Creating a Registration Page Part I
Submitted by GeePee on Sunday, April 19, 2015 - 23:21.
In this tutorial, I will show you how create our Registration Page using Bootstrap framework. The Registration form together with the Login form will welcome you when you access the site. And it will require the new member to register on the site, so that they can login and to have access to the site.
First, create a PHP file named “index.php”, save it inside “philsocial” folder. And this PHP file is composed of different parts. For the first part, add the following code:
This part of the code, will handle the declaration of your HTML document before the
And here’s the different classes used in this tutorial:
If you want to see more of my works, new Source Code or Application and Tutorials Just click here.
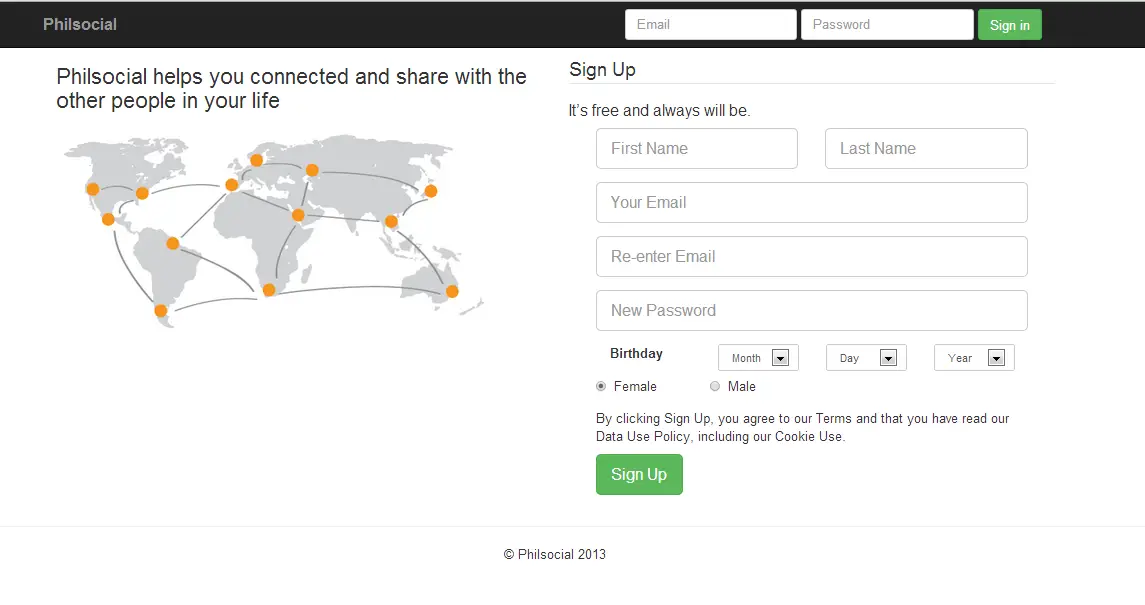
- class="navbar navbar-inverse navbar-fixed-top"
- class="container"
- class="navbar-header"
- class="navbar-toggle"
- class="navbar-collapse collapse"
- class="navbar-form navbar-right"
- class="form-group"
- class="form-control"
- class="rows"
- class="col-xs-6"
- class="form-horizontal"
- class="form-control input-lg"
- class="form-inline"
- class="form-control input-sm"
- class="radio"