Python - Django Simple Search Filter
Submitted by razormist on Tuesday, October 17, 2017 - 21:16.
In this tutorial we will create a Simple Search Filter in Django. Django was created to achieve key objective and to ease the development of complicated, database-driven websites. It is a high-level Python Web framework with efficient programming capability . So let's now do the coding...
Note: Type '127.0.0.1:8000' in the url browser to view the server. When there is code changes in the server just (ctrl + C) to command prompt to stop the server from running, then start again to avoid errors.
It will be look like this:
Then after that create a view that will catch the redirect url. To do that create a file "views.py" inside the blog directory then copy/paste the code below.
Save it as "base.html" inside the blog directory "sub directory of templates".
index.html
Save it as "index.html" inside the blog directory "sub directory of templates".
There you have it we successfully created a Simple Search Filter in Django. I hope that this simple tutorial help you for what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!
Getting Started
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/. After Python IDLE's is installed, open the command prompt then type "pip install Django", and hit enter.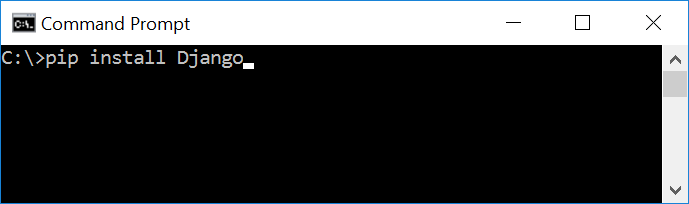
Creating the App
After django is set we will now create the web app. While your in the command prompt cd to the directory you want to save the app, then type "django-admin startproject server" and hit enter. A new folder will be created on the directory named 'server'.
Running The Server
After creating the project, cd to the newly created directory, then type "manage.py runserver" and hit enter to start the server running. The "manage.py" is a command of django-admin that utilize the administrative tasks of python web framework. Here is the image of python web server: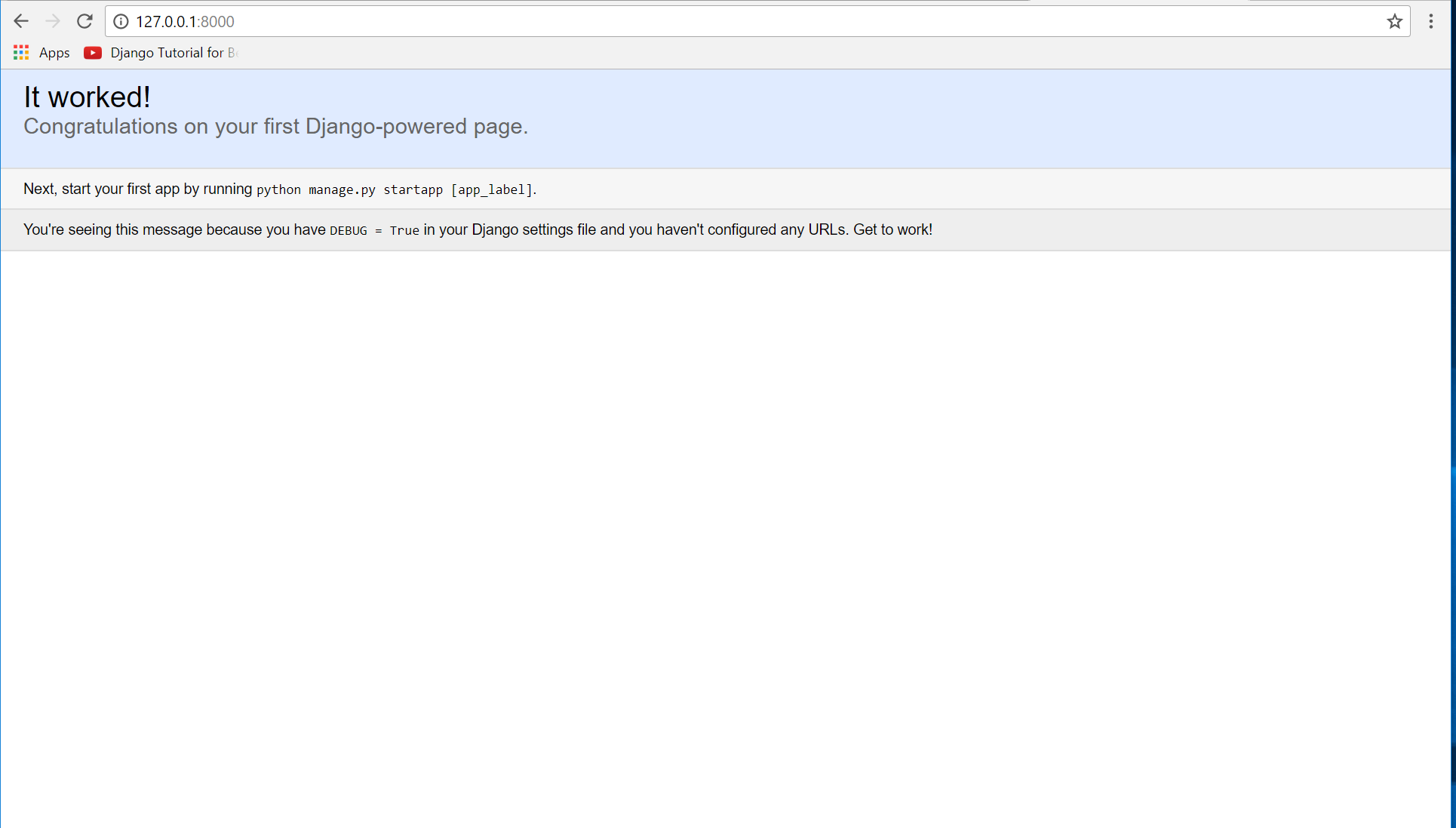
Creating The Website
This time will now create the web app to display the web models. First locate the directory of the app via command prompt cd, then type "manage.py startapp blog" and hit enter. A new directory will be created named "blog".
Setting up The URL
This time will now create a url address to connect the app to the server. First go to server directory, then open urls.py via Python IDLE's or any text editor. Then import "include" module beside the url module and import additional module to make a redirect url to your site "from . import views". After that copy/paste the code below inside the urlpatterns.- url(r'^$', views.index_redirect, name='index_redirect'),
- url(r'^blog/', include('blog.urls')),
- from django.conf.urls import include, url
- from django.contrib import admin
- from . import views
- urlpatterns = [
- url(r'^$', views.index_redirect, name='index_redirect'),
- url(r'^blog/', include('blog.urls')),
- url(r'^admin/', admin.site.urls),
- ]
- from django.shortcuts import redirect
- def index_redirect(request):
- return redirect('/blog/')
Creating The Path For The Pages
Now that we are all set we will now create a path for the web pages. All you have to do first is to go to blog directory, then copy/paste the code below and save it inside "blog" directory named it as 'urls.py' The file name must be urls.py or else there will be an error in the code.- from django.conf.urls import url
- from . import views
- urlpatterns = [
- url(r'^$', views.index, name='index'),
- url(r'^create$', views.create, name='create'),
- url(r'^search$', views.search, name='search'),
- ]
Creating A Static Folder
This time we will create a directory that store an external file. First go to the blog directory then create a directory called "static", after that create a sub directory called "blog". You'll notice that it is the same as your main app directory name to assure the absolute link. This is where you import the css, js, etc directory. For the bootstrap framework you get it from here http://getbootstrap.com/Creating The Views
The views contains the interface of the website. This is where you assign the html code for rendering it to django framework and contains a methods that call a specific functions. To do that open the views.py, the copy/paste the code below.- from django.shortcuts import render, redirect
- from .models import Member
- from django.db.models import Q
- # Create your views here.
- def index(request):
- members = Member.objects.all().order_by('lastname')
- return render(request, 'blog/index.html', {'members': members})
- def create(request):
- member = Member(firstname=request.POST['firstname'], lastname=request.POST['lastname'])
- member.save()
- return redirect('/')
- def search(request):
- members = Member.objects.filter(Q(firstname=request.GET.get('search')) | Q(lastname=request.GET.get('search')))
- return render(request, 'blog/index.html', {'members': members})
Creating The Models
Now that we're done with the views we will then create a models. Models is module that will store the database information to django. To do that locate and go to blog directory, then open the "models.py" after that copy/paste the code.- from django.db import models
- # Create your models here.
- class Member(models.Model):
- firstname = models.CharField(max_length=50)
- lastname = models.CharField(max_length=50)
- def __str__(self):
- return self.firstname + " " + self.lastname
Registering The App To The Server
Now that we created the interface we will now then register the app to the server. To do that go to the server directory, then open "settings.py" via Python IDLE's or any text editor. Then copy/paste this script inside the INSTALLED_APP variables 'blog'. It will be like this:- INSTALLED_APPS = [
- 'blog',
- 'django.contrib.admin',
- 'django.contrib.auth',
- 'django.contrib.contenttypes',
- 'django.contrib.sessions',
- 'django.contrib.messages',
- 'django.contrib.staticfiles',
- ]
Creating The Mark up Language
Now we will create the html interface for the django framework. First go to blog directory, then create a directory called "templates" and create a sub directory on it called blog. base.html- <!DOCTYPE html>
- <html lang="en">
- <head>
- {% load static %}
- <link rel="stylesheet" type="text/css" href="{% static 'blog/css/bootstrap.css' %}"/>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #000;"/>
- {% block body %}
- {% endblock %}
- </div>
- </body>
- </html>
- {% extends 'blog/base.html' %}
- {% block body %}
- <form method="POST" action="create">
- {% csrf_token %}
- <div class="form-inline">
- <input type="text" name="firstname" size="12" class="form-control" required="required"/>
- <input type="text" name="lastname" size="12" class="form-control" required="required"/>
- </div>
- </form>
- <form method="GET" action="search">
- <div class="form-inline">
- <input type="text" placeholder="Search" name="search" class="form-control" required="required" />
- </div>
- </form>
- <br />
- <table class="table table-bordered">
- <thead class="alert-danger">
- <tr>
- </tr>
- </thead>
- <tbody>
- {% for member in members%}
- <tr>
- </tr>
- {% endfor %}
- </tbody>
- </table>
- {% endblock %}
Migrating The App To The Server
Now that we're done in setting up all the necessary needed, we will now then make a migration and migrate the app to the server. To do that open the command prompt then cd to the "server" directory, then type "manage.py makemigrations" and hit enter. After that type again "manage.py migrate" then hit enter.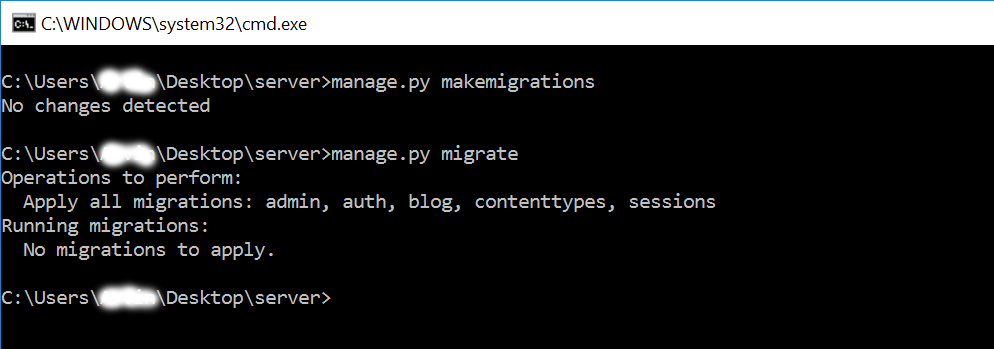