How to Add the Items in the ListBox Using InputBox in VB.Net
Submitted by janobe on Thursday, May 15, 2014 - 20:43.
In this tutorial I will teach you how to add the items in the ListBox in VB.Net. With this, you can type whatever data you want in the InputBox and it will be added in the ListBox. The InputBox has been just like a MessageBox, their only difference is that the InputBox has a TextBox that you can input the data.
Let’s begin:
Open the Visual Studio and create a new Project. Drag a Button and a ListBox. Then, do the Form just like this.
After that, double click the Button to fire the
Then, you have to create a constant variable to store a message on it and set it to its default message.
After that, you have to add the input data from the InputBox in the ListBox.
Lastly, do this following code for clearing the data in the ListBox of the “Clear” Button.
These are all the codes that we made.
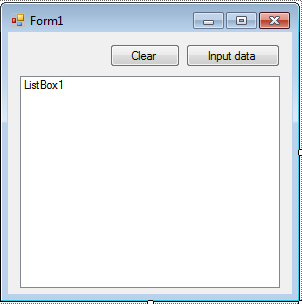
click
event handler.
Now, create a string variable to store the data that you have input and set the value on it.
- Dim inputdata As String = Nothing
- Const msg As String = "Input data"
- Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
- 'CLEARING THE DATA IN THE LISTBOX
- ListBox1.Items.Clear()
- End Sub
- Public Class Form1
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- 'SET A STRING VARIABLE FOR THE DATA
- 'TO BE INPUT AND FOR THE MESSAGE OF THE INPUTBOX
- Dim inputdata As String = Nothing
- Const msg As String = "Input data"
- 'ADDING THE DATA IN THE LISTBOX THROUGH INPUTBOX
- End Sub
- Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
- 'CLEARING THE DATA IN THE LISTBOX
- ListBox1.Items.Clear()
- End Sub
- End Class