Retrieving Data in MySQL Database Using DataReader in VB.Net
Submitted by janobe on Friday, August 17, 2018 - 07:28.
Today you will learn on how to retrieve data in MySQL Database using DataReader in VB.net. This method provides an effective way to retrieve data from the database. It is efficient to use this kind of method because you can design the datagridview column according to your desire.
This time, go to the code view and do the following codes above the Public Class for your imports.
After that, do the following codes below the Public Class to declare all the classes that are needed. Then, initialize your MySQL Connection
Go back to the Design View and double click the button in the form to fire the
Press f5 to run your project
If you find an error, please download mysql-connector-net-6.4.4.msi and install it on your machine. Add the
Let’s get started:
Create a database and name it, “peopledb” After creating database, add a table in it.
After adding a table, insert a data in the table that you have created
Open Microsoft Visual Studio 2015 and create a new windows form application. After that, do the Windows Form just like shown below.
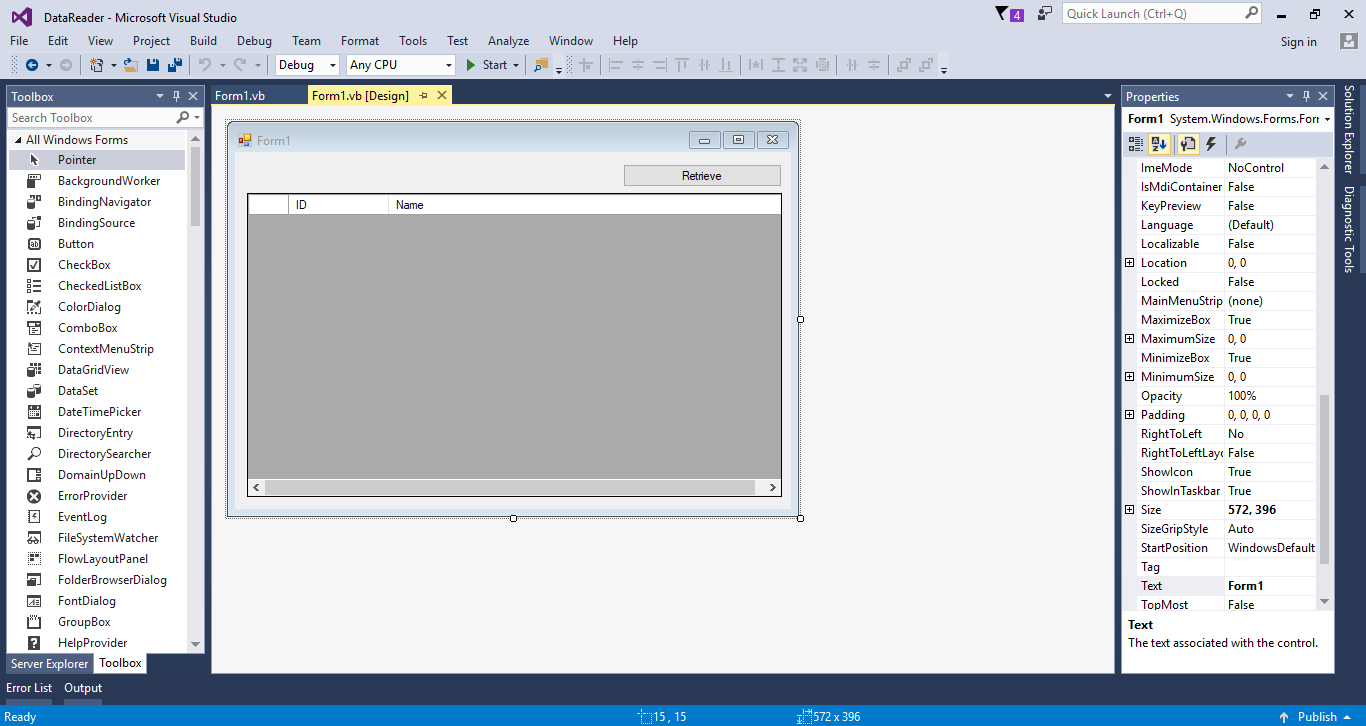
- Imports MySql.Data.MySqlClient
- Dim con As New MySqlConnection("server=localhost;user id=root;Password=janobe;database=peopledb")
- Dim cmd As MySqlCommand
- Dim dr As MySqlDataReader
- Dim sql As String
click event handler
of it.
Do the following code for retrieving data in the MySQL database.
- Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
- Try
- con.Open()
- sql = "SELECT * FROM `tbluser`"
- cmd = New MySqlCommand
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- dr = cmd.ExecuteReader()
- DataGridView1.Rows.Clear()
- Do While dr.Read = True
- DataGridView1.Rows.Add(dr(0), dr(1))
- Loop
- Catch ex As Exception
- MsgBox(ex.Message)
- Finally
- con.Close()
- End Try
- End Sub
MySQL.Data.dll
as a reference for the project that you have created.
You can download the complete source code and run it on your computer
For more question about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
FB Account – https://www.facebook.com/onnaj.soicalap