How To Create User Login Page In PHP/MySQL Using PDO Query
Submitted by alpha_luna on Friday, May 6, 2016 - 13:44.
On my previous tutorial, I make an article and it's called Registration Form In PHP/MySQL Using PDO Query. If you are looking for on How To Create User Login Page In PHP/MySQL Using PDO Query then you are at the right place. And this is the follow-up tutorial for Registration Form In PHP/MySQL Using PDO Query. Let's start with:
List of Users:
We are going to make our Log In Page.
We are going to make our database connection.
We are going to make our login script.
And, this code for the logout query then saves it as "log_out_query.php".
And, this source code for the home page kindly copy and save it as "home.php".
And, this is the style.
If the user types incorrect details in the login page.
And, this is our Home Page.
You can use this source code to merge the previous tutorial that I made and it's called Registration Form In PHP/MySQL Using PDO Query.
This is all the steps on How To Create User Login Page In PHP/MySQL Using PDO Query. So, this is it, or you can download the full source code below by clicking the "Download Code" button below.
Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at [email protected]. Practice Coding. Thank you very much.
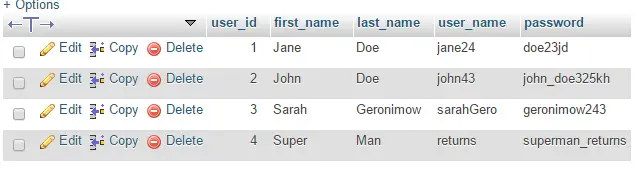
Creating our Table
We are going to make our database. To create a database:- Open the PHPMyAdmin.
- Create a database and name it as "registration_pdo".
- After creating a database name, then we are going to create our table. And name it as "user_registration".
- --
- -- Table structure for table `user_registration`
- --
- CREATE TABLE `user_registration` (
- `user_id` INT(11) NOT NULL,
- `first_name` VARCHAR(100) NOT NULL,
- `last_name` VARCHAR(100) NOT NULL,
- `user_name` VARCHAR(100) NOT NULL,
- `password` VARCHAR(100) NOT NULL
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Creating Log In Page
This source code that the user types their username and password to log in.Database Connection
This PHP Script is our database. Copy and paste this then save it as "connection.php".- <?php $conn = new PDO('mysql:host=localhost; dbname=registration_pdo','root', ''); ?>
Log In Query
This step is to create our login script and save it as "log_in_query.php".- <?php
- include('connection.php');
- $user_name = $_POST['user_name'];
- $password = $_POST['password'];
- $query = $conn->query("Select * from user_registration where user_name = '$user_name' and password ='$password' ");
- $count = $query->rowcount();
- $row = $query->fetch();
- if ($count > 0){
- $_SESSION['id'] = $row['user_id'];
- } else {
- ?>
- <script>
- alert("Incorrect Details. Check your User Name or Password.")
- window.location="index.php";
- </script>
- <?php
- }
- ?>
- <?php
- ?>
- <?php include ('connection.php'); ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>How To Create User Login Page In PHP/MySQL Using PDO Query</title>
- <link rel="stylesheet" type="text/css" href="styles.css" />
- </head>
- <body>
- <?php
- }
- $session_id = $_SESSION['id'];
- $session_query = $conn->query("select * from user_registration where user_id = '$session_id'");
- $user_row = $session_query->fetch();
- ?>
- <table border="0" cellpadding="10" cellspacing="1" width="100%" class="tblLogin">
- <tr class="tableheader">
- <td align="center">Home</td>
- </tr>
- <tr class="tablerow">
- <td align="center">
- <p class="blink_text">Welcome User!!!</p>
- <?php echo $user_row['first_name']." ".$user_row['last_name']; ?>
- </td>
- </tr>
- <tr class="tableheader">
- <td align="center">
- Click here to <a href="log_out_query.php"> Logout </a>
- </td>
- </tr>
- </table>
- </body>
- </html>
- body {
- width:500px;
- margin:auto;
- }
- h2 {
- color:red;
- font-family:cursive;
- text-align:center;
- }
- a {
- text-decoration:none;
- color:black;
- }
- a:hover {
- color:red;
- }
- .tableheader {
- background-color: aliceblue;
- color:blue;
- font-weight:bold;
- font-size:large;
- }
- .tablerow {
- background-color: whitesmoke;
- color:blue;
- font-weight:bold;
- font-size:18px;
- }
- #message {
- color: white;
- border: red 1px solid;
- background: red;
- padding:20px;
- font-size:18px;
- font-weight:bold;
- margin-top: 31px;
- border-radius:4px;
- width: 305px;
- }
- .tblLogin{
- margin : auto;
- border:blue 3px solid;
- }
- .textbox_detail {
- font-size:18px;
- text-indent:5px;
- background:azure;
- border:blue 1px solid;
- border-radius:4px;
- cursor:pointer;
- }
- .btn_submit {
- font-size:18px;
- width:100px;
- border:blue 1px solid;
- background:azure;
- color:blue;
- cursor:pointer;
- }
- .blink_text {
- -webkit-animation-name: blinker;
- -webkit-animation-duration: 1s;
- -webkit-animation-timing-function: linear;
- -webkit-animation-iteration-count: infinite;
- -moz-animation-name: blinker;
- -moz-animation-duration: 1s;
- -moz-animation-timing-function: linear;
- -moz-animation-iteration-count: infinite;
- animation-name: blinker;
- animation-duration: 1s;
- animation-timing-function: linear;
- animation-iteration-count: infinite;
- color:red;
- font-size:large;
- }
- @-moz-keyframes blinker {
- 0% { opacity: 1.0; }
- 50% { opacity: 0.0; }
- 100% { opacity: 1.0; }
- }
- @-webkit-keyframes blinker {
- 0% { opacity: 1.0; }
- 50% { opacity: 0.0; }
- 100% { opacity: 1.0; }
- }
- @keyframes blinker {
- 0% { opacity: 1.0; }
- 50% { opacity: 0.0; }
- 100% { opacity: 1.0; }
- }
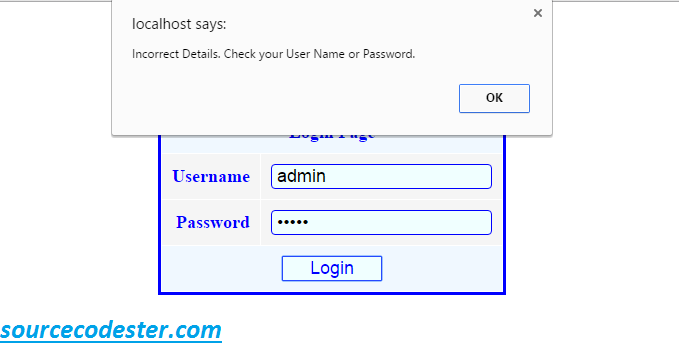
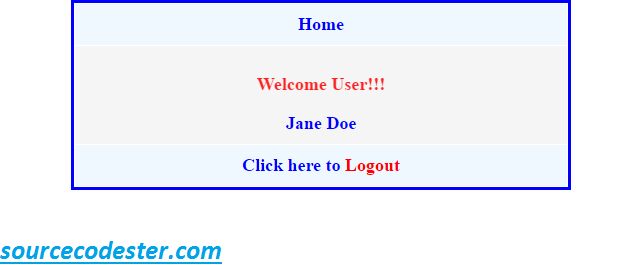