Python - Django Templating and Includes
Submitted by nurhodelta_17 on Friday, October 20, 2017 - 13:46.
Getting Started
First, we gonna create our website using the Django Framework,. if you haven't done so, you may refer to my tutorial entitled Python Getting Started w/ Django Framework.Creating and Installing our App
Next is we're gonna create and install a new app named aboutme. If you have no idea on how to create new app, please refer to my tutorial about Python - Django Creating New App.Setting up our URLs
1. In your site directory, open urls.py and include our app in the urlpatterns by typing: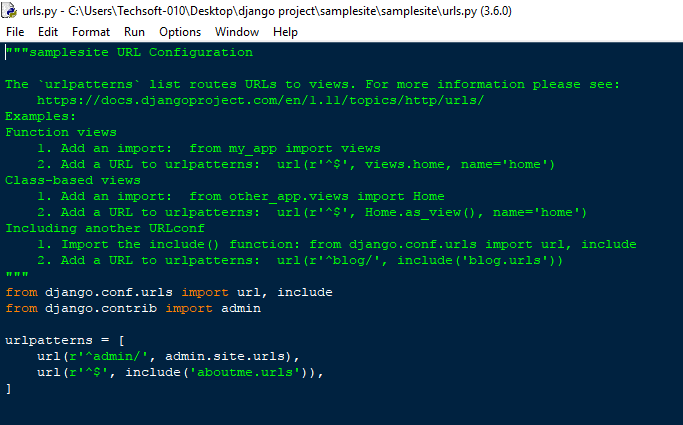
- from django.shortcuts import render
- def index(request):
- return render (request, 'aboutme/home.html')
- from django.conf.urls import url, include
- from . import views
- urlpatterns = [
- url(r'^$', views.index, name='index'),
- ]
Creating our Templates Directory
1. We're creating a directory for our templates by creating a new folder in our aboutme app. This will organise all our templates into one directory. 2. Inside our templates folder, we're gonna create another folder named aboutme. Basically, django process all templates in a folder regardless of any apps and to avoid conflicts in the names of the templates, we create a new folder to group our templates.Creating our HTML files
In the aboutme folder inside our templates folder, we create our html files. header.html
The {%%} is what we call the jinja logic
home.html
footer.html
So basically, we have set up our views.py to direct to home.html. In our home.html, we have our header.html template which we have extends using {% extends "" %} and we have our include which we have included using {% include "" %}.
Running the Server
Run your server and it should look like this: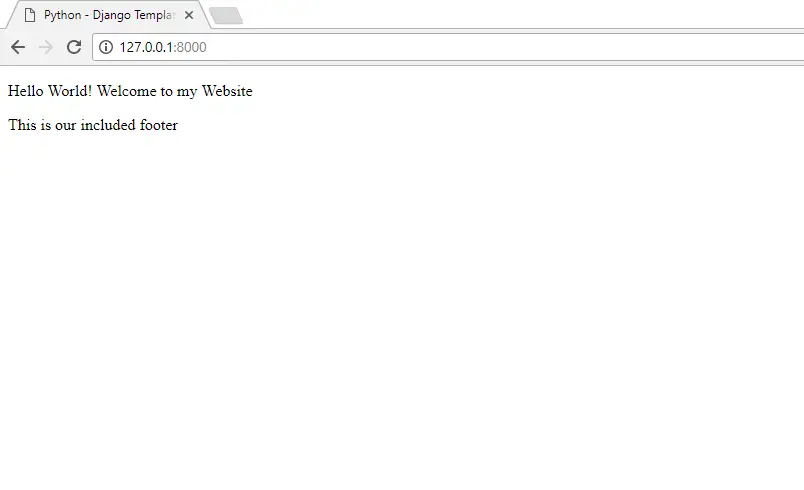
Add new comment
- 74 views