Simple Query Builder using Visual Basic.Net
Submitted by joken on Tuesday, October 8, 2013 - 15:10.
In this tutorial, It covers how to create a simple MySQL query builder using Visual basic. This application allows user to select MySQL database and when the “use” button is clicked, the program will then load all the tables existing from a specific database. And every time the user select the name of a table it will generate a query and display the result on the datagridview provided. And it looks like as shown below.
To start building this application, add a following object on the form such as: one textbox, two buttons, three groupbox, listbox, label and a datagridview. Then design all the objects same as shown above.
This time we’re going to functionality to our application. First double click the form, and as you have observed you will be redirected into code view, and add the following declaration and one function for Mysql Connection below the public class. And here’s the code:
Next, on the form load. Add the following code:
This code will simply load all the databases on the combobox provided.
And it looks like as shown below.
Then, on the “use” button add the following code:
This code will display all the tables on a listbox based on the database selected by the user.
Next, we will add functionality to “Execute query” button. Add the following code:
Then on creating a query based on tables selected by the user. Here’s the following code:
And here’s all the codes for this tutorial:
And this time, you can test your program by pressing “F5”.
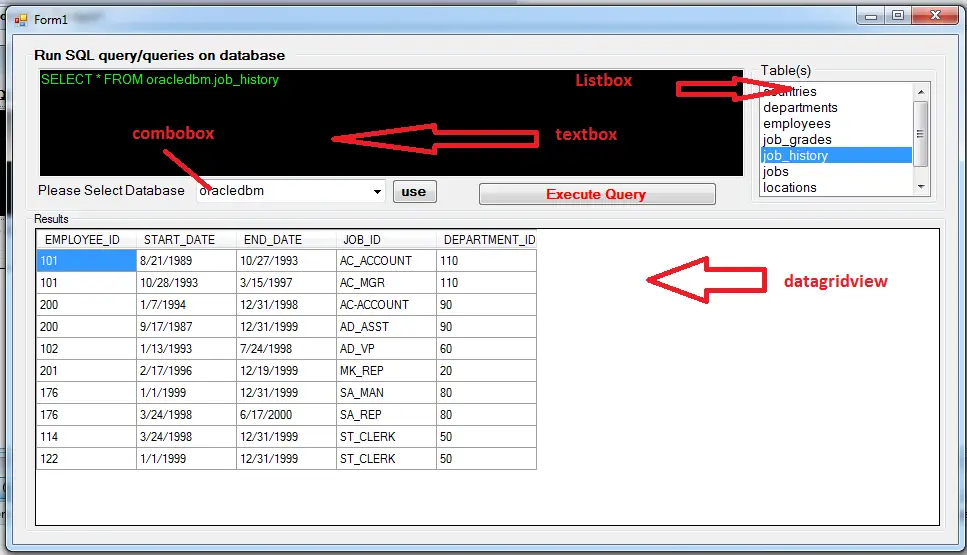
- 'Represents an SQL statement or stored procedure to execute against a data source.
- Dim cmd As New MySqlCommand
- Dim da As New MySqlDataAdapter
- Public total As Integer
- Dim publictable As New DataTable
- 'declare conn as connection and it will now a new connection because
- 'it is equal to Getconnection Function
- Dim con As MySqlConnection = jokenconn()
- Public Function jokenconn() As MySqlConnection
- Return New MySqlConnection("server=localhost;user id=root;password=;database=")
- End Function
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- Dim sql As String = "SHOW DATABASES"
- Dim publictable As New DataTable
- Try
- 'bind the connection and query
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- da.SelectCommand = cmd
- da.Fill(publictable)
- With cbdb
- .DataSource = publictable
- .DisplayMember = "Database"
- .ValueMember = "Database"
- End With
- da.Dispose()
- Catch ex As Exception
- End Try
- con.Clone()
- End Sub
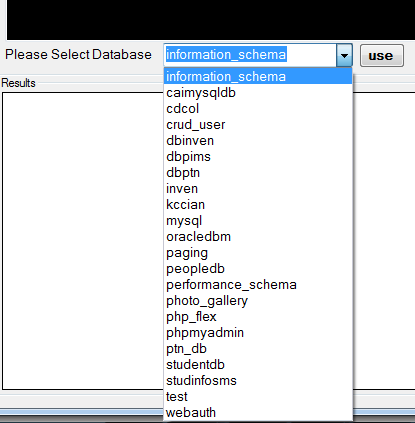
- Private Sub btntest_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btntest.Click
- Dim sql As String = "SHOW TABLES FROM " & cbdb.Text
- Dim publictable As New DataTable
- Try
- 'bind the connection and query
- With cmd
- .Connection = con
- .CommandText = Sql
- End With
- da.SelectCommand = cmd
- da.Fill(publictable)
- ' publictable.Rows.Add("Please Select...")
- With lstfield
- .DataSource = publictable
- .DisplayMember = "Tables_in_" & cbdb.Text
- .ValueMember = "Tables_in_" & cbdb.Text
- End With
- ' dtgrd.Columns(1).Visible = False
- da.Dispose()
- Catch ex As Exception
- End Try
- con.Clone()
- End Sub
- Private Sub btngo_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btngo.Click
- Dim publictable As New DataTable
- Dim sql As String
- sql = txtquery.Text
- Try
- 'bind the connection and query
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- 'Gets or sets a Transact-SQL statement or stored procedure used to select records in the data source.
- da.SelectCommand = cmd
- da.Fill(publictable)
- 'get the datasource from publictable and passed to datagridview
- dtresultview.DataSource = publictable
- 'dispose the dataadapter
- da.Dispose()
- Catch ex As Exception
- End Try
- da.Dispose()
- End Sub
- Private Sub lstfield_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles lstfield.Click
- txtquery.Text = "SELECT * FROM " & cbdb.Text & "." & lstfield.SelectedValue.ToString
- Call btngo_Click(sender, e)
- End Sub
- Imports MySql.Data.MySqlClient
- Public Class Form1
- 'Represents an SQL statement or stored procedure to execute against a data source.
- Dim cmd As New MySqlCommand
- Dim da As New MySqlDataAdapter
- Public total As Integer
- Dim publictable As New DataTable
- 'declare conn as connection and it will now a new connection because
- 'it is equal to Getconnection Function
- Dim con As MySqlConnection = jokenconn()
- Public Function jokenconn() As MySqlConnection
- Return New MySqlConnection("server=localhost;user id=root;password=;database=")
- End Function
- Private Sub btngo_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btngo.Click
- Dim publictable As New DataTable
- Dim sql As String
- sql = txtquery.Text
- Try
- 'bind the connection and query
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- 'Gets or sets a Transact-SQL statement or stored procedure used to select records in the data source.
- da.SelectCommand = cmd
- da.Fill(publictable)
- 'get the datasource from publictable and passed to datagridview
- dtresultview.DataSource = publictable
- 'dispose the dataadapter
- da.Dispose()
- Catch ex As Exception
- End Try
- da.Dispose()
- End Sub
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- Dim sql As String = "SHOW DATABASES"
- Dim publictable As New DataTable
- Try
- 'bind the connection and query
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- da.SelectCommand = cmd
- da.Fill(publictable)
- With cbdb
- .DataSource = publictable
- .DisplayMember = "Database"
- .ValueMember = "Database"
- End With
- da.Dispose()
- Catch ex As Exception
- End Try
- con.Clone()
- End Sub
- Private Sub btntest_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btntest.Click
- Dim sql As String = "SHOW TABLES FROM " & cbdb.Text
- Dim publictable As New DataTable
- Try
- 'bind the connection and query
- With cmd
- .Connection = con
- .CommandText = Sql
- End With
- da.SelectCommand = cmd
- da.Fill(publictable)
- ' publictable.Rows.Add("Please Select...")
- With lstfield
- .DataSource = publictable
- .DisplayMember = "Tables_in_" & cbdb.Text
- .ValueMember = "Tables_in_" & cbdb.Text
- End With
- ' dtgrd.Columns(1).Visible = False
- da.Dispose()
- Catch ex As Exception
- End Try
- con.Clone()
- End Sub
- Private Sub lstfield_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles lstfield.Click
- txtquery.Text = "SELECT * FROM " & cbdb.Text & "." & lstfield.SelectedValue.ToString
- Call btngo_Click(sender, e)
- End Sub
- End Class