method is a built-in function in Python intended for use with dictionaries. This method is designed to return a view object that displays a list of (key, value) tuple pairs from a dictionary, which is why this error is triggered.
To gain a deeper understanding, here's an example snippet that reproduces the error:
- # Sample List Object
- myList = [ "SourceCodester", "Free Source Code", "Python", "Programming" ]
- # Output list Items
- print(myList.items())
Solutions
Explore these potential fixes if you require the use of the items() method.
Converting a List Object into a Dictionary
You can easily transform a List object into a Dictionary by using the index of each item as the key and the item itself as the value for the 'dict' data structure. To accomplish this, you can employ the built-in Python function known as enumerate() to iterate through the list items while obtaining their indices to set as keys. Here's a sample code snippet that illustrates this solution:
- # Sample List Object
- myList = [ "SourceCodester", "Free Source Code", "Python", "Programming" ]
- # Convert List to Dict
- myDict = [(index, item) for index, item in enumerate(myList)]
- myDict = dict(myDict)
- # Output list Items
- print(myDict.items())
Assigning Keys to List Items
You can easily convert or set the list object to a dictionary object by simply adding keys to the item values and using the dict() function. For a practical example of this solution, please refer to the following code snippet:
- # Sample List Object
- myList = [ (0, "SourceCodester"), (1, "Free Source Code"), (2, "Python"), (3, "Programming") ]
- # Convert List to Dict
- myDict = dict(myList)
- # Output list Items
- print(myDict.items())
Implementing a Function for List-to-Dictionary Conversion
This solution resembles the first one you provided, with the added benefit of creating a reusable function. With this approach, you can convert a list object into a dictionary effortlessly by calling the function multiple times, eliminating the need to repeatedly write the entire conversion process. For a demonstration of this solution, refer to the code snippet below:
- def listToDict(listObj):
- newDict = {}
- for index, item in enumerate(listObj):
- newDict[index] = item
- return newDict
- # Sample List Object
- myList = [ "SourceCodester", "Free Source Code", "Python", "Programming" ]
- myDict = listToDict(myList)
- # Output list Items
- print(myDict.items())
Conclusion
To put it simple, the AttributeError: 'list' object has no attribute 'items' error occurs when attempting to use or call the items() method on a list data type. This issue can be easily resolved by converting the list object into a dictionary.
There you have it! I trust that this article will assist you in addressing the error you've encountered during your Python development journey. Don't hesitate to explore more Free Source Codes, Tutorials, and Articles covering a wide range of programming languages.
Happy Coding =)
- 13386 views