Authentication Secure Login, Registration and View Profile
Submitted by rinvizle on Wednesday, November 16, 2016 - 14:32.
Authentication Secure Login, Registration and View Profile where the users of your websites can be able to create their accounts, and log in with their valid information so as to access the system to their various accounts. Users information during registration process have been validated and stored in the database. The system can show you the details about your profile that you've been created before and after you logging in. The system validates new users for duplicate email addresses and performs valid authentication during registered users log in. And this is compose of PHP and MySql, this programs are written in a way that any one can understand and customize.
Reg.php - For the registration of users here is the sample code.
Profile.php -and for the viewing of each users profile from the database to display in the webpage.
Hope that you learn in this tutorial. And for more updates and programming tutorials don't hesitate to ask and we will answer your suggestions. Don't forget to LIKE & SHARE this website.
Sample Code
Index.php - And for the login page here is the scripted code to be use.- <?php
- $email=$_POST['email'];
- $pass=$_POST['pass'];
- if($email==''){
- echo "<script>alert('Email Id Incorrect, try again..')</script>";
- }
- if($pass==''){
- echo "<script>alert('Password Incorrect, try again.. !!')</script>";
- }
- else
- {
- $query="select * from user_reg where email='$email' AND pass='$pass'";
- echo "<script>alert('Your Logged in Successfully !!')</script>";
- echo "<script>window.open('home.php','_self')</script>";
- }
- }
- }
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>Authentication Secure Login, Registration and View Profile</title>
- <link rel="stylesheet" href="css/bootstrap.min.css">
- <script src="js/jquery.min.js"></script>
- <script src="js/bootstrap.min.js"></script>
- </head>
- <body>
- <h2 align="center"><em>Authentication Secure Login, Registration and View Profile</em></h2>
- <div class="container">
- <form action="" method="post">
- <h2 align="center"><em>Sign In Here !!</em></h2>
- <div class="form-group">
- <label for="email">Email address</label>
- <input type="email" name="email" class="form-control" id="email" required>
- </div>
- <div class="form-group">
- <label for="pwd">Password</label>
- <input type="password" name="pass" class="form-control" id="pwd" required>
- </div>
- <div class="checkbox">
- <label><input type="checkbox"> Remember me</label>
- <label><a href="reg.php" style="color:#fff"> <b>Sign Up</label>
- </div>
- <button type="submit" name="submit" class="btn btn-default">Submit</button>
- </form>
- </div>
- </body>
- </html>
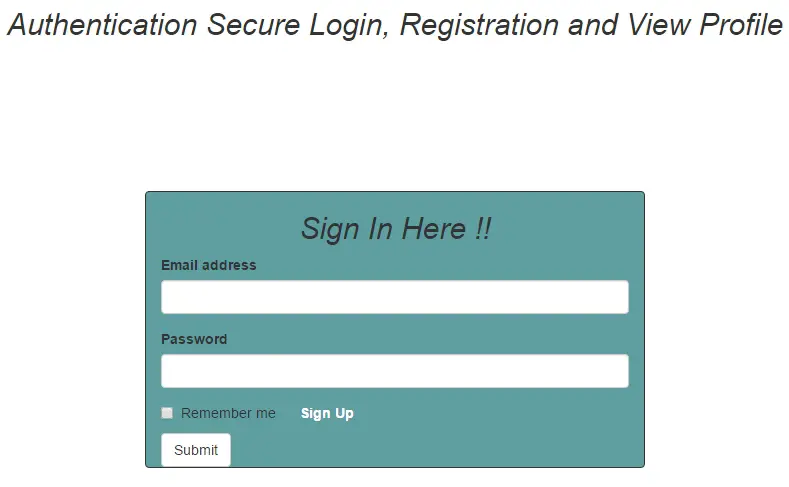
- <?php
- {
- $fname=$_POST['fname'];
- $lname=$_POST['lname'];
- $pass=$_POST['pass'];
- $cpass=$_POST['cpass'];
- $email=$_POST['email'];
- $mo=$_POST['mobile'];
- $city=$_POST['city'];
- if($fname=='')
- {
- echo "<script>alert('Please Enter First Name')</script>";
- }
- if($lname=='')
- {
- echo "<script>alert('Please Enter Last Name')</script>";
- }
- if($pass=='')
- {
- echo "<script>alert('Please Enter a Password')</script>";
- }
- if($cpass=='')
- {
- echo "<script>alert('Password is do not Match,Please try again')</script>";
- }
- if($email=='')
- {
- echo "<script>alert('Please Enter Email')</script>";
- }
- if($mo=='')
- {
- echo "<script>alert('Please Enter Mobile No')</script>";
- }
- if($city=='')
- {
- echo "<script>alert('Please Enter City')</script>";
- }
- else
- {
- $query="insert into user_reg(fname,lname,pass,cpass,email,mobile,city) values ('$fname','$lname','$pass','$cpass','$email','$mo','$city')";
- echo "<script>alert('Signing Successfully !!')</script>";
- echo "<script>window.open('index.php','_self')</script>";
- }
- }
- }
- ?>
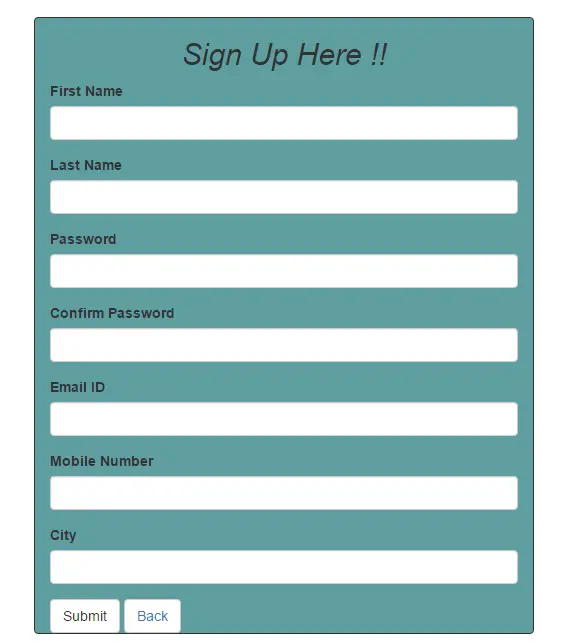
- <div align="center" class="container">
- <h3>My Profile</h3>
- <?php
- include "connect.php";
- $q="select * from user_reg order by id asc";
- $fname=$rs['fname'];
- $lname=$rs['lname'];
- $email=$rs['email'];
- $mobile=$rs['mobile'];
- $city=$rs['city'];
- echo "
- <table class='table table-condensed'>
- <thead>
- <tr>
- <th>Firstname</th>
- <th>Lastname</th>
- <th>Email</th>
- <th>Mobile</th>
- <th>City</th>
- </tr>
- </thead>
- <tbody>
- <tr>
- <td>".$fname."</td>
- <td>".$lname."</td>
- <td>".$email."</td>
- <td>".$mobile."</td>
- <td>".$city."</td>
- </tr>
- </tbody>
- </table>";
- }
- ?>
- </div>

Add new comment
- 619 views